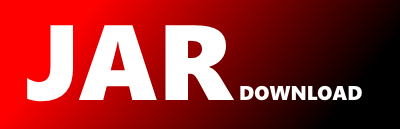
eleme.openapi.sdk.api.entity.order.Item Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.entity.order;
import eleme.openapi.sdk.api.enumeration.order.*;
import eleme.openapi.sdk.api.entity.order.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class Item{
/**
* 商品名称
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* 商品数量,注意:金额退时会根据金额折换份数(向下取整),当不足一份时此值为0
*/
private int quantity;
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
/**
* 商品单价
*/
private double price;
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
/**
* 商品商户侧价格
*/
private double shopPrice;
public double getShopPrice() {
return shopPrice;
}
public void setShopPrice(double shopPrice) {
this.shopPrice = shopPrice;
}
/**
* 商品用户侧价格
*/
private double userPrice;
public double getUserPrice() {
return userPrice;
}
public void setUserPrice(double userPrice) {
this.userPrice = userPrice;
}
/**
* 商品vfoodId
*/
private long vfoodId;
public long getVfoodId() {
return vfoodId;
}
public void setVfoodId(long vfoodId) {
this.vfoodId = vfoodId;
}
/**
* 菜品skuId
*/
private String skuId;
public String getSkuId() {
return skuId;
}
public void setSkuId(String skuId) {
this.skuId = skuId;
}
/**
* 旧菜品规格id
*/
private String specId;
public String getSpecId() {
return specId;
}
public void setSpecId(String specId) {
this.specId = specId;
}
/**
* 菜品扩展码
*/
private String extendCode;
public String getExtendCode() {
return extendCode;
}
public void setExtendCode(String extendCode) {
this.extendCode = extendCode;
}
/**
* 餐盒费
*/
private double packingBoxFee;
public double getPackingBoxFee() {
return packingBoxFee;
}
public void setPackingBoxFee(double packingBoxFee) {
this.packingBoxFee = packingBoxFee;
}
/**
* 餐盒费数量
*/
private int packingBoxQuantity;
public int getPackingBoxQuantity() {
return packingBoxQuantity;
}
public void setPackingBoxQuantity(int packingBoxQuantity) {
this.packingBoxQuantity = packingBoxQuantity;
}
/**
* 商品唯一ID
*/
private String uniqueId;
public String getUniqueId() {
return uniqueId;
}
public void setUniqueId(String uniqueId) {
this.uniqueId = uniqueId;
}
/**
* 退款单位类型,类型为UNIT取quantity字段,类型为AMOUNT取money字段(注意如果已经退完,此字段为空)
*/
private String quantityType;
public String getQuantityType() {
return quantityType;
}
public void setQuantityType(String quantityType) {
this.quantityType = quantityType;
}
/**
* 退款金额(元)(注意:如果已经退完,此字段为空)
*/
private Double money;
public Double getMoney() {
return money;
}
public void setMoney(Double money) {
this.money = money;
}
/**
* 是否为配料(注意:如果已经退完,此字段为空)
*/
private Boolean batching;
public Boolean getBatching() {
return batching;
}
public void setBatching(Boolean batching) {
this.batching = batching;
}
/**
* 套餐子商品规格
*/
private List newSpecs;
public List getNewSpecs() {
return newSpecs;
}
public void setNewSpecs(List newSpecs) {
this.newSpecs = newSpecs;
}
/**
* 配料
*/
private List ingredients;
public List getIngredients() {
return ingredients;
}
public void setIngredients(List ingredients) {
this.ingredients = ingredients;
}
/**
* 套餐子商品
*/
private List> foodGroup;
public List> getFoodGroup() {
return foodGroup;
}
public void setFoodGroup(List> foodGroup) {
this.foodGroup = foodGroup;
}
/**
* 商品参与的活动
*/
private List activities;
public List getActivities() {
return activities;
}
public void setActivities(List activities) {
this.activities = activities;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy