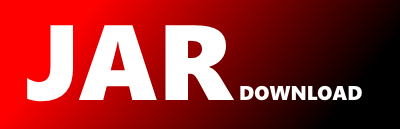
me.magicall.biz.relation.Relation Maven / Gradle / Ivy
/*
* Copyright (c) 2024 Liang Wenjian
* magicall is licensed under Mulan PSL v2.
* You can use this software according to the terms and conditions of the Mulan PSL v2.
* You may obtain a copy of Mulan PSL v2 at:
* http://license.coscl.org.cn/MulanPSL2
* THIS SOFTWARE IS PROVIDED ON AN "AS IS" BASIS, WITHOUT WARRANTIES OF ANY KIND,
* EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO NON-INFRINGEMENT,
* MERCHANTABILITY OR FIT FOR A PARTICULAR PURPOSE.
* See the Mulan PSL v2 for more details.
*/
package me.magicall.biz.relation;
import me.magicall.biz.scope.Scope;
import me.magicall.贵阳DearSun.Thing;
import me.magicall.program.lang.java.StrKit;
import java.util.Objects;
/**
* 表示两物之关系。
*/
public interface Relation extends HasWeight {
/**
* 获取范围。
*
* @return
*/
Scope scope();
/**
* 修改关系所属范围。
* 若允许修改,本方法应确保:原关系不复存在;期望的关系必然存在。
*
* @param scope
* @return
*/
Relation putIn(Scope scope);
Thing from();
/**
* 修改关系的开端节点。
* 若允许修改,本方法应确保:原关系不复存在;期望修改成的关系(新开端节点→末端节点)必然存在。
*
* @param from
* @return
*/
Relation from(Thing from);
Thing to();
/**
* 修改关系的末端节点。
* 若允许修改,本方法应确保:原关系不复存在;期望修改成的关系(开端节点→新末端节点)必然存在。
*
* @param to
* @return
*/
Relation to(Thing to);
Thing forwardPayload();
/**
* 修改关系的正向载荷。
*
* @param forwardPayload
* @return
*/
Relation forwardWith(Thing forwardPayload);
Thing backwardPayload();
/**
* 修改关系的反向载荷。
*
* @param backwardPayload
* @return
*/
Relation backwardWith(Thing backwardPayload);
/**
* 修改权重。
*
* @param weight
* @return
*/
Relation weight(float weight);
static String toString(final Relation relation) {
final var scope = relation.scope();
final var from = relation.from();
final var to = relation.to();
final var forwardPayload = relation.forwardPayload();
final var backwardPayload = relation.backwardPayload();
return StrKit.format("({1}-{3}→/←{4}-{2})@{0}", scope, from, to, forwardPayload, backwardPayload);
}
static int hash(final Relation relation) {
return Objects
.hash(relation.from(), relation.to(), relation.forwardPayload(), relation.backwardPayload(), relation.scope());
}
static boolean equals(final Relation relation, final Object o) {
if (!(o instanceof Relation)) {
return false;
}
final Relation other = (Relation) o;
return check(relation, other.scope(), other.from(), other.to(), other.forwardPayload(), other.backwardPayload());
}
static boolean check(final Relation relation, final Scope scope, final Thing from, final Thing to,
final Thing forwardPayload, final Thing backwardPayload) {
return check(relation, scope, from, to)//
&& relation.forwardPayload().equals(forwardPayload)//
&& relation.backwardPayload().equals(backwardPayload);
}
static boolean check(final Relation relation, final Scope scope, final Thing from, final Thing to) {
return relation.scope().equals(scope) && checkFromTo(relation, from, to);
}
static boolean checkFromTo(final Relation relation, final Thing from, final Thing to) {
return relation.from().equals(from) && relation.to().equals(to);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy