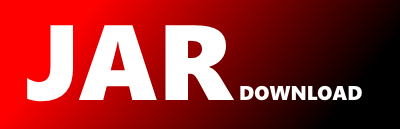
me.magicall.biz.service.DomainServices Maven / Gradle / Ivy
/*
* Copyright (c) 2024 Liang Wenjian
* magicall is licensed under Mulan PSL v2.
* You can use this software according to the terms and conditions of the Mulan PSL v2.
* You may obtain a copy of Mulan PSL v2 at:
* http://license.coscl.org.cn/MulanPSL2
* THIS SOFTWARE IS PROVIDED ON AN "AS IS" BASIS, WITHOUT WARRANTIES OF ANY KIND,
* EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO NON-INFRINGEMENT,
* MERCHANTABILITY OR FIT FOR A PARTICULAR PURPOSE.
* See the Mulan PSL v2 for more details.
*/
package me.magicall.biz.service;
import me.magicall.biz.DomainObject;
import me.magicall.dear_sun.exception.NoSuchThingException;
import me.magicall.dear_sun.exception.NullValException;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public interface DomainServices<_Id, _Domain extends DomainObject<_Id, _ValueObject>, _ValueObject>
extends Services<_Domain, _ValueObject> {
/**
* 寻找指定id的资源对象。
*
* @param id 指定的id。
* @return 若不存在,返回null。
*/
default _Domain find(final _Id id) {
checkId(id);
return filter(e -> id.equals(e.id())).findFirst().orElse(null);
}
/**
* 获取指定id的资源对象。若不存在,抛出 {@link NoSuchThingException},所以若期望当元素不存在时不抛异常,使用 {@link #find(Object)} 。
*
* @param id 指定的id。
* @return 若不存在,抛出 {@link NoSuchThingException}。
* @throws NoSuchThingException 若未找到,抛出 {@link NoSuchThingException}。
*/
default _Domain get(final _Id id) {
checkId(id);
final var one = find(id);
if (one == null) {
throw new NoSuchThingException(resourceName(), id.toString());
}
return one;
}
/**
* 检查指定id的资源对象是否存在。
*
* @param id 指定的id。
* @return 指定的资源对象。
*/
default boolean exists(final _Id id) {
checkId(id);
return streaming().anyMatch(e -> id.equals(e.id()));
}
/**
* 获取指定id列表对应的资源对象。不存在的资源对象不会出现在返回流中。
*
* @param ids 指定的id列表
* @return 指定的资源对象流。
*/
default Stream<_Domain> some(final Stream<_Id> ids) {
final var idSet = ids.collect(Collectors.toSet());
if (idSet.isEmpty()) {
return Stream.empty();
}
return filter(e -> idSet.contains(e.id()));
}
default long count(final Stream<_Id> ids) {
return some(ids).count();
}
/**
* 丢弃指定id的资源对象。
*
* @param id 指定的id。
* @return 被丢弃的对象。若不存在,则返回null。
*/
default _Domain dropById(final _Id id) {
checkId(id);
return drop(e -> e.id().equals(id)).findFirst().orElse(null);
}
/**
* 丢弃指定id列表对应的资源对象。不存在的资源对象不会出现在返回流中。
*
* @param ids 指定的id列表。
* @return 被丢弃的资源对象。
*/
default Stream<_Domain> dropById(final Stream<_Id> ids) {
final var idSet = ids.collect(Collectors.toSet());
if (idSet.isEmpty()) {
return Stream.empty();
}
return drop(e -> idSet.contains(e.id()));
}
/**
* 检查id。
*
* @param id id。
* @throws NullValException id为null。
*/
default void checkId(final _Id id) {
if (id == null) {
throw new NullValException("id");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy