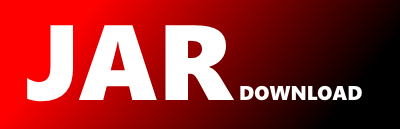
eleme.openapi.sdk.api.entity.product.OSpu Maven / Gradle / Ivy
package eleme.openapi.sdk.api.entity.product;
import eleme.openapi.sdk.api.enumeration.product.*;
import eleme.openapi.sdk.api.entity.product.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class OSpu{
/**
* 品牌标品ID
*/
private Long spuId;
public Long getSpuId() {
return spuId;
}
public void setSpuId(Long spuId) {
this.spuId = spuId;
}
/**
* 连锁总店id
*/
private Long shopId;
public Long getShopId() {
return shopId;
}
public void setShopId(Long shopId) {
this.shopId = shopId;
}
/**
* SPU名称
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* spu类别
*/
private OSpuCategory spuCategory;
public OSpuCategory getSpuCategory() {
return spuCategory;
}
public void setSpuCategory(OSpuCategory spuCategory) {
this.spuCategory = spuCategory;
}
/**
* spu类型
*/
private OSpuType spuType;
public OSpuType getSpuType() {
return spuType;
}
public void setSpuType(OSpuType spuType) {
this.spuType = spuType;
}
/**
* 商品描述
*/
private String description;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
* 图片信息
*/
private List images;
public List getImages() {
return images;
}
public void setImages(List images) {
this.images = images;
}
/**
* spu外部编号
*/
private String spuOutCode;
public String getSpuOutCode() {
return spuOutCode;
}
public void setSpuOutCode(String spuOutCode) {
this.spuOutCode = spuOutCode;
}
/**
* CSPU对应的SPU外部编号
*/
private String parentOutCode;
public String getParentOutCode() {
return parentOutCode;
}
public void setParentOutCode(String parentOutCode) {
this.parentOutCode = parentOutCode;
}
/**
* 菜单外部编号
*/
private String menuOutCode;
public String getMenuOutCode() {
return menuOutCode;
}
public void setMenuOutCode(String menuOutCode) {
this.menuOutCode = menuOutCode;
}
/**
* 类目ID
*/
private Long stdCategoryId;
public Long getStdCategoryId() {
return stdCategoryId;
}
public void setStdCategoryId(Long stdCategoryId) {
this.stdCategoryId = stdCategoryId;
}
/**
* 主料
*/
private List mainMaterials;
public List getMainMaterials() {
return mainMaterials;
}
public void setMainMaterials(List mainMaterials) {
this.mainMaterials = mainMaterials;
}
/**
* 类目属性
*/
private List categoryProperties;
public List getCategoryProperties() {
return categoryProperties;
}
public void setCategoryProperties(List categoryProperties) {
this.categoryProperties = categoryProperties;
}
/**
* 售卖状态
*/
private Integer saleStatus;
public Integer getSaleStatus() {
return saleStatus;
}
public void setSaleStatus(Integer saleStatus) {
this.saleStatus = saleStatus;
}
/**
* 规格
*/
private List specs;
public List getSpecs() {
return specs;
}
public void setSpecs(List specs) {
this.specs = specs;
}
/**
* 商品规格描述
*/
private List specAttrs;
public List getSpecAttrs() {
return specAttrs;
}
public void setSpecAttrs(List specAttrs) {
this.specAttrs = specAttrs;
}
/**
* 配料分组
*/
private List ingredientGroups;
public List getIngredientGroups() {
return ingredientGroups;
}
public void setIngredientGroups(List ingredientGroups) {
this.ingredientGroups = ingredientGroups;
}
/**
* 属性
*/
private List attributes;
public List getAttributes() {
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
/**
* 售卖时间
*/
private OItemSellingTime saleTime;
public OItemSellingTime getSaleTime() {
return saleTime;
}
public void setSaleTime(OItemSellingTime saleTime) {
this.saleTime = saleTime;
}
/**
* 最小购买数量
*/
private Integer minPurchaseQuantity;
public Integer getMinPurchaseQuantity() {
return minPurchaseQuantity;
}
public void setMinPurchaseQuantity(Integer minPurchaseQuantity) {
this.minPurchaseQuantity = minPurchaseQuantity;
}
/**
* 标签
*/
private OLabel labels;
public OLabel getLabels() {
return labels;
}
public void setLabels(OLabel labels) {
this.labels = labels;
}
/**
* 是否单点不送
*/
private Boolean deliverAlone;
public Boolean getDeliverAlone() {
return deliverAlone;
}
public void setDeliverAlone(Boolean deliverAlone) {
this.deliverAlone = deliverAlone;
}
/**
* 是否展示在热销分类
*/
private Boolean joinHot;
public Boolean getJoinHot() {
return joinHot;
}
public void setJoinHot(Boolean joinHot) {
this.joinHot = joinHot;
}
/**
* 是否单独售卖(套餐场景,本字段无需传入)
*/
private Boolean saleAlone;
public Boolean getSaleAlone() {
return saleAlone;
}
public void setSaleAlone(Boolean saleAlone) {
this.saleAlone = saleAlone;
}
/**
* 是否隐藏互斥加料
*/
private Boolean hideMutexSkus;
public Boolean getHideMutexSkus() {
return hideMutexSkus;
}
public void setHideMutexSkus(Boolean hideMutexSkus) {
this.hideMutexSkus = hideMutexSkus;
}
/**
* 是否折叠非必选加料组
*/
private Boolean foldNotRequiredGroup;
public Boolean getFoldNotRequiredGroup() {
return foldNotRequiredGroup;
}
public void setFoldNotRequiredGroup(Boolean foldNotRequiredGroup) {
this.foldNotRequiredGroup = foldNotRequiredGroup;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy