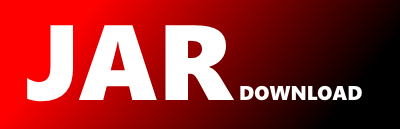
eleme.openapi.sdk.api.entity.invoice.NaposInvoiceInfo Maven / Gradle / Ivy
package eleme.openapi.sdk.api.entity.invoice;
import eleme.openapi.sdk.api.entity.invoice.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class NaposInvoiceInfo{
/**
* 发票类型
*/
private String invoiceType;
public String getInvoiceType() {
return invoiceType;
}
public void setInvoiceType(String invoiceType) {
this.invoiceType = invoiceType;
}
/**
* 发票材质
*/
private String invoiceMaterial;
public String getInvoiceMaterial() {
return invoiceMaterial;
}
public void setInvoiceMaterial(String invoiceMaterial) {
this.invoiceMaterial = invoiceMaterial;
}
/**
* 发票种类
*/
private String invoiceClass;
public String getInvoiceClass() {
return invoiceClass;
}
public void setInvoiceClass(String invoiceClass) {
this.invoiceClass = invoiceClass;
}
/**
* 发票代码
*/
private String invoiceCode;
public String getInvoiceCode() {
return invoiceCode;
}
public void setInvoiceCode(String invoiceCode) {
this.invoiceCode = invoiceCode;
}
/**
* 发票号码
*/
private String invoiceNo;
public String getInvoiceNo() {
return invoiceNo;
}
public void setInvoiceNo(String invoiceNo) {
this.invoiceNo = invoiceNo;
}
/**
* 开票日期
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date invoiceDate;
public Date getInvoiceDate() {
return invoiceDate;
}
public void setInvoiceDate(Date invoiceDate) {
this.invoiceDate = invoiceDate;
}
/**
* 校验码
*/
private String validationCode;
public String getValidationCode() {
return validationCode;
}
public void setValidationCode(String validationCode) {
this.validationCode = validationCode;
}
/**
* 机器编号
*/
private String machineCode;
public String getMachineCode() {
return machineCode;
}
public void setMachineCode(String machineCode) {
this.machineCode = machineCode;
}
/**
* 用户抬头
*/
private NaposUserTitle buyerTitle;
public NaposUserTitle getBuyerTitle() {
return buyerTitle;
}
public void setBuyerTitle(NaposUserTitle buyerTitle) {
this.buyerTitle = buyerTitle;
}
/**
* 销方信息
*/
private NaposSellerInfo sellerInfo;
public NaposSellerInfo getSellerInfo() {
return sellerInfo;
}
public void setSellerInfo(NaposSellerInfo sellerInfo) {
this.sellerInfo = sellerInfo;
}
/**
* 开票总金额(含税总金额)
*/
private BigDecimal amountWithTax;
public BigDecimal getAmountWithTax() {
return amountWithTax;
}
public void setAmountWithTax(BigDecimal amountWithTax) {
this.amountWithTax = amountWithTax;
}
/**
* 不含税总金额
*/
private BigDecimal amountWithoutTax;
public BigDecimal getAmountWithoutTax() {
return amountWithoutTax;
}
public void setAmountWithoutTax(BigDecimal amountWithoutTax) {
this.amountWithoutTax = amountWithoutTax;
}
/**
* 总税额
*/
private BigDecimal taxAmount;
public BigDecimal getTaxAmount() {
return taxAmount;
}
public void setTaxAmount(BigDecimal taxAmount) {
this.taxAmount = taxAmount;
}
/**
* 发票备注
*/
private String memo;
public String getMemo() {
return memo;
}
public void setMemo(String memo) {
this.memo = memo;
}
/**
* 发票PDF文件URL
*/
private String fileUrl;
public String getFileUrl() {
return fileUrl;
}
public void setFileUrl(String fileUrl) {
this.fileUrl = fileUrl;
}
/**
* 发票行列表
*/
private List lineList;
public List getLineList() {
return lineList;
}
public void setLineList(List lineList) {
this.lineList = lineList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy