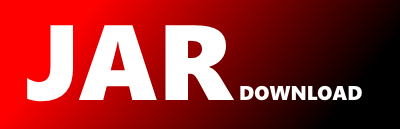
eleme.openapi.sdk.api.entity.finance.PromotionBill Maven / Gradle / Ivy
package eleme.openapi.sdk.api.entity.finance;
import eleme.openapi.sdk.api.enumeration.finance.*;
import eleme.openapi.sdk.api.entity.finance.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class PromotionBill{
/**
* 店铺ID
*/
private Long shopId;
public Long getShopId() {
return shopId;
}
public void setShopId(Long shopId) {
this.shopId = shopId;
}
/**
* 饿了么订单号
*/
private Long elemeOrderId;
public Long getElemeOrderId() {
return elemeOrderId;
}
public void setElemeOrderId(Long elemeOrderId) {
this.elemeOrderId = elemeOrderId;
}
/**
* 推广类型
*/
private Integer promotionType;
public Integer getPromotionType() {
return promotionType;
}
public void setPromotionType(Integer promotionType) {
this.promotionType = promotionType;
}
/**
* 订单类型
*/
private Integer orderType;
public Integer getOrderType() {
return orderType;
}
public void setOrderType(Integer orderType) {
this.orderType = orderType;
}
/**
* 订单创建时间
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date orderCreateTime;
public Date getOrderCreateTime() {
return orderCreateTime;
}
public void setOrderCreateTime(Date orderCreateTime) {
this.orderCreateTime = orderCreateTime;
}
/**
* 订单完成时间
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date orderFinishTime;
public Date getOrderFinishTime() {
return orderFinishTime;
}
public void setOrderFinishTime(Date orderFinishTime) {
this.orderFinishTime = orderFinishTime;
}
/**
* 账单日期
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date billDate;
public Date getBillDate() {
return billDate;
}
public void setBillDate(Date billDate) {
this.billDate = billDate;
}
/**
* 结算日期
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date settleDate;
public Date getSettleDate() {
return settleDate;
}
public void setSettleDate(Date settleDate) {
this.settleDate = settleDate;
}
/**
* 结算状态
*/
private Integer settleStatus;
public Integer getSettleStatus() {
return settleStatus;
}
public void setSettleStatus(Integer settleStatus) {
this.settleStatus = settleStatus;
}
/**
* 结算金额,单位分
*/
private Long settleAmount;
public Long getSettleAmount() {
return settleAmount;
}
public void setSettleAmount(Long settleAmount) {
this.settleAmount = settleAmount;
}
/**
* 结算基数,单位分
*/
private Long settleBaseAmount;
public Long getSettleBaseAmount() {
return settleBaseAmount;
}
public void setSettleBaseAmount(Long settleBaseAmount) {
this.settleBaseAmount = settleBaseAmount;
}
/**
* 抽佣服务费,单位分
*/
private Long commissionFee;
public Long getCommissionFee() {
return commissionFee;
}
public void setCommissionFee(Long commissionFee) {
this.commissionFee = commissionFee;
}
/**
* 招商服务费,单位分
*/
private Long investmentServiceFee;
public Long getInvestmentServiceFee() {
return investmentServiceFee;
}
public void setInvestmentServiceFee(Long investmentServiceFee) {
this.investmentServiceFee = investmentServiceFee;
}
/**
* 奖励金费用,单位分
*/
private Long rewardFee;
public Long getRewardFee() {
return rewardFee;
}
public void setRewardFee(Long rewardFee) {
this.rewardFee = rewardFee;
}
/**
* 评价服务费,单位分
*/
private Long evaluationServiceFee;
public Long getEvaluationServiceFee() {
return evaluationServiceFee;
}
public void setEvaluationServiceFee(Long evaluationServiceFee) {
this.evaluationServiceFee = evaluationServiceFee;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy