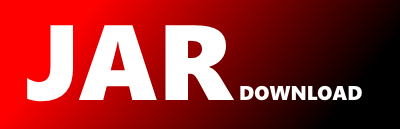
eleme.openapi.sdk.api.entity.product.OItemUpdateRequest Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.entity.product;
import eleme.openapi.sdk.api.enumeration.product.*;
import eleme.openapi.sdk.api.entity.product.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class OItemUpdateRequest{
/**
* 商品id
*/
private Long itemId;
public Long getItemId() {
return itemId;
}
public void setItemId(Long itemId) {
this.itemId = itemId;
}
/**
* 商品分组id
*/
private Long categoryId;
public Long getCategoryId() {
return categoryId;
}
public void setCategoryId(Long categoryId) {
this.categoryId = categoryId;
}
/**
* 商品名称
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* 商品描述
*/
private String description;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
* 图片imageHash
*/
private String imageHash;
public String getImageHash() {
return imageHash;
}
public void setImageHash(String imageHash) {
this.imageHash = imageHash;
}
/**
* 标签属性集合
*/
private OLabel labels;
public OLabel getLabels() {
return labels;
}
public void setLabels(OLabel labels) {
this.labels = labels;
}
/**
* 规格
*/
private List specs;
public List getSpecs() {
return specs;
}
public void setSpecs(List specs) {
this.specs = specs;
}
/**
* 售卖时间
*/
private OItemSellingTime sellingTime;
public OItemSellingTime getSellingTime() {
return sellingTime;
}
public void setSellingTime(OItemSellingTime sellingTime) {
this.sellingTime = sellingTime;
}
/**
* 属性
*/
private List attributes;
public List getAttributes() {
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
/**
* 商品最小起购量
*/
private Integer minPurchaseQuantity;
public Integer getMinPurchaseQuantity() {
return minPurchaseQuantity;
}
public void setMinPurchaseQuantity(Integer minPurchaseQuantity) {
this.minPurchaseQuantity = minPurchaseQuantity;
}
/**
* 商品单位
*/
private String unit;
public String getUnit() {
return unit;
}
public void setUnit(String unit) {
this.unit = unit;
}
/**
* 商品套餐标签
*/
private Integer setMeal;
public Integer getSetMeal() {
return setMeal;
}
public void setSetMeal(Integer setMeal) {
this.setMeal = setMeal;
}
/**
* 商品副图片imageHashs
*/
private List imageHashs;
public List getImageHashs() {
return imageHashs;
}
public void setImageHashs(List imageHashs) {
this.imageHashs = imageHashs;
}
/**
* 商品类型
*/
private ItemType itemType;
public ItemType getItemType() {
return itemType;
}
public void setItemType(ItemType itemType) {
this.itemType = itemType;
}
/**
* 主图联动方式方式
*/
private Integer imageLinkageType;
public Integer getImageLinkageType() {
return imageLinkageType;
}
public void setImageLinkageType(Integer imageLinkageType) {
this.imageLinkageType = imageLinkageType;
}
/**
* 商品规格描述
*/
private List specAttrs;
public List getSpecAttrs() {
return specAttrs;
}
public void setSpecAttrs(List specAttrs) {
this.specAttrs = specAttrs;
}
/**
* 商品详情图片的hash值
*/
private List descPhotoHashes;
public List getDescPhotoHashes() {
return descPhotoHashes;
}
public void setDescPhotoHashes(List descPhotoHashes) {
this.descPhotoHashes = descPhotoHashes;
}
/**
* 商品扩展
*/
private OItemFeature itemFeature;
public OItemFeature getItemFeature() {
return itemFeature;
}
public void setItemFeature(OItemFeature itemFeature) {
this.itemFeature = itemFeature;
}
/**
* 商品排序
*/
private Integer rankWeight;
public Integer getRankWeight() {
return rankWeight;
}
public void setRankWeight(Integer rankWeight) {
this.rankWeight = rankWeight;
}
/**
* 商品extend code
*/
private String extCode;
public String getExtCode() {
return extCode;
}
public void setExtCode(String extCode) {
this.extCode = extCode;
}
/**
* 配料分组
*/
private List ingredientGroups;
public List getIngredientGroups() {
return ingredientGroups;
}
public void setIngredientGroups(List ingredientGroups) {
this.ingredientGroups = ingredientGroups;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy