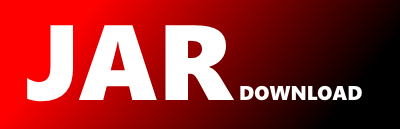
me.scolastico.mysql.manager.MysqlManager Maven / Gradle / Ivy
package me.scolastico.mysql.manager;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import me.scolastico.mysql.manager.dataholders.Database;
import me.scolastico.mysql.manager.dataholders.Fields;
import me.scolastico.mysql.manager.etc.SimplifiedFunctions;
import me.scolastico.mysql.manager.exceptions.NoDataException;
import me.scolastico.mysql.manager.exceptions.NoFieldsException;
import me.scolastico.mysql.manager.exceptions.NotATableException;
import me.scolastico.mysql.manager.exceptions.NotSavedEntryException;
import me.scolastico.mysql.manager.interfaces.Annotations.Table;
import me.scolastico.mysql.manager.interfaces.Annotations.TableEntry;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
public class MysqlManager {
private final String host;
private final int port;
private final String db;
private final String username;
private final String password;
private final boolean sqLite;
private final Connection connection;
private final Gson gson = new GsonBuilder().create();
public MysqlManager(String sqLiteFileName) throws SQLException {
this.host = sqLiteFileName;
this.port = 0;
this.db = null;
this.username = null;
this.password = null;
this.sqLite = true;
this.connection = DriverManager.getConnection("jdbc:sqlite:" + sqLiteFileName);
try {
Database.generateTable(this);} catch (NotATableException | NoSuchMethodException | NotSavedEntryException | NoFieldsException | InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchFieldException | NoDataException ignored) {}
}
public MysqlManager(String host, int port, String db, String username, String password) throws SQLException {
this.host = host;
this.port = port;
this.db = db;
this.username = username;
this.password = password;
this.sqLite = false;
this.connection = DriverManager.getConnection(
"jdbc:mysql://" + host + "/" + db +
"?user=" + SimplifiedFunctions.urlEncode(username) +
"&password=" + SimplifiedFunctions.urlEncode(password) +
"&autoReconnect=true"
);
try {Database.generateTable(this);} catch (NotATableException | NoSuchMethodException | NotSavedEntryException | NoFieldsException | InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchFieldException | NoDataException ignored) {}
}
public MysqlManager(String host, int port, String db, String username, String password, String additionalArguments) throws SQLException {
this.host = host;
this.port = port;
this.db = db;
this.username = username;
this.password = password;
this.sqLite = false;
this.connection = DriverManager.getConnection(
"jdbc:mysql://" + host + "/" + db +
"?user=" + SimplifiedFunctions.urlEncode(username) +
"&password=" + SimplifiedFunctions.urlEncode(password) +
"&autoReconnect=true" +
"&" + additionalArguments
);
try {Database.generateTable(this);} catch (NotATableException | NoSuchMethodException | NotSavedEntryException | NoFieldsException | InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchFieldException | NoDataException ignored) {}
}
public void generateTable(Object table) throws NotATableException, SQLException, InvocationTargetException, NoSuchMethodException, InstantiationException, IllegalAccessException, NoSuchFieldException, NoFieldsException, NotSavedEntryException, NoDataException {
String tableName = getTableName(table);
Map fields = getAllFields(table);
StringBuilder value = new StringBuilder();
for (String key:fields.keySet()) {
Field field = fields.get(key);
getKeysWithType(value, key, field);
}
if (!tableName.equals("MySQL_Manager_Internal")) {
Database[] databases = Database.searchByDatabaseName(tableName, this);
if (databases.length == 1) {
Database database = databases[0];
ArrayList notExistingFields = new ArrayList<>(database.getFields().getFieldsList());
for (String key:fields.keySet()) {
notExistingFields.remove(key);
Field field = fields.get(key);
if (!database.getFields().getFieldsList().contains(key)) {
String query = null;
if (field.getType() == String.class) {
query = "ALTER TABLE `" + tableName + "` ADD `" + key + "` text;";
} else if (field.getType() == Integer.class) {
query = "ALTER TABLE `" + tableName + "` ADD `" + key + "` int;";
} else if (field.getType() == Long.class) {
query = "ALTER TABLE `" + tableName + "` ADD `" + key + "` bigint;";
} else if (field.getType() == Double.class) {
query = "ALTER TABLE `" + tableName + "` ADD `" + key + "` double;";
} else {
query = "ALTER TABLE `" + tableName + "` ADD `" + key + "` text;";
}
connection.prepareStatement(query).execute();
database.getFields().getFieldsList().add(key);
}
}
if (sqLite && notExistingFields.size() > 0) {
StringBuilder keysWithType = new StringBuilder();
StringBuilder keys = new StringBuilder();
for (String key:fields.keySet()) {
Field field = fields.get(key);
keys.append(", ").append(key);
getKeysWithType(keysWithType, key, field);
}
String[] query = {
"PRAGMA foreign_keys=off;",
"ALTER TABLE " + tableName + " RENAME TO _old_" + tableName + ";",
"CREATE TABLE `" + tableName + "` (`id` INTEGER PRIMARY KEY" + keysWithType.toString() + ");",
"INSERT INTO " + tableName + " (id" + keys.toString() + ") SELECT id" + keys.toString() + " FROM _old_" + tableName + ";",
"PRAGMA foreign_keys=on;",
"DROP TABLE _old_" + tableName + ";"
};
for (String q:query) {
connection.prepareStatement(q).execute();
}
} else {
for (String field:notExistingFields) {
connection.prepareStatement("ALTER TABLE " + tableName + " DROP " + field + ";").execute();
database.getFields().getFieldsList().remove(field);
}
}
database.update();
return;
}
}
if (sqLite) {
connection.prepareStatement("CREATE TABLE IF NOT EXISTS `" + tableName + "` (`id` INTEGER PRIMARY KEY" + value.toString() + ");").execute();
} else {
connection.prepareStatement("CREATE TABLE IF NOT EXISTS `" + tableName + "` (`id` INT NOT NULL AUTO_INCREMENT" + value.toString() + ", PRIMARY KEY( `id` ));").execute();
}
if (!tableName.equals("MySQL_Manager_Internal")) {
Fields f = new Fields();
ArrayList fieldList = new ArrayList<>(fields.keySet());
f.setFieldsList(fieldList);
new Database(this, tableName, f).save();
}
}
private void getKeysWithType(StringBuilder keysWithType, String key, Field field) {
if (field.getType() == String.class) {
keysWithType.append(", `").append(key).append("` text");
} else if (field.getType() == Integer.class) {
keysWithType.append(", `").append(key).append("` int");
} else if (field.getType() == Long.class) {
keysWithType.append(", `").append(key).append("` bigint");
} else if (field.getType() == Double.class) {
keysWithType.append(", `").append(key).append("` double");
} else {
keysWithType.append(", `").append(key).append("` text");
}
}
public Object getFromTableById(Object table, Long id) throws NotATableException, SQLException, IllegalAccessException, NoSuchFieldException, InvocationTargetException, NoSuchMethodException {
String tableName = getTableName(table);
ResultSet rs = connection.createStatement().executeQuery("SELECT * FROM `" + tableName + "` WHERE id=" + id + ";");
if (rs.next()) {
Map fields = getAllFields(table);
fillDataHolder(table, rs, fields, table);
rs.close();
rs.getStatement().close();
return table;
}
return null;
}
public Object[] getFromTableBySearch(Object table, String name, Object object) throws NotATableException, SQLException, InvocationTargetException, IllegalAccessException, NoSuchMethodException, NoSuchFieldException, InstantiationException {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy