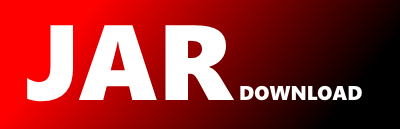
eleme.openapi.sdk.media.utils.CompressUtils Maven / Gradle / Ivy
package eleme.openapi.sdk.media.utils;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
/**
* Created by huamulou on 15/12/10.
*/
public class CompressUtils {
public static byte[] compress(byte[] input) {
ByteArrayInputStream inputStream = new ByteArrayInputStream(input);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
try {
compress(inputStream, outputStream);
} catch (IOException e) {
return null;
}
return outputStream.toByteArray();
}
public static void compress(InputStream is, OutputStream os)
throws IOException {
GZIPOutputStream gos = new GZIPOutputStream(os);
int count;
byte data[] = new byte[1024];
while ((count = is.read(data, 0, 1024)) != -1) {
gos.write(data, 0, count);
}
gos.finish();
gos.flush();
gos.close();
}
public static void decompress(InputStream is, OutputStream os)
throws IOException {
GZIPInputStream gis = new GZIPInputStream(is);
int count;
byte data[] = new byte[1024];
while ((count = gis.read(data, 0, 1024)) != -1) {
os.write(data, 0, count);
}
gis.close();
}
public static byte[] decompress(byte[] input) {
ByteArrayInputStream inputStream = new ByteArrayInputStream(input);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
try {
decompress(inputStream, outputStream);
} catch (IOException e) {
return null;
}
return outputStream.toByteArray();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy