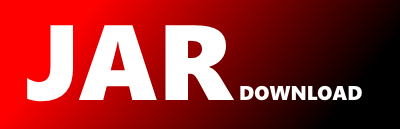
me.shaftesbury.utils.functional.FunctionalHelper Maven / Gradle / Ivy
package me.shaftesbury.utils.functional;
/**
* Created by Bob on 16/05/14.
*
* Common use patterns written here so that we don't have to wrestle continuously with the clunkiness and waffle that is the Java syntax.
*/
public final class FunctionalHelper
{
/**
* Given an input sequence of {@link me.shaftesbury.utils.functional.Option} types return a new lazily-evaluated sequence of those which are {@link me.shaftesbury.utils.functional.Option#Some()}
* @param input the input sequence
* @param the type of the element underlying the {@link me.shaftesbury.utils.functional.Option}
* @return a sequence of {@link me.shaftesbury.utils.functional.Option} which are {@link me.shaftesbury.utils.functional.Option#Some()}
*/
public static Iterable2
© 2015 - 2024 Weber Informatics LLC | Privacy Policy