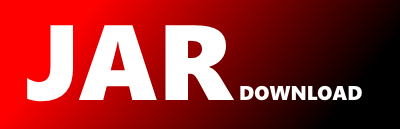
me.lightspeed7.mongofs.MongoFileCursor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoFS Show documentation
Show all versions of mongoFS Show documentation
An extension to the MongoDB Java Driver library that goes beyond what the GridFS feature supports.
Compressed file storage, zip files, temporary files
package me.lightspeed7.mongofs;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.mongodb.Document;
import org.mongodb.MongoCursor;
import org.mongodb.ServerCursor;
import org.mongodb.connection.ServerAddress;
/**
* This class is a surrogate holder for the actual MongoCursor object underneath. It store the
*
* @author David Buschman
*
*/
public class MongoFileCursor implements MongoCursor, Iterator, Iterable {
private final MongoCursor cursor;
private final MongoFileStore store;
/* package */MongoFileCursor(final MongoFileStore store, final MongoCursor cursor) {
this.store = store;
this.cursor = cursor;
}
@Override
public Iterator iterator() {
return this;
}
@Override
public MongoFile next() {
if (cursor.hasNext()) {
return new MongoFile(store, cursor.next());
}
return null;
}
@Override
public boolean hasNext() {
return cursor.hasNext();
}
@Override
public void remove() {
throw new UnsupportedOperationException("remove");
}
@Override
public void close() {
cursor.close();
}
@Override
public ServerAddress getServerAddress() {
return cursor.getServerAddress();
}
@Override
public ServerCursor getServerCursor() {
return cursor.getServerCursor();
}
/**
* This method will iterate the cursor a return all of the object from the cursor. Be sure you know the size of your result set.
*
* @return a List objects
*/
public List toList() {
List files = new ArrayList();
try {
while (cursor.hasNext()) {
files.add(new MongoFile(store, cursor.next()));
}
} finally {
if (cursor != null) {
cursor.close();
}
}
return files;
}
//
// toString
// /////////////////////////
@Override
public String toString() {
return String.format("MongoFileCursor [ store=%s, %n cursor=%s%n]", store, cursor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy