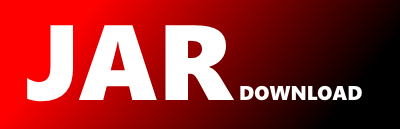
me.prettyprint.hom.CFMappingDef Maven / Gradle / Ivy
package me.prettyprint.hom;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import javax.persistence.DiscriminatorType;
import javax.persistence.Entity;
import javax.persistence.InheritanceType;
import me.prettyprint.hom.cache.HectorObjectMapperException;
import com.google.common.collect.Sets;
/**
* Holder for the mapping between a Class annotated with {@link Entity} and the
* Cassandra column family name.
*
* @author Todd Burruss
*
* @param
*/
public class CFMappingDef {
private Class realClass;
private Class effectiveClass;
private CFMappingDef super T> cfBaseMapDef;
private CFMappingDef super T> cfSuperMapDef;
private String colFamName;
private InheritanceType inheritanceType;
private String discColumn;
private DiscriminatorType discType;
private Object discValue; // this can be a variety of types
private String[] sliceColumnNameArr;
private Method anonymousPropertyAddHandler;
private KeyDefinition keyDef;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy