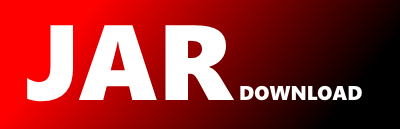
eleme.openapi.sdk.api.service.FinanceService Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.service;
import eleme.openapi.sdk.api.annotation.Service;
import eleme.openapi.sdk.api.base.BaseNopService;
import eleme.openapi.sdk.api.exception.ServiceException;
import eleme.openapi.sdk.oauth.response.Token;
import eleme.openapi.sdk.config.Config;
import eleme.openapi.sdk.api.entity.finance.*;
import eleme.openapi.sdk.api.enumeration.finance.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.*;
/**
* 金融服务
*/
@Service("eleme.finance")
public class FinanceService extends BaseNopService {
public FinanceService(Config config,Token token) {
super(config, token, FinanceService.class);
}
/**
* 查询商户余额 返回可用余额和子账户余额明细
*
* @param shopId 饿了么总店店铺id
* @return 商户余额与子账户明细
* @throws ServiceException 服务异常
*/
public BalanceResponse queryNewBalance(Long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.finance.queryNewBalance", params);
}
/**
* 查询余额流水,有流水改动的交易
*
* @param request 查询条件
* @return 余额流水
* @throws ServiceException 服务异常
*/
public OBalanceLogResponse queryBalanceLog(OQueryBalanceLogRequest request) throws ServiceException {
Map params = new HashMap();
params.put("request", request);
return call("eleme.finance.queryBalanceLog", params);
}
/**
* 查询总店账单(新接口)
*
* @param shopId 饿了么总店店铺id
* @param query 查询条件
* @return 总店账单
* @throws ServiceException 服务异常
*/
public BillsNew queryHeadBillsNew(Long shopId, HeadQuery query) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("query", query);
return call("eleme.finance.queryHeadBillsNew", params);
}
/**
* 查询总店订单(新接口)
*
* @param shopId 饿了么总店店铺id
* @param query 查询条件
* @return 总店金融订单
* @throws ServiceException 服务异常
*/
public FinanceOrdersNew queryHeadOrdersNew(Long shopId, HeadQuery query) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("query", query);
return call("eleme.finance.queryHeadOrdersNew", params);
}
/**
* 查询分店账单(新接口)
*
* @param shopId 饿了么分店店铺id
* @param query 查询条件
* @return 分店账单
* @throws ServiceException 服务异常
*/
public BillsNew queryBranchBillsNew(Long shopId, BranchQuery query) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("query", query);
return call("eleme.finance.queryBranchBillsNew", params);
}
/**
* 查询分店订单(新接口)
*
* @param shopId 饿了么分店店铺id
* @param query 查询条件
* @return 分店金融订单
* @throws ServiceException 服务异常
*/
public FinanceOrdersNew queryBranchOrdersNew(Long shopId, BranchQuery query) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("query", query);
return call("eleme.finance.queryBranchOrdersNew", params);
}
/**
* 查询订单(新接口)
*
* @param shopId 饿了么店铺id
* @param orderId 订单id
* @return 金融订单
* @throws ServiceException 服务异常
*/
public FinanceOrdersNew getOrderNew(Long shopId, String orderId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("orderId", orderId);
return call("eleme.finance.getOrderNew", params);
}
/**
* 查询返现汇总信息账单
*
* @param shopId 饿了么分店、单店、总店店铺id
* @param query 查询条件
* @return 分店账单
* @throws ServiceException 服务异常
*/
public AllowanceBills queryAllowanceBills(Long shopId, BranchQuery query) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("query", query);
return call("eleme.finance.queryAllowanceBills", params);
}
/**
* 查询返现每日详单
*
* @param shopId 饿了么分店、单店、总店店铺id
* @param query 查询条件
* @return 分店详单
* @throws ServiceException 服务异常
*/
public AllowanceBillDetails queryAllowanceBillDetail(Long shopId, HeadQuery query) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("query", query);
return call("eleme.finance.queryAllowanceBillDetail", params);
}
/**
* 查询商户帐期和名称
*
* @param termAndNameQuery 查询条件
* @return 账户账期、名称、及结算方式
* @throws ServiceException 服务异常
*/
public TermAndNameResult queryTermAndName(TermAndNameQuery termAndNameQuery) throws ServiceException {
Map params = new HashMap();
params.put("termAndNameQuery", termAndNameQuery);
return call("eleme.finance.queryTermAndName", params);
}
/**
* 子资金账号查询关系
*
* @param relationsRequest 查询条件
* @return 子资金账号关系
* @throws ServiceException 服务异常
*/
public List queryBySlave(FundRelationsRequest relationsRequest) throws ServiceException {
Map params = new HashMap();
params.put("relationsRequest", relationsRequest);
return call("eleme.finance.queryBySlave", params);
}
/**
* 查询连锁总店结算子门店关系列表
*
* @param chainId 饿了么连锁店店铺id
* @param checkoutDate 入账日期
* @return 子门店id列表
* @throws ServiceException 服务异常
*/
public List querySlaveShopIdsByChainId(Long chainId, Date checkoutDate) throws ServiceException {
Map params = new HashMap();
params.put("chainId", chainId);
params.put("checkoutDate", checkoutDate);
return call("eleme.finance.querySlaveShopIdsByChainId", params);
}
/**
* 批量查询分店商品维度的账单数据
*
* @param settleAccountShopId 结算入账ID
* @param shopIdList 门店id列表(限制100)
* @param query 查询条件
* @return 商品明细订单
* @throws ServiceException 服务异常
*/
public FinanceGoodsOrders queryGoodsOrders(Long settleAccountShopId, List shopIdList, BranchQuery query) throws ServiceException {
Map params = new HashMap();
params.put("settleAccountShopId", settleAccountShopId);
params.put("shopIdList", shopIdList);
params.put("query", query);
return call("eleme.finance.queryGoodsOrders", params);
}
/**
* 分页查询总店通兑卡账单
*
* @param pageQuery 总店账单分页查询条件
* @return 通兑卡账单信息
* @throws ServiceException 服务异常
*/
public GenericCardBillsResponse queryHeadShopGenericCardBills(HeadShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.queryHeadShopGenericCardBills", params);
}
/**
* 分页查询分店通兑卡账单列表
*
* @param pageQuery 分页查询条件
* @return 通兑卡账单信息
* @throws ServiceException 服务异常
*/
public GenericCardBillsResponse queryBranchShopGenericCardBills(BranchShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.queryBranchShopGenericCardBills", params);
}
/**
* 查询外卖订单通兑卡账单信息
*
* @param orderBillQuery 外卖订单查询条件
* @return 通兑卡账单信息
* @throws ServiceException 服务异常
*/
public GenericCardBillsResponse queryGenericCardBillByOrder(OrderBillQuery orderBillQuery) throws ServiceException {
Map params = new HashMap();
params.put("orderBillQuery", orderBillQuery);
return call("eleme.finance.queryGenericCardBillByOrder", params);
}
/**
* 分页查询店铺返佣账单信息
*
* @param pageQuery 分页查询返佣账单条件
* @return 返佣账单查询结果
* @throws ServiceException 服务异常
*/
public RewardBillResult queryChainShopRewardBill(RewardBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.queryChainShopRewardBill", params);
}
/**
* 查询外卖订单的返佣账单信息
*
* @param query 分页查询返佣账单条件
* @return 返佣账单查询结果
* @throws ServiceException 服务异常
*/
public RewardBillResult queryRewardBillByOrder(RewardBillQuery query) throws ServiceException {
Map params = new HashMap();
params.put("query", query);
return call("eleme.finance.queryRewardBillByOrder", params);
}
/**
* 分页查询总店推广账单
*
* @param pageQuery 总店账单分页查询条件
* @return 推广账单信息
* @throws ServiceException 服务异常
*/
public PromotionBillResponse pageQueryChainShopPromotionBill(HeadShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.pageQueryChainShopPromotionBill", params);
}
/**
* 分页查询单店推广账单
*
* @param branchShopBillPageQuery 分页账单查询条件
* @return 推广账单信息
* @throws ServiceException 服务异常
*/
public PromotionBillResponse pageQueryBranchShopPromotionBill(BranchShopBillPageQuery branchShopBillPageQuery) throws ServiceException {
Map params = new HashMap();
params.put("branchShopBillPageQuery", branchShopBillPageQuery);
return call("eleme.finance.pageQueryBranchShopPromotionBill", params);
}
/**
* 查询外卖订单推广账单
*
* @param orderBillQuery 外卖订单查询条件
* @return 推广账单信息
* @throws ServiceException 服务异常
*/
public PromotionBillResponse queryPromotionBillsByOrder(OrderBillQuery orderBillQuery) throws ServiceException {
Map params = new HashMap();
params.put("orderBillQuery", orderBillQuery);
return call("eleme.finance.queryPromotionBillsByOrder", params);
}
/**
* 分页查询总店保险账单
*
* @param pageQuery 总店账单分页查询条件
* @return 保险账单信息
* @throws ServiceException 服务异常
*/
public InsuranceBillResponse pageQueryChainShopInsuranceBills(HeadShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.pageQueryChainShopInsuranceBills", params);
}
/**
* 分页查询单店保险账单
*
* @param pageQuery 分页查询条件
* @return 保险账单信息
* @throws ServiceException 服务异常
*/
public InsuranceBillResponse pageQueryBranchShopInsuranceBills(BranchShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.pageQueryBranchShopInsuranceBills", params);
}
/**
* 查询外卖订单保险账单
*
* @param orderBillQuery 外卖订单查询条件
* @return 保险账单信息
* @throws ServiceException 服务异常
*/
public InsuranceBillResponse queryInsuranceBillsByOrder(OrderBillQuery orderBillQuery) throws ServiceException {
Map params = new HashMap();
params.put("orderBillQuery", orderBillQuery);
return call("eleme.finance.queryInsuranceBillsByOrder", params);
}
/**
* 分页查询总店代运营账单
*
* @param pageQuery 总店账单分页查询条件
* @return 代运营账单信息
* @throws ServiceException 服务异常
*/
public AgentCommissionBillResponse pageQueryChainShopAgentCommissionBills(HeadShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.pageQueryChainShopAgentCommissionBills", params);
}
/**
* 分页查询单店代运营账单
*
* @param pageQuery 分页查询条件
* @return 代运营账单信息
* @throws ServiceException 服务异常
*/
public AgentCommissionBillResponse pageQueryBranchShopAgentCommissionBills(BranchShopBillPageQuery pageQuery) throws ServiceException {
Map params = new HashMap();
params.put("pageQuery", pageQuery);
return call("eleme.finance.pageQueryBranchShopAgentCommissionBills", params);
}
/**
* 查询外卖订单代运营账单
根据订单ID查询
一笔订单可能有多个代运营账单,存在正常单和退单
*
* @param orderBillQuery 外卖订单查询条件
* @return 代运营账单信息
* @throws ServiceException 服务异常
*/
public AgentCommissionBillResponse queryAgentCommissionBillsByOrder(OrderBillQuery orderBillQuery) throws ServiceException {
Map params = new HashMap();
params.put("orderBillQuery", orderBillQuery);
return call("eleme.finance.queryAgentCommissionBillsByOrder", params);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy