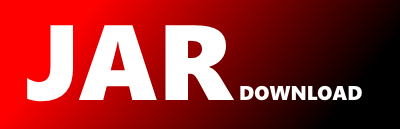
eleme.openapi.sdk.api.service.ContentService Maven / Gradle / Ivy
package eleme.openapi.sdk.api.service;
import eleme.openapi.sdk.api.annotation.Service;
import eleme.openapi.sdk.api.base.BaseNopService;
import eleme.openapi.sdk.api.exception.ServiceException;
import eleme.openapi.sdk.oauth.response.Token;
import eleme.openapi.sdk.config.Config;
import eleme.openapi.sdk.api.entity.content.*;
import eleme.openapi.sdk.api.enumeration.content.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.*;
/**
* 视频服务
*/
@Service("eleme.content")
public class ContentService extends BaseNopService {
public ContentService(Config config,Token token) {
super(config, token, ContentService.class);
}
/**
* 上传视频
*
* @param oVideoInfo 视频信息
* @param shopId 店铺Id
* @param videoType 视频类型
* @return 视频Id
* @throws ServiceException 服务异常
*/
public Long uploadVideo(OVideoInfo oVideoInfo, Long shopId, OVideoTypeEnum videoType) throws ServiceException {
Map params = new HashMap();
params.put("oVideoInfo", oVideoInfo);
params.put("shopId", shopId);
params.put("videoType", videoType);
return call("eleme.content.uploadVideo", params);
}
/**
* 获取efs配置
*
* @param videoType 视频类型
* @return efs配置参数
* @throws ServiceException 服务异常
*/
public OEfsConfig getEfsConfig(OVideoTypeEnum videoType) throws ServiceException {
Map params = new HashMap();
params.put("videoType", videoType);
return call("eleme.content.getEfsConfig", params);
}
/**
* 建立视频与相对应的业务的关联关系
*
* @param videoId 视频Id
* @param bizId 业务Id(如业务类型为GOOD,业务Id为商品Id)
* @param bindBizType 业务类型
* @return 关联的业务关系信息
* @throws ServiceException 服务异常
*/
public OBindInfo setVideoBindRelation(Long videoId, Long bizId, OBindBizEnum bindBizType) throws ServiceException {
Map params = new HashMap();
params.put("videoId", videoId);
params.put("bizId", bizId);
params.put("bindBizType", bindBizType);
return call("eleme.content.setVideoBindRelation", params);
}
/**
* 取消视频与对应业务的关联关系
*
* @param videoId 视频Id
* @param bizId 业务Id(如业务类型为GOOD,业务Id为商品Id)
* @param bindBizType 业务类型
* @throws ServiceException 服务异常
*/
public void unsetVideoBindRelation(Long videoId, Long bizId, OBindBizEnum bindBizType) throws ServiceException {
Map params = new HashMap();
params.put("videoId", videoId);
params.put("bizId", bizId);
params.put("bindBizType", bindBizType);
call("eleme.content.unsetVideoBindRelation", params);
}
/**
* 通过视频id查询视频信息
*
* @param videoId 视频Id
* @return 视频基本信息
* @throws ServiceException 服务异常
*/
public OVideo getVideoInfo(Long videoId) throws ServiceException {
Map params = new HashMap();
params.put("videoId", videoId);
return call("eleme.content.getVideoInfo", params);
}
/**
* 通过视频id获取所有相关联的业务关系
*
* @param videoId 视频Id
* @return 视频关联商品信息
* @throws ServiceException 服务异常
*/
public OVideoBindInfo getVideoBindInfo(Long videoId) throws ServiceException {
Map params = new HashMap();
params.put("videoId", videoId);
return call("eleme.content.getVideoBindInfo", params);
}
/**
* 获取视频上传token
*
* @param scene 场景码
* @return 上传token
* @throws ServiceException 服务异常
*/
public String getUploadToken(ContentSceneEnum scene) throws ServiceException {
Map params = new HashMap();
params.put("scene", scene);
return call("eleme.content.getUploadToken", params);
}
/**
* 发布视频
*
* @param request 内容发布对象
* @return 内容id
* @throws ServiceException 服务异常
*/
public String publishVideoContent(OCreateContentReq request) throws ServiceException {
Map params = new HashMap();
params.put("request", request);
return call("eleme.content.publishVideoContent", params);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy