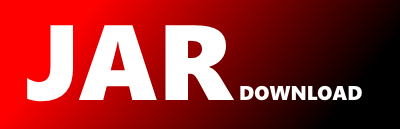
eleme.openapi.sdk.media.trace.ReportClient Maven / Gradle / Ivy
package eleme.openapi.sdk.media.trace;
import eleme.openapi.sdk.media.MediaConfiguration;
import eleme.openapi.sdk.media.Result;
import eleme.openapi.sdk.media.common.AuthUtil;
import eleme.openapi.sdk.media.common.http.HttpClientBase;
import eleme.openapi.sdk.media.utils.CompressUtils;
import eleme.openapi.sdk.media.utils.IOUtils;
import org.apache.http.client.entity.EntityBuilder;
import org.apache.http.client.methods.HttpPost;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.List;
/**
* Created by huamulou on 15/12/10.
*/
public class ReportClient extends HttpClientBase {
private final static String TRACE_URI = "/3.0/trace";
private static byte[] LINE_BREAK;
static {
try {
LINE_BREAK = "\n".getBytes("utf-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
public void report(List reports) {
MediaConfiguration configuration = MediaConfiguration.getLastConfiguration();
if (configuration == null || configuration.getAk() == null || configuration.getSk() == null) {
throw new RuntimeException("no configuration");
}
HttpPost post = new HttpPost(MediaConfiguration.DATA_ENDPOINT + TRACE_URI);
byte[] rawBody = getRawBodyOfString(reports);
byte[] body = rawBody;
if (rawBody == null) return;
boolean isGzip = false;
if (rawBody.length > 1024) { // compress if longer than 1024
isGzip = true;
body = CompressUtils.compress(rawBody);
body = body == null ? rawBody : body;
}
post.setHeader("Content-Type", "application/octet-stream");
if (isGzip)
post.setHeader("Content-Encoding", "gzip");
post.setEntity(EntityBuilder.create().setBinary(body).build());
try {
AuthUtil.authRequest(post, configuration.getAk(), configuration.getSk(), false);
} catch (IOException e) {
throw new RuntimeException(e.getMessage());
}
Result result = execute(post, Void.class);
if (!result.isSuccess()) {
throw new RuntimeException(result.getHttpStatus() + ":" + result.getCode() + ":" + result.getMessage() + ":" + result.getRequestId(),
result.getT());
}
}
private byte[] getRawBodyOfString(List reports) {
ByteArrayOutputStream out = null;
try {
out = new ByteArrayOutputStream();
for (String report : reports) {
out.write(report.getBytes("utf-8"));
out.write(LINE_BREAK);
}
return out.toByteArray();
} catch (IOException e) {
//
} finally {
IOUtils.closeQuietly(out);
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy