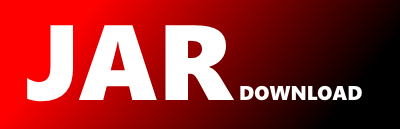
eleme.openapi.sdk.oauth.parser.Converters Maven / Gradle / Ivy
package eleme.openapi.sdk.oauth.parser;
import eleme.openapi.sdk.oauth.OAuthException;
import eleme.openapi.sdk.oauth.mapping.ApiListField;
import eleme.openapi.sdk.oauth.mapping.TokenField;
import eleme.openapi.sdk.oauth.response.ErrorResponse;
import eleme.openapi.sdk.utils.StringUtils;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
public class Converters {
/**
* 是否对JSON返回的数据类型进行校验,默认不校验。给内部测试JSON返回时用的开关。
* 规则:返回的"基本"类型只有String,Long,Boolean,Date,采取严格校验方式,如果类型不匹配,报错
*/
public static boolean isCheckJsonType = false;
private static final Map> baseProps = new HashMap>();
private static final Map fieldCache = new ConcurrentHashMap();
static {
baseProps.put(ErrorResponse.class.getName(), StringUtils.getClassProperties(ErrorResponse.class, false));
}
private Converters() {
}
public static T convert(Class clazz, Reader reader) {
T rsp = null;
try {
rsp = clazz.newInstance();
BeanInfo beanInfo = Introspector.getBeanInfo(clazz);
PropertyDescriptor[] pds = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor pd : pds) {
Method method = pd.getWriteMethod();
if (method == null) { // ignore read-only fields
continue;
}
String itemName = pd.getName();
String listName = null;
Field field = null;
Set stopProps = baseProps.get(clazz.getSuperclass().getName());
if (stopProps != null && stopProps.contains(itemName)) {
field = getField(clazz.getSuperclass(), pd);
} else {
field = getField(clazz, pd);
}
if (field == null) {
continue;
}
TokenField jsonField = field.getAnnotation(TokenField.class);
if (jsonField != null) {
itemName = jsonField.value();
}
ApiListField jsonListField = field.getAnnotation(ApiListField.class);
if (jsonListField != null) {
listName = jsonListField.value();
}
if (!reader.hasReturnField(itemName)) {
if (listName == null || !reader.hasReturnField(listName)) {
continue; // ignore non-return field
}
}
Class> typeClass = field.getType();
// 目前
if (String.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof String) {
method.invoke(rsp, value.toString());
} else {
if (isCheckJsonType && value != null) {
throw new OAuthException(itemName + " is not a String");
}
if (value != null) {
method.invoke(rsp, value.toString());
} else {
method.invoke(rsp, "");
}
}
} else if (Long.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof Long) {
method.invoke(rsp, (Long) value);
} else {
if (isCheckJsonType && value != null) {
throw new OAuthException(itemName + " is not a Number(Long)");
}
if (StringUtils.isNumeric(value)) {
method.invoke(rsp, Long.valueOf(value.toString()));
}
}
} else if (Boolean.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof Boolean) {
method.invoke(rsp, (Boolean) value);
} else {
if (isCheckJsonType && value != null) {
throw new OAuthException(itemName + " is not a Boolean");
}
if (value != null) {
method.invoke(rsp, Boolean.valueOf(value.toString()));
}
}
} else if (Date.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof String) {
method.invoke(rsp, StringUtils.parseDateTime(value.toString()));
}
} else if (List.class.isAssignableFrom(typeClass)) {
Type fieldType = field.getGenericType();
if (fieldType instanceof ParameterizedType) {
ParameterizedType paramType = (ParameterizedType) fieldType;
Type[] genericTypes = paramType.getActualTypeArguments();
if (genericTypes != null && genericTypes.length > 0) {
if (genericTypes[0] instanceof Class>) {
Class> subType = (Class>) genericTypes[0];
List> listObjs = reader.getListObjects(listName, itemName, subType);
if (listObjs != null) {
method.invoke(rsp, listObjs);
}
}
}
}
} else if (Integer.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof Integer) {
method.invoke(rsp, (Integer) value);
} else {
if (isCheckJsonType && value != null) {
throw new OAuthException(itemName + " is not a Number(Integer)");
}
if (StringUtils.isNumeric(value)) {
method.invoke(rsp, Integer.valueOf(value.toString()));
}
}
} else if (Double.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof Double) {
method.invoke(rsp, (Double) value);
} else {
if (isCheckJsonType && value != null) {
throw new OAuthException(itemName + " is not a Double");
}
}
} else if (Number.class.isAssignableFrom(typeClass)) {
Object value = reader.getPrimitiveObject(itemName);
if (value instanceof Number) {
method.invoke(rsp, (Number) value);
} else {
if (isCheckJsonType && value != null) {
throw new OAuthException(itemName + " is not a Number");
}
}
} else {
Object obj = reader.getObject(itemName, typeClass);
if (obj != null) {
method.invoke(rsp, obj);
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return rsp;
}
private static Field getField(Class> clazz, PropertyDescriptor pd) throws Exception {
String key = new StringBuilder(clazz.getName()).append("_").append(pd.getName()).toString();
Field field = fieldCache.get(key);
if (field == null) {// 这个方法不加锁,初始化并发也没关系,无非多put几次
try {
field = clazz.getDeclaredField(pd.getName());
fieldCache.put(key, field);
} catch (NoSuchFieldException e) {
return null;
}
}
return field;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy