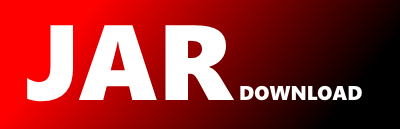
eleme.openapi.sdk.api.service.ShopService Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.api.service;
import eleme.openapi.sdk.api.annotation.Service;
import eleme.openapi.sdk.api.base.BaseNopService;
import eleme.openapi.sdk.api.exception.ServiceException;
import eleme.openapi.sdk.oauth.response.Token;
import eleme.openapi.sdk.config.Config;
import eleme.openapi.sdk.api.entity.shop.*;
import eleme.openapi.sdk.api.enumeration.shop.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.*;
/**
* 店铺服务
*/
@Service("eleme.shop")
public class ShopService extends BaseNopService {
public ShopService(Config config,Token token) {
super(config, token, ShopService.class);
}
/**
* 查询店铺信息
*
* @param shopId 店铺Id
* @return 店铺
* @throws ServiceException 服务异常
*/
public OShop getShop(long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.getShop", params);
}
/**
* 抖音回调接口
*
* @param ele_dto 回调对象
* @return 状态
* @throws ServiceException 服务异常
*/
public boolean dyCallBackForEle(DyCallBackEleDto ele_dto) throws ServiceException {
Map params = new HashMap();
params.put("ele_dto", ele_dto);
return call("eleme.shop.dyCallBackForEle", params);
}
/**
* 抖音解绑回调接口
*
* @param accountId 抖音总户ID
* @param merchantId 抖音门店户ID
* @param version 版本号
* @return 状态
* @throws ServiceException 服务异常
*/
public boolean dyUnBindShopForEle(String accountId, String merchantId, String version) throws ServiceException {
Map params = new HashMap();
params.put("accountId", accountId);
params.put("merchantId", merchantId);
params.put("version", version);
return call("eleme.shop.dyUnBindShopForEle", params);
}
/**
* 更新店铺信息
*
* @param shopId 店铺Id
* @param properties 店铺属性
* @return 店铺
* @throws ServiceException 服务异常
*/
public OShop updateShop(long shopId, Map properties) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("properties", properties);
return call("eleme.shop.updateShop", params);
}
/**
* 更新店铺基本信息
*
* @param shopId 店铺Id
* @param properties 店铺基本属性
* @return 是否成功
* @throws ServiceException 服务异常
*/
public boolean updateShopBasicInfo(long shopId, Map properties) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("properties", properties);
return call("eleme.shop.updateShopBasicInfo", params);
}
/**
* 批量获取店铺简要
*
* @param shopIds 店铺Id的列表
* @return 店铺简要列表
* @throws ServiceException 服务异常
*/
public Map mgetShopStatus(List shopIds) throws ServiceException {
Map params = new HashMap();
params.put("shopIds", shopIds);
return call("eleme.shop.mgetShopStatus", params);
}
/**
* 设置送达时间
*
* @param shopId 店铺Id
* @param deliveryBasicMins 配送基准时间(单位分钟)
* @param deliveryAdjustMins 配送调整时间(单位分钟)
* @param specialDeliveryTime 特殊时段配送时间(单位分钟)
* @param distancePlusTime 3km以上每km增加的时间(单位分钟)
* @throws ServiceException 服务异常
*/
public void setDeliveryTime(long shopId, int deliveryBasicMins, int deliveryAdjustMins, List specialDeliveryTime, int distancePlusTime) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("deliveryBasicMins", deliveryBasicMins);
params.put("deliveryAdjustMins", deliveryAdjustMins);
params.put("specialDeliveryTime", specialDeliveryTime);
params.put("distancePlusTime", distancePlusTime);
call("eleme.shop.setDeliveryTime", params);
}
/**
* 自配送商家设置送达时间
*
* @param shopId 店铺Id
* @param deliveryTime 配送总时间(单位:分钟)
* @param specialDeliveryTime 特殊时段配送时间(单位分钟)
* @param distancePlusTime 3km以上每km增加的时间(单位分钟)
* @throws ServiceException 服务异常
*/
public void setSelfShopDeliveryTime(long shopId, Integer deliveryTime, List specialDeliveryTime, int distancePlusTime) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("deliveryTime", deliveryTime);
params.put("specialDeliveryTime", specialDeliveryTime);
params.put("distancePlusTime", distancePlusTime);
call("eleme.shop.setSelfShopDeliveryTime", params);
}
/**
* 查询自配送推荐送达时间
*
* @param shopId 店铺Id
* @return 推荐送达时间
* @throws ServiceException 服务异常
*/
public ShopRecommendDeliveryTimeDto getRecommendDeliveryTime(long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.getRecommendDeliveryTime", params);
}
/**
* 设置是否支持在线退单
*
* @param shopId 店铺Id
* @param enable 是否支持
* @throws ServiceException 服务异常
*/
public void setOnlineRefund(long shopId, boolean enable) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("enable", enable);
call("eleme.shop.setOnlineRefund", params);
}
/**
* 设置是否支持预定单及预定天数
*
* @param shopId 店铺id
* @param enabled 是否支持预订
* @param maxBookingDays 最大预定天数
* @throws ServiceException 服务异常
*/
public void setBookingStatus(long shopId, boolean enabled, Integer maxBookingDays) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("enabled", enabled);
params.put("maxBookingDays", maxBookingDays);
call("eleme.shop.setBookingStatus", params);
}
/**
* 批量通过店铺Id获取Oid
*
* @param shopIds 店铺Id的列表
* @return 店铺id,oid映射列表
* @throws ServiceException 服务异常
*/
public Map getOidByShopIds(List shopIds) throws ServiceException {
Map params = new HashMap();
params.put("shopIds", shopIds);
return call("eleme.shop.getOidByShopIds", params);
}
/**
* 更新店铺营业时间预设置
*
* @param shopId 店铺id
* @param weekSetting 一周营业时间预设置, 参考 OShopBusyLevelSetting weekSetting 字段定义
* @param dateSetting 特定日期营业时间预设置, 参考 OShopBusyLevelSetting dateSetting 字段定义
* @throws ServiceException 服务异常
*/
public void updateBusyLevelSetting(long shopId, Map weekSetting, Map dateSetting) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("weekSetting", weekSetting);
params.put("dateSetting", dateSetting);
call("eleme.shop.updateBusyLevelSetting", params);
}
/**
* 获取店铺营业时间预设置
*
* @param shopId 店铺id
* @return 店铺营业时间预设置
* @throws ServiceException 服务异常
*/
public OShopBusyLevelSetting getBusyLevelSetting(long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.getBusyLevelSetting", params);
}
/**
* 设置品牌排序权重
*
* @param shopId 店铺Id
* @param weight 权重值(取值范围[0~10])
* @throws ServiceException 服务异常
*/
public void setBrandRankWeight(long shopId, double weight) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("weight", weight);
call("eleme.shop.setBrandRankWeight", params);
}
/**
* 获取店铺可补贴配送费的标品及补贴上限
*
* @param shopId 店铺Id
* @return 店铺可补贴配送费的标品及补贴上限集合
* @throws ServiceException 服务异常
*/
public List getProductSubsidyLimit(long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.getProductSubsidyLimit", params);
}
/**
* 设置店铺T模型
*
* @param shopId 店铺Id
* @param deliveryTime 配送总时间(单位:分钟)
* @param specialDeliveryTime 特殊时段配送时间(单位分钟)
* @param distancePlusTime 3km以上每km增加的时间(单位分钟)
* @throws ServiceException 服务异常
*/
public void setShopTModel(long shopId, Integer deliveryTime, List specialDeliveryTime, int distancePlusTime) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("deliveryTime", deliveryTime);
params.put("specialDeliveryTime", specialDeliveryTime);
params.put("distancePlusTime", distancePlusTime);
call("eleme.shop.setShopTModel", params);
}
/**
* 设置店铺假期歇业
*
* @param shopId 店铺Id
* @param vocationDates 店铺休假日期
* @param enabled 店铺休假是否有效
* @throws ServiceException 服务异常
*/
public void setShopVocations(long shopId, List vocationDates, Boolean enabled) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("vocationDates", vocationDates);
params.put("enabled", enabled);
call("eleme.shop.setShopVocations", params);
}
/**
* 获取店铺有效的假期歇业日期
*
* @param shopId 店铺Id
* @return 返回店铺有效的假期歇业日期list |["2018-11-05:2018-11-06","2018-11-09:2018-11-11"]
* @throws ServiceException 服务异常
*/
public List getShopVocation(long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.getShopVocation", params);
}
/**
* 查询店铺主体资质信息
*
* @param shopId 店铺id
* @return 返回店铺的主体资质信息
* @throws ServiceException 服务异常
*/
public ShopLicenseInfo getShopLicense(long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.getShopLicense", params);
}
/**
* 查询长链地址
*
* @param queryLongLineAddressDTO 查询长链地址表单
* @return 长链地址
* @throws ServiceException 服务异常
*/
public String getWssAddress(QueryLongLineAddressDTO queryLongLineAddressDTO) throws ServiceException {
Map params = new HashMap();
params.put("queryLongLineAddressDTO", queryLongLineAddressDTO);
return call("eleme.shop.im.getWssAddress", params);
}
/**
* 查询店铺IM状态
*
* @param shopId 店铺ID
* @return IM开关状态(0:关闭 1:开启)| TRUE/FALSE/NULL
* @throws ServiceException 服务异常
*/
public Boolean getIMStatus(Long shopId) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
return call("eleme.shop.im.getIMStatus", params);
}
/**
* 更新店铺IM开关状态
*
* @param shopId 店铺ID
* @param status IM开关状态(0:关闭 1:开启)
* @return 处理结果
* @throws ServiceException 服务异常
*/
public Boolean updateIMStatus(Long shopId, Integer status) throws ServiceException {
Map params = new HashMap();
params.put("shopId", shopId);
params.put("status", status);
return call("eleme.shop.im.updateIMStatus", params);
}
/**
* 提交开店申请接口
*
* @param openStoreMessage 开店申请表单
* @return 请求提交id
* @throws ServiceException 服务异常
*/
public Long submitOpenStoreMessageAudit(OpenStoreMessageBody openStoreMessage) throws ServiceException {
Map params = new HashMap();
params.put("openStoreMessage", openStoreMessage);
return call("eleme.shop.setup.submitOpenStoreMessageAudit", params);
}
/**
* 星巴克提交开店申请接口
*
* @param openStoreMessage 开店申请表单
* @return 请求提交id
* @throws ServiceException 服务异常
*/
public Long submitOpenStoreForMermaid(OpenStoreMessageBody openStoreMessage) throws ServiceException {
Map params = new HashMap();
params.put("openStoreMessage", openStoreMessage);
return call("eleme.shop.setup.submitOpenStoreForMermaid", params);
}
/**
* 更新申请信息接口
*
* @param updateStoreMessageBody 开店申请表单
* @throws ServiceException 服务异常
*/
public void updateOpenStoreMessageAudit(UpdateStoreMessageBody updateStoreMessageBody) throws ServiceException {
Map params = new HashMap();
params.put("updateStoreMessageBody", updateStoreMessageBody);
call("eleme.shop.setup.updateOpenStoreMessageAudit", params);
}
/**
* 查询请求状态接口
*
* @param submitId 请求提交id
* @return 流程状态返回体
* @throws ServiceException 服务异常
*/
public ProcessStatusResult queryProcessStatusBySubmitId(Long submitId) throws ServiceException {
Map params = new HashMap();
params.put("submitId", submitId);
return call("eleme.shop.setup.queryProcessStatusBySubmitId", params);
}
/**
* 图片上传处理接口(5M以内图片)
*
* @param imageBase64 base64字节流
* @return 图片处理后的hash值
* @throws ServiceException 服务异常
*/
public String uploadImage(String imageBase64) throws ServiceException {
Map params = new HashMap();
params.put("imageBase64", imageBase64);
return call("eleme.shop.setup.uploadImage", params);
}
/**
* 图片上传处理接口(500K以内图片)
*
* @param imageBase64 base64字节流
* @return 图片处理后的hash值
* @throws ServiceException 服务异常
*/
public String uploadMinImage(String imageBase64) throws ServiceException {
Map params = new HashMap();
params.put("imageBase64", imageBase64);
return call("eleme.shop.setup.uploadMinImage", params);
}
/**
* 远程上传图片接口
*
* @param url 图片url
* @return 图片处理后的hash值
* @throws ServiceException 服务异常
*/
public String uploadImageWithRemoteUrl(String url) throws ServiceException {
Map params = new HashMap();
params.put("url", url);
return call("eleme.shop.setup.uploadImageWithRemoteUrl", params);
}
/**
* 第三方服务商传递摄像头状态变更消息
*
* @param videoDeviceDTO 设备状态信息
* @return 成功接收返回true,请求失败返回false
* @throws ServiceException 服务异常
*/
public Boolean setVideoStatus(OVideoDeviceDTO videoDeviceDTO) throws ServiceException {
Map params = new HashMap();
params.put("videoDeviceDTO", videoDeviceDTO);
return call("eleme.shop.video.setVideoStatus", params);
}
/**
* (仅电信用,现已废弃)根据统一社会信用代码查询在饿了么侧是否已开店
*
* @param licenseNo 统一社会信用代码
* @return 开店返回true,未开店返回false
* @throws ServiceException 服务异常
*/
public Boolean validateShopVideoStatus(String licenseNo) throws ServiceException {
Map params = new HashMap();
params.put("licenseNo", licenseNo);
return call("eleme.shop.video.validateShopVideoStatus", params);
}
/**
* 根据统一社会信用代码查询在饿了么侧是否已开店
*
* @param licenseCheckDTO 设备商及统一社会信用代码
* @return 开店返回true,未开店返回false
* @throws ServiceException 服务异常
*/
public Boolean validateShopVideoStatusNew(LicenseCheckDTO licenseCheckDTO) throws ServiceException {
Map params = new HashMap();
params.put("licenseCheckDTO", licenseCheckDTO);
return call("eleme.shop.video.validateShopVideoStatusNew", params);
}
/**
* 第三方服务商同步设备信息
*
* @param licenseDeviceInfo 设备信息
* @return 成功接收返回true,请求失败返回false
* @throws ServiceException 服务异常
*/
public Boolean syncShopVideoDevices(LicenseDeviceInfo licenseDeviceInfo) throws ServiceException {
Map params = new HashMap();
params.put("licenseDeviceInfo", licenseDeviceInfo);
return call("eleme.shop.video.syncShopVideoDevices", params);
}
/**
* 第三方服务商同步AI识别结果
*
* @param shopAiRecognition AI识别结果
* @return 成功接收返回true,请求失败返回false
* @throws ServiceException 服务异常
*/
public BizResponse syncShopAiRecognitionResult(List shopAiRecognition) throws ServiceException {
Map params = new HashMap();
params.put("shopAiRecognition", shopAiRecognition);
return call("eleme.shop.video.syncShopAiRecognitionResult", params);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy