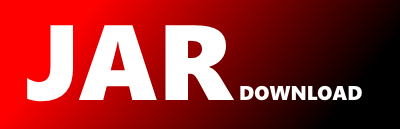
me.saro.sap.jco.SapUtils Maven / Gradle / Ivy
package me.saro.sap.jco;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Supplier;
import com.sap.conn.jco.JCoField;
import com.sap.conn.jco.JCoTable;
import me.saro.commons.Converter;
import me.saro.commons.function.ThrowableBiConsumer;
import me.saro.commons.function.ThrowableConsumer;
/**
* Sap Util
* @author PARK Yong Seo
* @since 0.1
*/
public class SapUtils {
// this class have only static method
private SapUtils() {
}
/**
* JCoTable to List[Map[String, Object]]
* @param table
* jCo table
* @return
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy