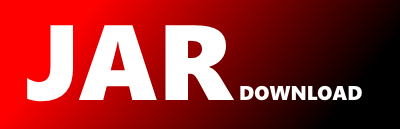
me.jakejmattson.kutils.internal.utils.ReflectionUtils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of KUtils Show documentation
Show all versions of KUtils Show documentation
A Discord bot framework for Kotlin.
package me.jakejmattson.kutils.internal.utils
import com.google.common.eventbus.Subscribe
import me.jakejmattson.kutils.api.annotations.*
import me.jakejmattson.kutils.api.dsl.command.*
import me.jakejmattson.kutils.api.dsl.preconditions.*
import me.jakejmattson.kutils.api.extensions.stdlib.pluralize
import org.reflections.Reflections
import org.reflections.scanners.MethodAnnotationsScanner
import java.lang.reflect.Method
internal object ReflectionUtils {
fun detectCommands(path: String): CommandsContainer {
val commandSets = detectMethodsWith(path)
.map { diService.invokeReturningMethod(it) to it.getAnnotation().category }
if (commandSets.isEmpty()) {
InternalLogger.startup("0 CommandSets -> 0 Commands")
return CommandsContainer()
}
val allCommands = commandSets
.flatMap { (container, cmdSetCategory) ->
container.commands.apply {
forEach { it.category = it.category.ifBlank { cmdSetCategory } }
}
}.toMutableList()
InternalLogger.startup(commandSets.size.pluralize("CommandSet") + " -> " + allCommands.size.pluralize("Command"))
return CommandsContainer(allCommands)
}
fun detectListeners(path: String) = detectMethodsWith(path)
.map { it.declaringClass }
.distinct()
.map { diService.invokeConstructor(it) }
fun detectPreconditions(path: String) = detectMethodsWith(path)
.map {
val annotation = it.getAnnotation()
val condition = diService.invokeReturningMethod<(CommandEvent<*>) -> PreconditionResult>(it)
PreconditionData(condition, annotation.priority)
}
inline fun detectClassesWith(path: String) = Reflections(path).getTypesAnnotatedWith(T::class.java)
inline fun detectSubtypesOf(path: String) = Reflections(path).getSubTypesOf(T::class.java)
inline fun detectMethodsWith(path: String) = Reflections(path, MethodAnnotationsScanner()).getMethodsAnnotatedWith(T::class.java)
}
internal inline fun Method.getAnnotation() = getAnnotation(T::class.java)
internal inline fun Class<*>.getAnnotation() = getAnnotation(T::class.java)
internal val Class<*>.simplerName
get() = simpleName.substringAfterLast(".")
© 2015 - 2024 Weber Informatics LLC | Privacy Policy