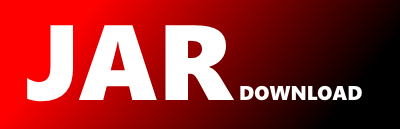
eleme.openapi.sdk.utils.JacksonUtils Maven / Gradle / Ivy
package eleme.openapi.sdk.utils;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import eleme.openapi.sdk.api.exception.JsonParseException;
import java.io.IOException;
import java.util.*;
public class JacksonUtils {
private static final ObjectMapper objectMapper = new ObjectMapper();
static {
//去掉默认的时间戳格式
//objectMapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, false);
//设置为中国上海时区
//objectMapper.setTimeZone(TimeZone.getTimeZone("Asia/Shanghai"));
objectMapper.configure(SerializationFeature.WRITE_NULL_MAP_VALUES, false);
//空值不序列化,入口网关验证签名会产生不一致不能去掉
// objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
//反序列化时,属性不存在的兼容处理
objectMapper.getDeserializationConfig().withoutFeatures(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
objectMapper.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
//单引号处理
objectMapper.configure(com.fasterxml.jackson.core.JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
}
private JacksonUtils() {
}
public static final ObjectMapper getInstance() {
return objectMapper;
}
public static String obj2json(Object obj) {
try {
return objectMapper.writeValueAsString(obj);
} catch (JsonProcessingException e) {
throw new JsonParseException();
}
}
public static T json2pojo(String jsonStr, Class clazz) {
try {
return objectMapper.readValue(jsonStr, clazz);
} catch (IOException e) {
throw new JsonParseException();
}
}
public static T json2pojo(String jsonStr, JavaType javaType) {
try {
return objectMapper.readValue(jsonStr, javaType);
} catch (IOException e) {
throw new JsonParseException();
}
}
public static Map json2map(String jsonStr) {
try {
return objectMapper.readValue(jsonStr, Map.class);
} catch (IOException e) {
throw new JsonParseException();
}
}
public static Map json2map(String jsonStr, Class clazz) {
Map> map;
try {
map = objectMapper.readValue(jsonStr,
new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy