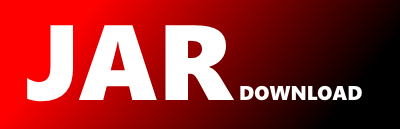
eleme.openapi.sdk.api.entity.finance.FinanceOrderNew Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.entity.finance;
import eleme.openapi.sdk.api.enumeration.finance.*;
import eleme.openapi.sdk.api.entity.finance.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class FinanceOrderNew{
/**
* 饿了么店铺id
*/
private long shopId;
public long getShopId() {
return shopId;
}
public void setShopId(long shopId) {
this.shopId = shopId;
}
/**
* 订单id
*/
private String orderId;
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
/**
* 账单日期
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date billDate;
public Date getBillDate() {
return billDate;
}
public void setBillDate(Date billDate) {
this.billDate = billDate;
}
/**
* 入账日期
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date checkoutDate;
public Date getCheckoutDate() {
return checkoutDate;
}
public void setCheckoutDate(Date checkoutDate) {
this.checkoutDate = checkoutDate;
}
/**
* 订单流水号
*/
private String transNo;
public String getTransNo() {
return transNo;
}
public void setTransNo(String transNo) {
this.transNo = transNo;
}
/**
* 订单类型
*/
private Byte detailType;
public Byte getDetailType() {
return detailType;
}
public void setDetailType(Byte detailType) {
this.detailType = detailType;
}
/**
* 订单子类型
*/
private Byte subDetailType;
public Byte getSubDetailType() {
return subDetailType;
}
public void setSubDetailType(Byte subDetailType) {
this.subDetailType = subDetailType;
}
/**
* 订单类型
*/
private Integer orderType;
public Integer getOrderType() {
return orderType;
}
public void setOrderType(Integer orderType) {
this.orderType = orderType;
}
/**
* 退单类型,只有退单类型有
*/
private Integer refundType;
public Integer getRefundType() {
return refundType;
}
public void setRefundType(Integer refundType) {
this.refundType = refundType;
}
/**
* 结算规则
*/
private Integer engineRule;
public Integer getEngineRule() {
return engineRule;
}
public void setEngineRule(Integer engineRule) {
this.engineRule = engineRule;
}
/**
* 接单序号
*/
private Long daySn;
public Long getDaySn() {
return daySn;
}
public void setDaySn(Long daySn) {
this.daySn = daySn;
}
/**
* 订单创建时间
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date createAt;
public Date getCreateAt() {
return createAt;
}
public void setCreateAt(Date createAt) {
this.createAt = createAt;
}
/**
* 订单完成时间
*/
@JsonFormat(locale = "zh" , timezone="GMT+8")
private Date completeAt;
public Date getCompleteAt() {
return completeAt;
}
public void setCompleteAt(Date completeAt) {
this.completeAt = completeAt;
}
/**
* 结算金额
*/
private BigDecimal checkoutAmount;
public BigDecimal getCheckoutAmount() {
return checkoutAmount;
}
public void setCheckoutAmount(BigDecimal checkoutAmount) {
this.checkoutAmount = checkoutAmount;
}
/**
* 货款
*/
private BigDecimal foodAmount;
public BigDecimal getFoodAmount() {
return foodAmount;
}
public void setFoodAmount(BigDecimal foodAmount) {
this.foodAmount = foodAmount;
}
/**
* 餐盒费
*/
private BigDecimal packingFee;
public BigDecimal getPackingFee() {
return packingFee;
}
public void setPackingFee(BigDecimal packingFee) {
this.packingFee = packingFee;
}
/**
* 赠品补贴
*/
private BigDecimal elemeGiftSubsidyAmount;
public BigDecimal getElemeGiftSubsidyAmount() {
return elemeGiftSubsidyAmount;
}
public void setElemeGiftSubsidyAmount(BigDecimal elemeGiftSubsidyAmount) {
this.elemeGiftSubsidyAmount = elemeGiftSubsidyAmount;
}
/**
* 商户承担活动补贴
*/
private BigDecimal merchantSubsidyAmount;
public BigDecimal getMerchantSubsidyAmount() {
return merchantSubsidyAmount;
}
public void setMerchantSubsidyAmount(BigDecimal merchantSubsidyAmount) {
this.merchantSubsidyAmount = merchantSubsidyAmount;
}
/**
* 商户承担代金券补贴
*/
private BigDecimal merchantCashCoupon;
public BigDecimal getMerchantCashCoupon() {
return merchantCashCoupon;
}
public void setMerchantCashCoupon(BigDecimal merchantCashCoupon) {
this.merchantCashCoupon = merchantCashCoupon;
}
/**
* 用户支付配送费
*/
private BigDecimal userPaidDeliveryFee;
public BigDecimal getUserPaidDeliveryFee() {
return userPaidDeliveryFee;
}
public void setUserPaidDeliveryFee(BigDecimal userPaidDeliveryFee) {
this.userPaidDeliveryFee = userPaidDeliveryFee;
}
/**
* 商户收取配送费
*/
private BigDecimal merchantDeliveryFee;
public BigDecimal getMerchantDeliveryFee() {
return merchantDeliveryFee;
}
public void setMerchantDeliveryFee(BigDecimal merchantDeliveryFee) {
this.merchantDeliveryFee = merchantDeliveryFee;
}
/**
* 商户配送费补贴
*/
private BigDecimal merchantDeliveryCost;
public BigDecimal getMerchantDeliveryCost() {
return merchantDeliveryCost;
}
public void setMerchantDeliveryCost(BigDecimal merchantDeliveryCost) {
this.merchantDeliveryCost = merchantDeliveryCost;
}
/**
* 商户呼单配送费
*/
private BigDecimal merchantCallDeliveryFee;
public BigDecimal getMerchantCallDeliveryFee() {
return merchantCallDeliveryFee;
}
public void setMerchantCallDeliveryFee(BigDecimal merchantCallDeliveryFee) {
this.merchantCallDeliveryFee = merchantCallDeliveryFee;
}
/**
* 呼单小费
*/
private BigDecimal callDeliveryTips;
public BigDecimal getCallDeliveryTips() {
return callDeliveryTips;
}
public void setCallDeliveryTips(BigDecimal callDeliveryTips) {
this.callDeliveryTips = callDeliveryTips;
}
/**
* 服务费费率
*/
private BigDecimal commissionRate;
public BigDecimal getCommissionRate() {
return commissionRate;
}
public void setCommissionRate(BigDecimal commissionRate) {
this.commissionRate = commissionRate;
}
/**
* 满额保底价
*/
private BigDecimal fullGuaranteeFee;
public BigDecimal getFullGuaranteeFee() {
return fullGuaranteeFee;
}
public void setFullGuaranteeFee(BigDecimal fullGuaranteeFee) {
this.fullGuaranteeFee = fullGuaranteeFee;
}
/**
* 服务费
*/
private BigDecimal commissionFee;
public BigDecimal getCommissionFee() {
return commissionFee;
}
public void setCommissionFee(BigDecimal commissionFee) {
this.commissionFee = commissionFee;
}
/**
* 取消赔偿费率
*/
private BigDecimal compensationRate;
public BigDecimal getCompensationRate() {
return compensationRate;
}
public void setCompensationRate(BigDecimal compensationRate) {
this.compensationRate = compensationRate;
}
/**
* 用户申请退单金额
*/
private BigDecimal refundAmount;
public BigDecimal getRefundAmount() {
return refundAmount;
}
public void setRefundAmount(BigDecimal refundAmount) {
this.refundAmount = refundAmount;
}
/**
* 取消呼单赔付金额
*/
private BigDecimal cancelledCallDeliveryAmount;
public BigDecimal getCancelledCallDeliveryAmount() {
return cancelledCallDeliveryAmount;
}
public void setCancelledCallDeliveryAmount(BigDecimal cancelledCallDeliveryAmount) {
this.cancelledCallDeliveryAmount = cancelledCallDeliveryAmount;
}
/**
* 饿了么承担活动补贴
*/
private BigDecimal elemeSubsidyAmount;
public BigDecimal getElemeSubsidyAmount() {
return elemeSubsidyAmount;
}
public void setElemeSubsidyAmount(BigDecimal elemeSubsidyAmount) {
this.elemeSubsidyAmount = elemeSubsidyAmount;
}
/**
* 饿了么承担代金券补贴
*/
private BigDecimal elemeCashCoupon;
public BigDecimal getElemeCashCoupon() {
return elemeCashCoupon;
}
public void setElemeCashCoupon(BigDecimal elemeCashCoupon) {
this.elemeCashCoupon = elemeCashCoupon;
}
/**
* 实际配送类型
*/
private Integer actualDeliveryType;
public Integer getActualDeliveryType() {
return actualDeliveryType;
}
public void setActualDeliveryType(Integer actualDeliveryType) {
this.actualDeliveryType = actualDeliveryType;
}
/**
* 备注
*/
private String comment;
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
/**
* 服务包ID
*/
private Integer zionServiceType;
public Integer getZionServiceType() {
return zionServiceType;
}
public void setZionServiceType(Integer zionServiceType) {
this.zionServiceType = zionServiceType;
}
/**
* 冷链配送
*/
private BigDecimal coldDeliveryFee;
public BigDecimal getColdDeliveryFee() {
return coldDeliveryFee;
}
public void setColdDeliveryFee(BigDecimal coldDeliveryFee) {
this.coldDeliveryFee = coldDeliveryFee;
}
/**
* 数据来源
*/
private String comeFromDesc;
public String getComeFromDesc() {
return comeFromDesc;
}
public void setComeFromDesc(String comeFromDesc) {
this.comeFromDesc = comeFromDesc;
}
/**
* cps扣费名称
*/
private String cpsName;
public String getCpsName() {
return cpsName;
}
public void setCpsName(String cpsName) {
this.cpsName = cpsName;
}
/**
* CPS扣费金额
*/
private BigDecimal cpsAmount;
public BigDecimal getCpsAmount() {
return cpsAmount;
}
public void setCpsAmount(BigDecimal cpsAmount) {
this.cpsAmount = cpsAmount;
}
/**
* 平台津贴金额
*/
private BigDecimal platformAllowanceAmount;
public BigDecimal getPlatformAllowanceAmount() {
return platformAllowanceAmount;
}
public void setPlatformAllowanceAmount(BigDecimal platformAllowanceAmount) {
this.platformAllowanceAmount = platformAllowanceAmount;
}
/**
* 商户津贴金额
*/
private BigDecimal merchantAllowanceAmount;
public BigDecimal getMerchantAllowanceAmount() {
return merchantAllowanceAmount;
}
public void setMerchantAllowanceAmount(BigDecimal merchantAllowanceAmount) {
this.merchantAllowanceAmount = merchantAllowanceAmount;
}
/**
* 代理商津贴金额
*/
private BigDecimal agentAllowanceAmount;
public BigDecimal getAgentAllowanceAmount() {
return agentAllowanceAmount;
}
public void setAgentAllowanceAmount(BigDecimal agentAllowanceAmount) {
this.agentAllowanceAmount = agentAllowanceAmount;
}
/**
* 智能满减服务费
*/
private BigDecimal allowanceServiceAmount;
public BigDecimal getAllowanceServiceAmount() {
return allowanceServiceAmount;
}
public void setAllowanceServiceAmount(BigDecimal allowanceServiceAmount) {
this.allowanceServiceAmount = allowanceServiceAmount;
}
/**
* 基础物流费
*/
private BigDecimal baseLogisticsAmount;
public BigDecimal getBaseLogisticsAmount() {
return baseLogisticsAmount;
}
public void setBaseLogisticsAmount(BigDecimal baseLogisticsAmount) {
this.baseLogisticsAmount = baseLogisticsAmount;
}
/**
* 时段加价
*/
private BigDecimal timePriceUp;
public BigDecimal getTimePriceUp() {
return timePriceUp;
}
public void setTimePriceUp(BigDecimal timePriceUp) {
this.timePriceUp = timePriceUp;
}
/**
* 用户改地址配送费
*/
private BigDecimal changeAddressDeliveryFee;
public BigDecimal getChangeAddressDeliveryFee() {
return changeAddressDeliveryFee;
}
public void setChangeAddressDeliveryFee(BigDecimal changeAddressDeliveryFee) {
this.changeAddressDeliveryFee = changeAddressDeliveryFee;
}
/**
* 订单来源
*/
private Integer orderComeFrom;
public Integer getOrderComeFrom() {
return orderComeFrom;
}
public void setOrderComeFrom(Integer orderComeFrom) {
this.orderComeFrom = orderComeFrom;
}
/**
* 业务类型
*/
private Integer orderBusinessType;
public Integer getOrderBusinessType() {
return orderBusinessType;
}
public void setOrderBusinessType(Integer orderBusinessType) {
this.orderBusinessType = orderBusinessType;
}
/**
* 增值服务费(店铺推广)
*/
private BigDecimal cpcAmount;
public BigDecimal getCpcAmount() {
return cpcAmount;
}
public void setCpcAmount(BigDecimal cpcAmount) {
this.cpcAmount = cpcAmount;
}
/**
* 增值服务费(返还至商家推广账户)
*/
private BigDecimal cpcIndividualAmount;
public BigDecimal getCpcIndividualAmount() {
return cpcIndividualAmount;
}
public void setCpcIndividualAmount(BigDecimal cpcIndividualAmount) {
this.cpcIndividualAmount = cpcIndividualAmount;
}
/**
* 增值服务费(返还至连锁推广账户)
*/
private BigDecimal cpcHeadStoreAmount;
public BigDecimal getCpcHeadStoreAmount() {
return cpcHeadStoreAmount;
}
public void setCpcHeadStoreAmount(BigDecimal cpcHeadStoreAmount) {
this.cpcHeadStoreAmount = cpcHeadStoreAmount;
}
/**
* 商户运费券补贴
*/
private BigDecimal merchantDeliveryCoupon;
public BigDecimal getMerchantDeliveryCoupon() {
return merchantDeliveryCoupon;
}
public void setMerchantDeliveryCoupon(BigDecimal merchantDeliveryCoupon) {
this.merchantDeliveryCoupon = merchantDeliveryCoupon;
}
/**
* 实收商家配送费
*/
private BigDecimal ffmTotalAmt;
public BigDecimal getFfmTotalAmt() {
return ffmTotalAmt;
}
public void setFfmTotalAmt(BigDecimal ffmTotalAmt) {
this.ffmTotalAmt = ffmTotalAmt;
}
/**
* 增值-弱驻店服务费
*/
private BigDecimal ffmShopInAmt;
public BigDecimal getFfmShopInAmt() {
return ffmShopInAmt;
}
public void setFfmShopInAmt(BigDecimal ffmShopInAmt) {
this.ffmShopInAmt = ffmShopInAmt;
}
/**
* 增值-慢必赔服务费
*/
private BigDecimal ffmSlowWillPayAmt;
public BigDecimal getFfmSlowWillPayAmt() {
return ffmSlowWillPayAmt;
}
public void setFfmSlowWillPayAmt(BigDecimal ffmSlowWillPayAmt) {
this.ffmSlowWillPayAmt = ffmSlowWillPayAmt;
}
/**
* 距离加价
*/
private BigDecimal distancePriceUp;
public BigDecimal getDistancePriceUp() {
return distancePriceUp;
}
public void setDistancePriceUp(BigDecimal distancePriceUp) {
this.distancePriceUp = distancePriceUp;
}
/**
* 价格加价
*/
private BigDecimal costAdditionalPrice;
public BigDecimal getCostAdditionalPrice() {
return costAdditionalPrice;
}
public void setCostAdditionalPrice(BigDecimal costAdditionalPrice) {
this.costAdditionalPrice = costAdditionalPrice;
}
/**
* 增值服务费
*/
private BigDecimal additionServiceAmount;
public BigDecimal getAdditionServiceAmount() {
return additionServiceAmount;
}
public void setAdditionServiceAmount(BigDecimal additionServiceAmount) {
this.additionServiceAmount = additionServiceAmount;
}
/**
* 募捐金额
*/
private BigDecimal contributionAmount;
public BigDecimal getContributionAmount() {
return contributionAmount;
}
public void setContributionAmount(BigDecimal contributionAmount) {
this.contributionAmount = contributionAmount;
}
/**
* 代理商配送费券补贴费
*/
private BigDecimal familyDeliCouponSubsidyAmt;
public BigDecimal getFamilyDeliCouponSubsidyAmt() {
return familyDeliCouponSubsidyAmt;
}
public void setFamilyDeliCouponSubsidyAmt(BigDecimal familyDeliCouponSubsidyAmt) {
this.familyDeliCouponSubsidyAmt = familyDeliCouponSubsidyAmt;
}
/**
* 代理商配送费活动补贴费
*/
private BigDecimal familyDeliActiSubsidyAmt;
public BigDecimal getFamilyDeliActiSubsidyAmt() {
return familyDeliActiSubsidyAmt;
}
public void setFamilyDeliActiSubsidyAmt(BigDecimal familyDeliActiSubsidyAmt) {
this.familyDeliActiSubsidyAmt = familyDeliActiSubsidyAmt;
}
/**
* 代理商代金券补贴
*/
private BigDecimal familyCashCouponAmount;
public BigDecimal getFamilyCashCouponAmount() {
return familyCashCouponAmount;
}
public void setFamilyCashCouponAmount(BigDecimal familyCashCouponAmount) {
this.familyCashCouponAmount = familyCashCouponAmount;
}
/**
* 平台配送费券补贴费
*/
private BigDecimal eleDeliCouponSubsidyAmt;
public BigDecimal getEleDeliCouponSubsidyAmt() {
return eleDeliCouponSubsidyAmt;
}
public void setEleDeliCouponSubsidyAmt(BigDecimal eleDeliCouponSubsidyAmt) {
this.eleDeliCouponSubsidyAmt = eleDeliCouponSubsidyAmt;
}
/**
* 平台配送费活动补贴费
*/
private BigDecimal eleDeliActiSubsidyAmt;
public BigDecimal getEleDeliActiSubsidyAmt() {
return eleDeliActiSubsidyAmt;
}
public void setEleDeliActiSubsidyAmt(BigDecimal eleDeliActiSubsidyAmt) {
this.eleDeliActiSubsidyAmt = eleDeliActiSubsidyAmt;
}
/**
* 代运营费用信息
*/
private AgentCommissionInfo agentCommissionInfo;
public AgentCommissionInfo getAgentCommissionInfo() {
return agentCommissionInfo;
}
public void setAgentCommissionInfo(AgentCommissionInfo agentCommissionInfo) {
this.agentCommissionInfo = agentCommissionInfo;
}
/**
* 联盟推广费用信息
*/
private PromotionCommissionInfo promotionCommissionInfo;
public PromotionCommissionInfo getPromotionCommissionInfo() {
return promotionCommissionInfo;
}
public void setPromotionCommissionInfo(PromotionCommissionInfo promotionCommissionInfo) {
this.promotionCommissionInfo = promotionCommissionInfo;
}
/**
* 保险费用明细(废弃)
*/
private InsuranceFeeInfo insuranceFeeInfo;
public InsuranceFeeInfo getInsuranceFeeInfo() {
return insuranceFeeInfo;
}
public void setInsuranceFeeInfo(InsuranceFeeInfo insuranceFeeInfo) {
this.insuranceFeeInfo = insuranceFeeInfo;
}
/**
* 保险费用明细(新)
*/
private List insuranceFeeInfoList;
public List getInsuranceFeeInfoList() {
return insuranceFeeInfoList;
}
public void setInsuranceFeeInfoList(List insuranceFeeInfoList) {
this.insuranceFeeInfoList = insuranceFeeInfoList;
}
/**
* 卡券相关信息
*/
private List ticketSettleInfoList;
public List getTicketSettleInfoList() {
return ticketSettleInfoList;
}
public void setTicketSettleInfoList(List ticketSettleInfoList) {
this.ticketSettleInfoList = ticketSettleInfoList;
}
/**
* 技术服务费—优惠总金额
*/
private BigDecimal totalReducedAmount;
public BigDecimal getTotalReducedAmount() {
return totalReducedAmount;
}
public void setTotalReducedAmount(BigDecimal totalReducedAmount) {
this.totalReducedAmount = totalReducedAmount;
}
/**
* 高客单优惠(浮动费率场景)—优惠金额
*/
private BigDecimal highPriceReducedAmount;
public BigDecimal getHighPriceReducedAmount() {
return highPriceReducedAmount;
}
public void setHighPriceReducedAmount(BigDecimal highPriceReducedAmount) {
this.highPriceReducedAmount = highPriceReducedAmount;
}
/**
* 高客单优惠(浮动费率场景)—基准费用
*/
private BigDecimal highPriceReducedThreshold;
public BigDecimal getHighPriceReducedThreshold() {
return highPriceReducedThreshold;
}
public void setHighPriceReducedThreshold(BigDecimal highPriceReducedThreshold) {
this.highPriceReducedThreshold = highPriceReducedThreshold;
}
/**
* 高客单优惠(浮动费率场景)—优惠比例
*/
private BigDecimal highPriceReducedRate;
public BigDecimal getHighPriceReducedRate() {
return highPriceReducedRate;
}
public void setHighPriceReducedRate(BigDecimal highPriceReducedRate) {
this.highPriceReducedRate = highPriceReducedRate;
}
/**
* 高客单优惠(浮动费率场景)—限额比例
*/
private BigDecimal highPriceReducedTopRate;
public BigDecimal getHighPriceReducedTopRate() {
return highPriceReducedTopRate;
}
public void setHighPriceReducedTopRate(BigDecimal highPriceReducedTopRate) {
this.highPriceReducedTopRate = highPriceReducedTopRate;
}
/**
* 高客单优惠(固定费率场景)—优惠限额
*/
private BigDecimal menBaoReducedAmount;
public BigDecimal getMenBaoReducedAmount() {
return menBaoReducedAmount;
}
public void setMenBaoReducedAmount(BigDecimal menBaoReducedAmount) {
this.menBaoReducedAmount = menBaoReducedAmount;
}
/**
* 高客单优惠(固定费率场景)—优惠比例
*/
private BigDecimal menBaoReducedRate;
public BigDecimal getMenBaoReducedRate() {
return menBaoReducedRate;
}
public void setMenBaoReducedRate(BigDecimal menBaoReducedRate) {
this.menBaoReducedRate = menBaoReducedRate;
}
/**
* 高客单优惠(固定费率场景)—优惠金额
*/
private BigDecimal menBaoReducedTopAmount;
public BigDecimal getMenBaoReducedTopAmount() {
return menBaoReducedTopAmount;
}
public void setMenBaoReducedTopAmount(BigDecimal menBaoReducedTopAmount) {
this.menBaoReducedTopAmount = menBaoReducedTopAmount;
}
/**
* 通用优惠—优惠比例
*/
private BigDecimal commonReducedRate;
public BigDecimal getCommonReducedRate() {
return commonReducedRate;
}
public void setCommonReducedRate(BigDecimal commonReducedRate) {
this.commonReducedRate = commonReducedRate;
}
/**
* 通用优惠—优惠金额
*/
private BigDecimal commonReducedAmount;
public BigDecimal getCommonReducedAmount() {
return commonReducedAmount;
}
public void setCommonReducedAmount(BigDecimal commonReducedAmount) {
this.commonReducedAmount = commonReducedAmount;
}
/**
* 智能特价菜金额
*/
private BigDecimal skuAllowanceServiceAmount;
public BigDecimal getSkuAllowanceServiceAmount() {
return skuAllowanceServiceAmount;
}
public void setSkuAllowanceServiceAmount(BigDecimal skuAllowanceServiceAmount) {
this.skuAllowanceServiceAmount = skuAllowanceServiceAmount;
}
/**
* 爆单红包增量出资
*/
private BigDecimal promotionActivityPackageAmount;
public BigDecimal getPromotionActivityPackageAmount() {
return promotionActivityPackageAmount;
}
public void setPromotionActivityPackageAmount(BigDecimal promotionActivityPackageAmount) {
this.promotionActivityPackageAmount = promotionActivityPackageAmount;
}
/**
* 先享后付服务费
*/
private BigDecimal userPayLaterServiceAmount;
public BigDecimal getUserPayLaterServiceAmount() {
return userPayLaterServiceAmount;
}
public void setUserPayLaterServiceAmount(BigDecimal userPayLaterServiceAmount) {
this.userPayLaterServiceAmount = userPayLaterServiceAmount;
}
/**
* 商品定价抽佣优惠金额
*/
private BigDecimal goodsPriceCommissionDiscountAmount;
public BigDecimal getGoodsPriceCommissionDiscountAmount() {
return goodsPriceCommissionDiscountAmount;
}
public void setGoodsPriceCommissionDiscountAmount(BigDecimal goodsPriceCommissionDiscountAmount) {
this.goodsPriceCommissionDiscountAmount = goodsPriceCommissionDiscountAmount;
}
/**
* 商品定价抽佣优惠商品列表
*/
private List goodsPriceDiscountSubOrderInfoList;
public List getGoodsPriceDiscountSubOrderInfoList() {
return goodsPriceDiscountSubOrderInfoList;
}
public void setGoodsPriceDiscountSubOrderInfoList(List goodsPriceDiscountSubOrderInfoList) {
this.goodsPriceDiscountSubOrderInfoList = goodsPriceDiscountSubOrderInfoList;
}
/**
* 低起送服务优惠,单位元
*/
private BigDecimal belowMinDeliveryAmountDiscountAmount;
public BigDecimal getBelowMinDeliveryAmountDiscountAmount() {
return belowMinDeliveryAmountDiscountAmount;
}
public void setBelowMinDeliveryAmountDiscountAmount(BigDecimal belowMinDeliveryAmountDiscountAmount) {
this.belowMinDeliveryAmountDiscountAmount = belowMinDeliveryAmountDiscountAmount;
}
/**
* 低起送服务优惠基数,单位元
*/
private BigDecimal belowMinDeliveryAmountDiscountBaseAmount;
public BigDecimal getBelowMinDeliveryAmountDiscountBaseAmount() {
return belowMinDeliveryAmountDiscountBaseAmount;
}
public void setBelowMinDeliveryAmountDiscountBaseAmount(BigDecimal belowMinDeliveryAmountDiscountBaseAmount) {
this.belowMinDeliveryAmountDiscountBaseAmount = belowMinDeliveryAmountDiscountBaseAmount;
}
/**
* 低起送服务优惠比例
*/
private BigDecimal belowMinDeliveryAmountDiscountRate;
public BigDecimal getBelowMinDeliveryAmountDiscountRate() {
return belowMinDeliveryAmountDiscountRate;
}
public void setBelowMinDeliveryAmountDiscountRate(BigDecimal belowMinDeliveryAmountDiscountRate) {
this.belowMinDeliveryAmountDiscountRate = belowMinDeliveryAmountDiscountRate;
}
/**
* 技术服务费叠加折扣费率
*/
private BigDecimal commissionServiceFeeAdditionalDiscountRate;
public BigDecimal getCommissionServiceFeeAdditionalDiscountRate() {
return commissionServiceFeeAdditionalDiscountRate;
}
public void setCommissionServiceFeeAdditionalDiscountRate(BigDecimal commissionServiceFeeAdditionalDiscountRate) {
this.commissionServiceFeeAdditionalDiscountRate = commissionServiceFeeAdditionalDiscountRate;
}
/**
* 配送服务费叠加折扣费率
*/
private BigDecimal deliveryServiceFeeAdditionalDiscountRate;
public BigDecimal getDeliveryServiceFeeAdditionalDiscountRate() {
return deliveryServiceFeeAdditionalDiscountRate;
}
public void setDeliveryServiceFeeAdditionalDiscountRate(BigDecimal deliveryServiceFeeAdditionalDiscountRate) {
this.deliveryServiceFeeAdditionalDiscountRate = deliveryServiceFeeAdditionalDiscountRate;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy