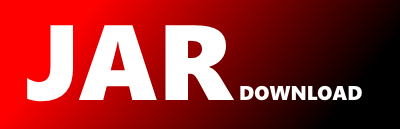
me.legrange.console.Head Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of console-animation Show documentation
Show all versions of console-animation Show documentation
A small console animation library
The newest version!
package me.legrange.console;
import java.util.LinkedList;
import java.util.List;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;
final class Head implements Animation {
private final List> animations = new LinkedList<>();
private final N min;
private final N max;
private N value;
private final Thread timer;
private boolean running;
Head(N min, N max) {
this.min = min;
this.max = max;
this.value = min;
timer = Thread.startVirtualThread(this::onTick);
}
@Override
public Animation progressBar(BarStyle style, int size) {
animations.add(new ProgressBar<>(size, style));
return this;
}
@Override
public Animation percent() {
animations.add(new Percent<>());
return this;
}
@Override
public Animation spinner(SpinnerStyle style) {
animations.add(new Spinner<>(style));
return this;
}
@Override
public Animation text(String text) {
animations.add(new Text<>(text));
return this;
}
@Override
public Animation counter() {
animations.add(new Counter<>());
return this;
}
@Override
public void update(N value) {
this.value = value;
timer.interrupt();
}
@Override
public void close() {
running = false;
timer.interrupt();
}
private void onTick() {
running = true;
while (running) {
try {
TimeUnit.MILLISECONDS.sleep(500);
} catch (InterruptedException ignore) {
}
if (running) {
refresh();
}
}
}
private void refresh() {
System.out.print("\r" + animations.stream()
.map(animator -> animator.draw(min, max, value))
.collect(Collectors.joining()));
System.out.flush();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy