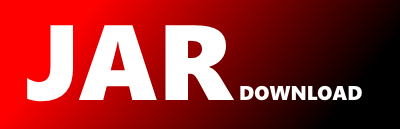
eleme.openapi.sdk.api.entity.product.OSpec Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.entity.product;
import eleme.openapi.sdk.api.enumeration.product.*;
import eleme.openapi.sdk.api.entity.product.*;
import java.util.*;
import java.math.BigDecimal;
import com.fasterxml.jackson.annotation.JsonFormat;
public class OSpec{
/**
* 规格Id
*/
private long specId;
public long getSpecId() {
return specId;
}
public void setSpecId(long specId) {
this.specId = specId;
}
/**
* 名称
*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* 商品价格
*/
private double price;
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
/**
* 价格类型
*/
private String priceType;
public String getPriceType() {
return priceType;
}
public void setPriceType(String priceType) {
this.priceType = priceType;
}
/**
* 套餐类型
*/
private String packageType;
public String getPackageType() {
return packageType;
}
public void setPackageType(String packageType) {
this.packageType = packageType;
}
/**
* 库存量
*/
private int stock;
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
/**
* 最大库存量
*/
private int maxStock;
public int getMaxStock() {
return maxStock;
}
public void setMaxStock(int maxStock) {
this.maxStock = maxStock;
}
/**
* 是否次日自动置满库存(此参数将替代并扩展无限库存的功能)
*/
private Integer stockStatus;
public Integer getStockStatus() {
return stockStatus;
}
public void setStockStatus(Integer stockStatus) {
this.stockStatus = stockStatus;
}
/**
* 打包费
*/
private double packingFee;
public double getPackingFee() {
return packingFee;
}
public void setPackingFee(double packingFee) {
this.packingFee = packingFee;
}
/**
* 是否上架,1:上架,0:下架,不填写默认0
*/
private int onShelf;
public int getOnShelf() {
return onShelf;
}
public void setOnShelf(int onShelf) {
this.onShelf = onShelf;
}
/**
* 商品扩展码
*/
private String extendCode;
public String getExtendCode() {
return extendCode;
}
public void setExtendCode(String extendCode) {
this.extendCode = extendCode;
}
/**
* 商品条形码
*/
private String barCode;
public String getBarCode() {
return barCode;
}
public void setBarCode(String barCode) {
this.barCode = barCode;
}
/**
* 商品重量,单位为克(已下线)
*/
private Integer weight;
public Integer getWeight() {
return weight;
}
public void setWeight(Integer weight) {
this.weight = weight;
}
/**
* 商品活动信息,1:有活动,0:无活动,活动商品不支持修改,如需修改请先取消活动,activityLevel字段只能查询,不能更新
*/
private int activityLevel;
public int getActivityLevel() {
return activityLevel;
}
public void setActivityLevel(int activityLevel) {
this.activityLevel = activityLevel;
}
/**
* 配送链路配置
*/
private OSupplyLink supplyLink;
public OSupplyLink getSupplyLink() {
return supplyLink;
}
public void setSupplyLink(OSupplyLink supplyLink) {
this.supplyLink = supplyLink;
}
/**
* 多层规格信息(已废弃)
*/
private List propertyList;
public List getPropertyList() {
return propertyList;
}
public void setPropertyList(List propertyList) {
this.propertyList = propertyList;
}
/**
* 总店商品pid
*/
private Long pid;
public Long getPid() {
return pid;
}
public void setPid(Long pid) {
this.pid = pid;
}
/**
* 规格卡路里
*/
private Long calorie;
public Long getCalorie() {
return calorie;
}
public void setCalorie(Long calorie) {
this.calorie = calorie;
}
/**
* 规格扩展信息
*/
private OSpecAttribute specAttribute;
public OSpecAttribute getSpecAttribute() {
return specAttribute;
}
public void setSpecAttribute(OSpecAttribute specAttribute) {
this.specAttribute = specAttribute;
}
/**
* 商品锁id
*/
private List lockIds;
public List getLockIds() {
return lockIds;
}
public void setLockIds(List lockIds) {
this.lockIds = lockIds;
}
/**
* 规格图片
*/
private String photoHash;
public String getPhotoHash() {
return photoHash;
}
public void setPhotoHash(String photoHash) {
this.photoHash = photoHash;
}
/**
* 规格选中图片
*/
private String selectPhotoHash;
public String getSelectPhotoHash() {
return selectPhotoHash;
}
public void setSelectPhotoHash(String selectPhotoHash) {
this.selectPhotoHash = selectPhotoHash;
}
/**
* 配料组
*/
private List groupRelations;
public List getGroupRelations() {
return groupRelations;
}
public void setGroupRelations(List groupRelations) {
this.groupRelations = groupRelations;
}
/**
* 规格维度商品属性,与商品维度互斥,不支持联动图片等
*/
private List attributes;
public List getAttributes() {
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
/**
* 互斥规格ID,为单向互斥
*/
private List mutexSpecIds;
public List getMutexSpecIds() {
return mutexSpecIds;
}
public void setMutexSpecIds(List mutexSpecIds) {
this.mutexSpecIds = mutexSpecIds;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy