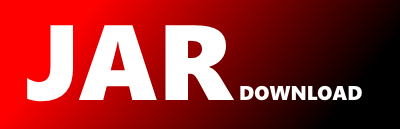
eleme.openapi.sdk.api.entity.product.OItem Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.entity.product;
import eleme.openapi.sdk.api.enumeration.product.*;
import eleme.openapi.sdk.api.entity.product.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class OItem{
/**
* 商品描述
*/
private String description;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
* 商品Id
*/
private long id;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
/**
* 商品名
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* 是否有效
*/
private int isValid;
public int getIsValid() {
return isValid;
}
public void setIsValid(int isValid) {
this.isValid = isValid;
}
/**
* 最近一个月的售出份数
*/
private int recentPopularity;
public int getRecentPopularity() {
return recentPopularity;
}
public void setRecentPopularity(int recentPopularity) {
this.recentPopularity = recentPopularity;
}
/**
* 最近一个月售出份数的数量级
*/
private String fuzzyRecentPopularity;
public String getFuzzyRecentPopularity() {
return fuzzyRecentPopularity;
}
public void setFuzzyRecentPopularity(String fuzzyRecentPopularity) {
this.fuzzyRecentPopularity = fuzzyRecentPopularity;
}
/**
* 商品分类Id
*/
private long categoryId;
public long getCategoryId() {
return categoryId;
}
public void setCategoryId(long categoryId) {
this.categoryId = categoryId;
}
/**
* 店铺Id
*/
private long shopId;
public long getShopId() {
return shopId;
}
public void setShopId(long shopId) {
this.shopId = shopId;
}
/**
* 店铺名称
*/
private String shopName;
public String getShopName() {
return shopName;
}
public void setShopName(String shopName) {
this.shopName = shopName;
}
/**
* 商品主图片
*/
private String imageUrl;
public String getImageUrl() {
return imageUrl;
}
public void setImageUrl(String imageUrl) {
this.imageUrl = imageUrl;
}
/**
* 标签
*/
private OLabel labels;
public OLabel getLabels() {
return labels;
}
public void setLabels(OLabel labels) {
this.labels = labels;
}
/**
* 规格的列表
*/
private List specs;
public List getSpecs() {
return specs;
}
public void setSpecs(List specs) {
this.specs = specs;
}
/**
* 售卖时间
*/
private OItemSellingTime sellingTime;
public OItemSellingTime getSellingTime() {
return sellingTime;
}
public void setSellingTime(OItemSellingTime sellingTime) {
this.sellingTime = sellingTime;
}
/**
* 属性
*/
private List attributes;
public List getAttributes() {
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
/**
* 规格属性
*/
private List attributesV2;
public List getAttributesV2() {
return attributesV2;
}
public void setAttributesV2(List attributesV2) {
this.attributesV2 = attributesV2;
}
/**
* 后台类目ID
*/
private long backCategoryId;
public long getBackCategoryId() {
return backCategoryId;
}
public void setBackCategoryId(long backCategoryId) {
this.backCategoryId = backCategoryId;
}
/**
* 商品最小购买量
*/
private int minPurchaseQuantity;
public int getMinPurchaseQuantity() {
return minPurchaseQuantity;
}
public void setMinPurchaseQuantity(int minPurchaseQuantity) {
this.minPurchaseQuantity = minPurchaseQuantity;
}
/**
* 商品单位
*/
private String unit;
public String getUnit() {
return unit;
}
public void setUnit(String unit) {
this.unit = unit;
}
/**
* 商品套餐标签
*/
private Integer setMeal;
public Integer getSetMeal() {
return setMeal;
}
public void setSetMeal(Integer setMeal) {
this.setMeal = setMeal;
}
/**
* 原材料
*/
private List materials;
public List getMaterials() {
return materials;
}
public void setMaterials(List materials) {
this.materials = materials;
}
/**
* 后台类目ID
*/
private long stdCategoryId;
public long getStdCategoryId() {
return stdCategoryId;
}
public void setStdCategoryId(long stdCategoryId) {
this.stdCategoryId = stdCategoryId;
}
/**
* 主料
*/
private List mainMaterials;
public List getMainMaterials() {
return mainMaterials;
}
public void setMainMaterials(List mainMaterials) {
this.mainMaterials = mainMaterials;
}
/**
* 后台类目属性
*/
private List categoryProperties;
public List getCategoryProperties() {
return categoryProperties;
}
public void setCategoryProperties(List categoryProperties) {
this.categoryProperties = categoryProperties;
}
/**
* 主图联动方式
*/
private int imageLinkageType;
public int getImageLinkageType() {
return imageLinkageType;
}
public void setImageLinkageType(int imageLinkageType) {
this.imageLinkageType = imageLinkageType;
}
/**
* 商品副图片集合
*/
private List imageUrls;
public List getImageUrls() {
return imageUrls;
}
public void setImageUrls(List imageUrls) {
this.imageUrls = imageUrls;
}
/**
* 商品详情图片集合
*/
private List descImageUrls;
public List getDescImageUrls() {
return descImageUrls;
}
public void setDescImageUrls(List descImageUrls) {
this.descImageUrls = descImageUrls;
}
/**
* 商品视频信息
*/
private OVideo video;
public OVideo getVideo() {
return video;
}
public void setVideo(OVideo video) {
this.video = video;
}
/**
* 商品类型
*/
private ItemType itemType;
public ItemType getItemType() {
return itemType;
}
public void setItemType(ItemType itemType) {
this.itemType = itemType;
}
/**
* 商品配料列表(已废弃)
*/
private List subItemIds;
public List getSubItemIds() {
return subItemIds;
}
public void setSubItemIds(List subItemIds) {
this.subItemIds = subItemIds;
}
/**
* 商品套餐信息(已废弃)
*/
private List oPackages;
public List getOPackages() {
return oPackages;
}
public void setOPackages(List oPackages) {
this.oPackages = oPackages;
}
/**
* 商品配料组(已废弃)
*/
private IngredientGroup ingredientGroup;
public IngredientGroup getIngredientGroup() {
return ingredientGroup;
}
public void setIngredientGroup(IngredientGroup ingredientGroup) {
this.ingredientGroup = ingredientGroup;
}
/**
* 上下架状态
*/
private int onShelf;
public int getOnShelf() {
return onShelf;
}
public void setOnShelf(int onShelf) {
this.onShelf = onShelf;
}
/**
* 商品多层规格信息(已废弃)
*/
private List specProperties;
public List getSpecProperties() {
return specProperties;
}
public void setSpecProperties(List specProperties) {
this.specProperties = specProperties;
}
/**
* 配料组
*/
private List groupRelations;
public List getGroupRelations() {
return groupRelations;
}
public void setGroupRelations(List groupRelations) {
this.groupRelations = groupRelations;
}
/**
* 商品扩展信息
*/
private OItemFeature itemFeature;
public OItemFeature getItemFeature() {
return itemFeature;
}
public void setItemFeature(OItemFeature itemFeature) {
this.itemFeature = itemFeature;
}
/**
* 商品排序权重
*/
private Integer rankWeight;
public Integer getRankWeight() {
return rankWeight;
}
public void setRankWeight(Integer rankWeight) {
this.rankWeight = rankWeight;
}
/**
* 商品扩展code
*/
private String extCode;
public String getExtCode() {
return extCode;
}
public void setExtCode(String extCode) {
this.extCode = extCode;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy