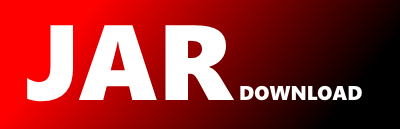
me.shaftesbury.utils.functional.BinaryFunction Maven / Gradle / Ivy
package me.shaftesbury.utils.functional;
/**
* An implementation of {@link me.shaftesbury.utils.functional.Func2} that is intended for use by the {@link me.shaftesbury.utils.functional.MException}
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the return value of the function
*/
public abstract class BinaryFunction implements Func2
{
/**
* Create a curried unary function from this binary function
* @return a function which takes an argument of type B and returns a function that accepts an argument of type A and returns
* an object of type C
*/
public Func> toFunc()
{
return toUnaryFunc(this);
}
/**
* Helper function. The same as {@link #toFunc(Object)} above with the addition that the curried function is partially applied
* by passing the argument b
* @param b the argument to be passed to the curried function
* @return a function that accepts an argument of type A and returns an object of type C
*/
public Func toFunc(B b)
{
return toUnaryFunc(this).apply(b);
}
private static final Func> toUnaryFunc(final BinaryFunction f)
{
return new Func>()
{
@Override
public Func apply(final B b) {
return new Func() {
@Override
public C apply(final A a) {
return f.apply(a,b);
}
};
}
};
}
/**
* Given a {@link me.shaftesbury.utils.functional.Func2} and its two arguments, produce a function of no arguments that
* can be evaluated at a later point.
* @param f the two-parameter function
* @param a the first parameter of f
* @param b the second parameter of f
* @param the type of the first parameter of f
* @param the type of the second parameter of f
* @param the type of the return value of the delayed function
* @return a function that takes no arguments and returns a value of type C
*/
public static final Func0 delay(final Func2 f, final A a, final B b)
{
return new Func0() {
@Override
public C apply() {
return f.apply(a,b);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy