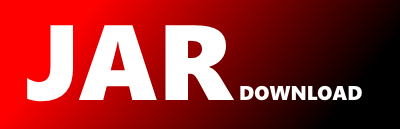
eleme.openapi.sdk.media.common.EncodeUtil Maven / Gradle / Ivy
package eleme.openapi.sdk.media.common;
import eleme.openapi.sdk.utils.Base64;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Objects;
/**
* @author anonymous
*/
public class EncodeUtil {
private static final Charset DEFAULT_CHARSET = StandardCharsets.UTF_8;
private static ThreadLocal TL_DIGEST = new ThreadLocal();
private static final char hexDigits[] = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd',
'e', 'f'};
private static final String HMAC_SHA1 = "HmacSHA1";
// ==========================================MD5==========================================
/**
* 对一个文件获取md5值
*
* @param file 文件
*
* @return md5串
*
* @throws IOException 异常
*
* @throws NoSuchAlgorithmException 异常
*/
public static String encodeWithMD5(File file) throws IOException,NoSuchAlgorithmException {
return encodeWithMD5(file, 2048);
}
/**
* 对一个字符串获取其MD5值
*
* @param s 字符
* @return md5值
* @throws NoSuchAlgorithmException 异常
*/
public static String encodeWithMD5(String s) throws NoSuchAlgorithmException {
return encodeWithMD5(s.getBytes(DEFAULT_CHARSET));
}
private static String encodeWithMD5(File file, int bufferSize) throws IOException, NoSuchAlgorithmException {
FileInputStream fileInputStream = null;
try {
MessageDigest messageDigest = getDigest();
fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[bufferSize];
int length;
while ((length = fileInputStream.read(buffer)) != -1) {
messageDigest.update(buffer, 0, length);
}
return bufferToHex(messageDigest.digest());
} finally {
try {
if (fileInputStream != null)
fileInputStream.close();
} catch (IOException e) {
fileInputStream = null;
//do nothing
}
}
}
public static String encodeWithMD5(byte[] bytes) {
getDigest().update(bytes);
return bufferToHex(getDigest().digest());
}
public static String encodeWithMD5(InputStream is, int bufferSize) throws IOException {
MessageDigest messageDigest = getDigest();
byte[] buffer = new byte[bufferSize];
int length;
while ((length = is.read(buffer)) != -1) {
messageDigest.update(buffer, 0, length);
}
return bufferToHex(messageDigest.digest());
}
private static MessageDigest getDigest() {
MessageDigest digest = TL_DIGEST.get();
if (digest == null) {
try {
digest = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException e) {
//
}
TL_DIGEST.set(digest);
}
return digest;
}
private static String bufferToHex(byte bytes[]) {
return bufferToHex(bytes, 0, bytes.length);
}
private static String bufferToHex(byte bytes[], int m, int n) {
StringBuffer stringbuffer = new StringBuffer(2 * n);
int k = m + n;
for (int l = m; l < k; l++) {
appendHexPair(bytes[l], stringbuffer);
}
return stringbuffer.toString();
}
private static void appendHexPair(byte bt, StringBuffer stringbuffer) {
char c0 = hexDigits[(bt & 0xf0) >> 4];
char c1 = hexDigits[bt & 0xf];
stringbuffer.append(c0);
stringbuffer.append(c1);
}
// ==========================================SHA1==========================================
/**
* 生成签名数据
*
* @param data 待加密的数据
* @param key 加密使用的key
* @return 生成MD5编码的字符串
* @throws InvalidKeyException 异常
* @throws NoSuchAlgorithmException 异常
*/
public static String encodeWithHmacSha1(String data, String key) throws InvalidKeyException,
NoSuchAlgorithmException {
if (data == null || key == null) {
throw new NullPointerException();
}
return encodeWithHmacSha1(data.getBytes(DEFAULT_CHARSET), key.getBytes(DEFAULT_CHARSET));
}
private static String encodeWithHmacSha1(byte[] data, byte[] key) throws InvalidKeyException,
NoSuchAlgorithmException {
if (data == null || key == null) {
throw new NullPointerException();
}
SecretKeySpec signingKey = new SecretKeySpec(key, HMAC_SHA1);
Mac mac = Mac.getInstance(HMAC_SHA1);
mac.init(signingKey);
byte[] rawHmac = mac.doFinal(data);
return bufferToHex(rawHmac);
}
// ==========================================BASE64==========================================
/**
* 通过base64加密
*
* @param arg 请求参数
* @return 加密结果
*/
public static String encodeWithURLSafeBase64(String arg) {
if (arg == null) {
throw new NullPointerException();
}
return removeNewLine(new String(Base64.encodeToByte(arg.getBytes(DEFAULT_CHARSET), false)));
}
/**
* 通过base64加密
*
* @param arg 请求参数
* @return base64值
*/
public static String encodeWithBase64(String arg) {
if (arg == null) {
throw new NullPointerException();
}
return removeNewLine(new String(Base64.encodeToByte(arg.getBytes(DEFAULT_CHARSET), false)));
}
public static byte[] encodeWithBase64(byte[] arg) {
if (arg == null) {
throw new NullPointerException();
}
return Base64.decode(arg);
}
// 修正Java当字符串过长时会添加换行符的问题
private static String removeNewLine(String input) {
if (input == null || input.equals("")) {
return input;
}
input = input.replaceAll("\r|\n", "");
return input;
}
public static String decodeWithURLSafeBase64(String arg) {
if (arg == null) {
throw new NullPointerException();
}
return new String(Objects.requireNonNull(Base64.decode(arg.getBytes(DEFAULT_CHARSET))));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy