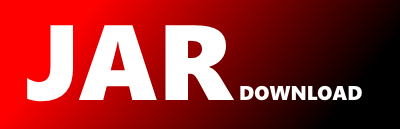
eleme.openapi.sdk.media.trace.DeviceId Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.media.trace;
import eleme.openapi.sdk.media.common.EncodeUtil;
import eleme.openapi.sdk.media.utils.IOUtils;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.UnsupportedEncodingException;
import java.util.UUID;
/**
* Created by huamulou on 15/12/9.
*/
public class DeviceId {
private String savePath;
private String deviceId;
public DeviceId(String savePath) {
this.savePath = savePath;
}
public String getValue() {
if (deviceId != null) return deviceId;
synchronized (this) {
if (deviceId != null) return deviceId;
deviceId = readFromFile();
if (deviceId != null) return deviceId;
deviceId = getUUID();
writeToFile(deviceId);
return deviceId;
}
}
public byte[] asBytes(String id) {
UUID uuid = UUID.fromString(id);
long msb = uuid.getMostSignificantBits();
long lsb = uuid.getLeastSignificantBits();
byte[] buffer = new byte[16];
for (int i = 0; i < 8; i++) {
buffer[i] = (byte) (msb >>> 8 * (7 - i));
}
for (int i = 8; i < 16; i++) {
buffer[i] = (byte) (lsb >>> 8 * (7 - i));
}
return buffer;
}
private String getUUID() {
return ShorterUUID.uuid();
}
private String shorter(String arg) {
byte[] res = EncodeUtil.encodeWithBase64(asBytes(arg));
try {
return new String(res, 0, res.length - 2, "utf-8");
} catch (UnsupportedEncodingException e) {
//
return null;
}
}
private String readFromFile() {
File file = new File(savePath);
if (file.exists()) {
InputStream is = null;
try {
is = new FileInputStream(file);
return IOUtils.toString(is);
} catch (Throwable e) {
//
} finally {
IOUtils.closeQuietly(is);
}
}
return null;
}
private String writeToFile(String arg) {
File file = new File(savePath);
OutputStream out = null;
try {
out = new FileOutputStream(file);
OutputStreamWriter writer = new OutputStreamWriter(out, "utf-8");
writer.write(arg);
writer.flush();
out.flush();
} catch (Throwable e) {
//
} finally {
IOUtils.closeQuietly(out);
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy