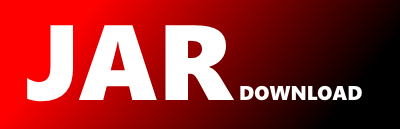
me.magicall.game.sub.chess.Position Maven / Gradle / Ivy
/*
* Copyright (c) 2024 Liang Wenjian
* magicall is licensed under Mulan PSL v2.
* You can use this software according to the terms and conditions of the Mulan PSL v2.
* You may obtain a copy of Mulan PSL v2 at:
* http://license.coscl.org.cn/MulanPSL2
* THIS SOFTWARE IS PROVIDED ON AN "AS IS" BASIS, WITHOUT WARRANTIES OF ANY KIND,
* EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO NON-INFRINGEMENT,
* MERCHANTABILITY OR FIT FOR A PARTICULAR PURPOSE.
* See the Mulan PSL v2 for more details.
*/
package me.magicall.game.sub.chess;
import me.magicall.game.event.EventTarget;
import me.magicall.game.map.Coordinate;
import me.magicall.game.util.GameUtil;
public class Position implements Coordinate, Comparable, EventTarget {
private final int row;
private final int column;
public Position(final int row, final int column) {
super();
this.row = row;
this.column = column;
}
public static Position[][] buildPositions(final int rows, final int columns) {
final var rt = new Position[rows][columns];
for (var i = 0; i < rows; ++i) {
for (var j = 0; j < columns; ++j) {
rt[i][j] = new Position(i, j);
}
}
return rt;
}
public int getRow() {
return row;
}
public int getColumn() {
return column;
}
@Override
public int compareTo(final Position o) {
return GameUtil.COORDINATE_COMPARATOR.compare(this, o);
}
@Override
public int[] getCoordinateIndexes() {
return new int[] {getRow(), getColumn()};
}
public boolean isSameColumn(final Position other) {
return getColumn() == other.getColumn();
}
public boolean isLefterThan(final Position other) {
return getColumn() < other.getColumn();
}
public boolean isRighterThan(final Position other) {
return getColumn() > other.getColumn();
}
public boolean isSameRow(final Position other) {
return getRow() == other.getRow();
}
public boolean isHigherThan(final Position other) {
return getRow() < other.getRow();
}
public boolean isLowerThan(final Position other) {
return getRow() > other.getRow();
}
public Position left(final int step) {
return new Position(getRow(), getColumn() - step);
}
public Position right(final int step) {
return new Position(getRow(), getColumn() + step);
}
public Position up(final int step) {
return new Position(getRow() - step, getColumn());
}
public Position down(final int step) {
return new Position(getRow() + step, getColumn());
}
public Position add(final Position position) {
return new Position(getRow() + position.getRow(), getColumn() + position.getColumn());
}
@Override
public String toString() {
return "(" + row + ',' + column + ')';
}
@Override
public int hashCode() {
final var prime = 31;
var result = 1;
result = prime * result + column;
result = prime * result + row;
return result;
}
@Override
public boolean equals(final Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final var other = (Position) obj;
if (column != other.column) {
return false;
}
return row == other.row;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy