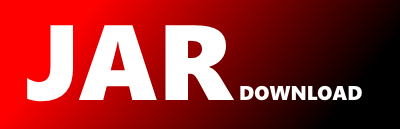
me.hsgamer.hscore.ui.BaseHolder Maven / Gradle / Ivy
package me.hsgamer.hscore.ui;
import org.jetbrains.annotations.NotNull;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Consumer;
/**
* A simple implementation of {@link Holder}
*
* @param The type of {@link Display}
*/
public abstract class BaseHolder implements Holder {
protected final Map displayMap = new ConcurrentHashMap<>();
private final Map, List>> classListMap = new HashMap<>();
/**
* Make a new display
*
* @param uuid the unique id
*
* @return the display
*/
@NotNull
protected abstract D newDisplay(UUID uuid);
/**
* Called when the display is removed
*
* @param display the display
*/
protected void onRemoveDisplay(@NotNull D display) {
// EMPTY
}
@Override
@NotNull
public D createDisplay(@NotNull UUID uuid) {
return displayMap.computeIfAbsent(uuid, uuid1 -> {
D display = newDisplay(uuid1);
display.init();
return display;
});
}
@Override
public void removeDisplay(@NotNull UUID uuid) {
Optional.ofNullable(displayMap.remove(uuid)).ifPresent(display -> {
onRemoveDisplay(display);
display.stop();
});
}
@Override
public void removeAllDisplay() {
displayMap.values().forEach(display -> {
onRemoveDisplay(display);
display.stop();
});
displayMap.clear();
}
@Override
public Optional<@NotNull D> getDisplay(@NotNull UUID uuid) {
return Optional.ofNullable(displayMap.get(uuid));
}
@Override
public void update() {
displayMap.values().forEach(D::update);
}
@Override
public void addEventConsumer(@NotNull Class eventClass, @NotNull Consumer eventConsumer) {
classListMap
.computeIfAbsent(eventClass, clazz -> new ArrayList<>())
.add(o -> eventConsumer.accept(eventClass.cast(o)));
}
@Override
public void stop() {
clearAllEventConsumer();
removeAllDisplay();
}
@Override
public void clearEventConsumer(@NotNull Class> eventClass) {
classListMap.remove(eventClass);
}
@Override
public void clearAllEventConsumer() {
classListMap.clear();
}
@Override
public void handleEvent(@NotNull Class> eventClass, @NotNull Object event) {
if (!classListMap.containsKey(eventClass)) return;
if (!eventClass.isInstance(event)) {
throw new IllegalArgumentException("The event is not an instance of " + eventClass.getName());
}
classListMap.get(eventClass).forEach(consumer -> consumer.accept(event));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy