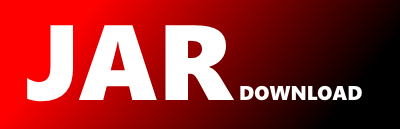
eleme.openapi.sdk.api.entity.order.RefundFoodItem Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.entity.order;
import eleme.openapi.sdk.api.enumeration.order.*;
import eleme.openapi.sdk.api.entity.order.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class RefundFoodItem{
/**
* 商品名称
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* 商品数量
*/
private int quantity;
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
/**
* 商品单价
*/
private double price;
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
/**
* 商品商户侧价格
*/
private double shopPrice;
public double getShopPrice() {
return shopPrice;
}
public void setShopPrice(double shopPrice) {
this.shopPrice = shopPrice;
}
/**
* 商品用户侧价格
*/
private double userPrice;
public double getUserPrice() {
return userPrice;
}
public void setUserPrice(double userPrice) {
this.userPrice = userPrice;
}
/**
* 商品vfoodId
*/
private long vfoodId;
public long getVfoodId() {
return vfoodId;
}
public void setVfoodId(long vfoodId) {
this.vfoodId = vfoodId;
}
/**
* 菜品skuId
*/
private String skuId;
public String getSkuId() {
return skuId;
}
public void setSkuId(String skuId) {
this.skuId = skuId;
}
/**
* 旧菜品规格id
*/
private String specId;
public String getSpecId() {
return specId;
}
public void setSpecId(String specId) {
this.specId = specId;
}
/**
* 菜品扩展码
*/
private String extendCode;
public String getExtendCode() {
return extendCode;
}
public void setExtendCode(String extendCode) {
this.extendCode = extendCode;
}
/**
* 商品唯一ID
*/
private String uniqueId;
public String getUniqueId() {
return uniqueId;
}
public void setUniqueId(String uniqueId) {
this.uniqueId = uniqueId;
}
/**
* 菜品总份数
*/
private int totalUnit;
public int getTotalUnit() {
return totalUnit;
}
public void setTotalUnit(int totalUnit) {
this.totalUnit = totalUnit;
}
/**
* 可退份数
*/
private int refundableUnit;
public int getRefundableUnit() {
return refundableUnit;
}
public void setRefundableUnit(int refundableUnit) {
this.refundableUnit = refundableUnit;
}
/**
* 已退份数
*/
private int refundedUnit;
public int getRefundedUnit() {
return refundedUnit;
}
public void setRefundedUnit(int refundedUnit) {
this.refundedUnit = refundedUnit;
}
/**
* 预计可退金额(单位:元)(注意仅当支持金额退时才会返回此字段)
*/
private double refundableMoney;
public double getRefundableMoney() {
return refundableMoney;
}
public void setRefundableMoney(double refundableMoney) {
this.refundableMoney = refundableMoney;
}
/**
* 已退金额(单位:元)
*/
private double refundedMoney;
public double getRefundedMoney() {
return refundedMoney;
}
public void setRefundedMoney(double refundedMoney) {
this.refundedMoney = refundedMoney;
}
/**
* 支持的退款单位类型(注意有可能为空,表示不支持部分退款)
*/
private List supportRefundQuantityType;
public List getSupportRefundQuantityType() {
return supportRefundQuantityType;
}
public void setSupportRefundQuantityType(List supportRefundQuantityType) {
this.supportRefundQuantityType = supportRefundQuantityType;
}
/**
* 是否为配料
*/
private Boolean batching;
public Boolean getBatching() {
return batching;
}
public void setBatching(Boolean batching) {
this.batching = batching;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy