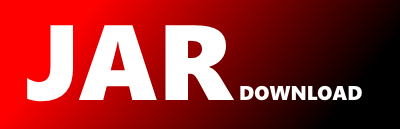
org.mongodb.CollectionAdministration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoFS Show documentation
Show all versions of mongoFS Show documentation
An extension to the MongoDB Java Driver library that goes beyond what the GridFS feature supports.
Compressed file storage, zip files, temporary files
package org.mongodb;
import java.util.ArrayList;
import java.util.List;
import com.mongodb.BasicDBObject;
import com.mongodb.DBCollection;
import com.mongodb.DBObject;
public class CollectionAdministration {
private DBCollection surrogate;
public CollectionAdministration(final DBCollection surrogate) {
this.surrogate = surrogate;
}
public List getIndexes() {
List indexes = new ArrayList();
for (DBObject dbObject : surrogate.getIndexInfo()) {
indexes.add(new Document(dbObject));
}
return indexes;
}
public void createIndexes(final List indexes) {
for (Index index : indexes) {
// keys
BasicDBObject keys = new BasicDBObject();
Document document = index.getKeys();
for (String key : document.keySet()) {
keys.put(key, document.get(key));
}
// options
BasicDBObject options = new BasicDBObject();
options.put("name", index.getName());
if (index.isUnique()) {
options.put("unique", index.isUnique());
}
if (index.isSparse()) {
options.put("sparse", index.isSparse());
}
if (index.isDropDups()) {
options.put("dropDups", index.isDropDups());
}
if (index.isBackground()) {
options.put("background", index.isBackground());
}
if (index.getExpireAfterSeconds() != -1) {
options.put("expireAfterSeconds", index.getExpireAfterSeconds());
}
// extras not supported yet, this is an adapter after all
surrogate.createIndex(keys, options);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy