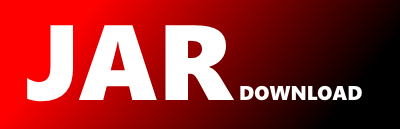
eleme.openapi.sdk.media.upload.UploadResponse Maven / Gradle / Ivy
The newest version!
package eleme.openapi.sdk.media.upload;
import java.io.Serializable;
import java.util.Date;
/**
* upload response
*
* @author jinli Feb 5, 2015
*/
public class UploadResponse implements Serializable {
private static final long serialVersionUID = 1L;
private String dir; // media file dir
private String name; // media file name
private String eTag; // media file md5
private long fileSize; // media file size
private String mimeType; // media file mime type
private Date fileModified; // media file last modified time
private String returnBody; // return body, rendered by magic vars and custom vars
private String customBody; // custom callback return body, rendered by magic vars and custom vars
private String uri; // media file uri
private boolean isImage; // is image file
private String url; // media file url
private String fileId; // media file id
private Long videoId;
public String getDir() {
return dir;
}
public void setDir(String dir) {
this.dir = dir;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String geteTag() {
return eTag;
}
public void seteTag(String eTag) {
this.eTag = eTag;
}
public long getFileSize() {
return fileSize;
}
public void setFileSize(long fileSize) {
this.fileSize = fileSize;
}
public String getMimeType() {
return mimeType;
}
public void setMimeType(String mimeType) {
this.mimeType = mimeType;
}
public Date getFileModified() {
return fileModified;
}
public void setFileModified(Date fileModified) {
this.fileModified = fileModified;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getReturnBody() {
return returnBody;
}
public void setReturnBody(String returnBody) {
this.returnBody = returnBody;
}
public String getCustomBody() {
return customBody;
}
public void setCustomBody(String customBody) {
this.customBody = customBody;
}
public String getUri() {
return uri;
}
public void setUri(String uri) {
this.uri = uri;
}
public boolean getIsImage() {
return isImage;
}
public void setIsImage(boolean isImage) {
this.isImage = isImage;
}
public String getFileId() {
return fileId;
}
public void setFileId(String fileId) {
this.fileId = fileId;
}
public Long getVideoId() {
return videoId;
}
public void setVideoId(Long videoId) {
this.videoId = videoId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy