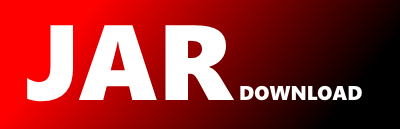
eleme.openapi.sdk.api.service.CardService Maven / Gradle / Ivy
package eleme.openapi.sdk.api.service;
import eleme.openapi.sdk.api.annotation.Service;
import eleme.openapi.sdk.api.base.BaseNopService;
import eleme.openapi.sdk.api.exception.ServiceException;
import eleme.openapi.sdk.oauth.response.Token;
import eleme.openapi.sdk.config.Config;
import eleme.openapi.sdk.api.entity.card.*;
import eleme.openapi.sdk.api.enumeration.card.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.*;
/**
* 商户会员卡服务
*/
@Service("eleme.card")
public class CardService extends BaseNopService {
public CardService(Config config,Token token) {
super(config, token, CardService.class);
}
/**
* 上传图片
*
* @param imageBase64 上传图片
* @return 图片信息
* @throws ServiceException 服务异常
*/
public UploadResult uploadImage(String imageBase64) throws ServiceException {
Map params = new HashMap();
params.put("imageBase64", imageBase64);
return call("eleme.card.uploadImage", params);
}
/**
* 创建模板
*
* @param templateInfo 模板信息
* @return 模板id
* @throws ServiceException 服务异常
*/
public String createTemplate(TemplateInfo templateInfo) throws ServiceException {
Map params = new HashMap();
params.put("templateInfo", templateInfo);
return call("eleme.card.createTemplate", params);
}
/**
* 查询模板信息
*
* @param templateId 模板id列表
* @return 模板信息列表
* @throws ServiceException 服务异常
*/
public Map mgetTemplateInfo(List templateId) throws ServiceException {
Map params = new HashMap();
params.put("templateId", templateId);
return call("eleme.card.mgetTemplateInfo", params);
}
/**
* 更新模板信息
*
* @param templateId 模板id
* @param templateInfo 模板更新信息
* @return 更新后的模板信息
* @throws ServiceException 服务异常
*/
public Map updateTemplate(String templateId, TemplateInfo templateInfo) throws ServiceException {
Map params = new HashMap();
params.put("templateId", templateId);
params.put("templateInfo", templateInfo);
return call("eleme.card.updateTemplate", params);
}
/**
* 查询模板应用的店铺
*
* @param templateId 模板id列表
* @return 模板及应用该模板的店铺
* @throws ServiceException 服务异常
*/
public Map> mgetShopIdsByTemplateIds(List templateId) throws ServiceException {
Map params = new HashMap();
params.put("templateId", templateId);
return call("eleme.card.mgetShopIdsByTemplateIds", params);
}
/**
* 应用模板
*
* @param templateId 模板id
* @param shopIds 店铺列表
* @return 应用成功的店铺集合
* @throws ServiceException 服务异常
*/
public List applyTemplate(String templateId, List shopIds) throws ServiceException {
Map params = new HashMap();
params.put("templateId", templateId);
params.put("shopIds", shopIds);
return call("eleme.card.applyTemplate", params);
}
/**
* 开卡
*
* @param templateId 模板ID
* @param cardUserInfo 会员用户信息
* @param cardAccountInfo 会员账户信息
* @throws ServiceException 服务异常
*/
public void openCard(String templateId, CardUserInfo cardUserInfo, CardAccountInfo cardAccountInfo) throws ServiceException {
Map params = new HashMap();
params.put("templateId", templateId);
params.put("cardUserInfo", cardUserInfo);
params.put("cardAccountInfo", cardAccountInfo);
call("eleme.card.openCard", params);
}
/**
* 更新会员信息
*
* @param cardUserInfo 用户基本信息
* @param cardAccountInfo 用户账户信息
* @throws ServiceException 服务异常
*/
public void updateUserInfo(CardUserInfo cardUserInfo, CardAccountInfo cardAccountInfo) throws ServiceException {
Map params = new HashMap();
params.put("cardUserInfo", cardUserInfo);
params.put("cardAccountInfo", cardAccountInfo);
call("eleme.card.updateUserInfo", params);
}
/**
* 根据userToken获取用户信息(该接口不再使用)
*
* @param userToken userToken有效期10分钟.饿了么app上跳转到外部H5页面https://www.abc.com?accessToken=c8cea843-1fb5-473f-bb10-a9d2aa239c39,其中accessToken为userToken,用其作为该接口的入参获取到用户信息
* @return 用户信息
* @throws ServiceException 服务异常
*/
public CardUserInfo getUserByToken(String userToken) throws ServiceException {
Map params = new HashMap();
params.put("userToken", userToken);
return call("eleme.card.getUserByToken", params);
}
/**
* 确认是否发券接口
*
* @param couponRequest 是否发券请求
* @throws ServiceException 服务异常
*/
public void confirmSendCoupon(CouponRequest couponRequest) throws ServiceException {
Map params = new HashMap();
params.put("couponRequest", couponRequest);
call("eleme.card.confirmSendCoupon", params);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy