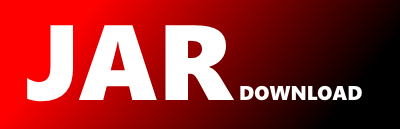
annotations.me.snowdrop.applicationcrd.api.model.IngressSelectorFluentImpl Maven / Gradle / Ivy
package me.snowdrop.applicationcrd.api.model;
import java.lang.StringBuffer;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
import java.lang.String;
import java.lang.Boolean;
import javax.validation.constraints.NotNull;
public class IngressSelectorFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements IngressSelectorFluent{
private String apiVersion;
private String fieldPath;
private String host;
private String kind;
private String name;
private String namespace;
private String path;
private String resourceVersion;
private String uid;
public IngressSelectorFluentImpl(){
}
public IngressSelectorFluentImpl(IngressSelector instance){
this.withApiVersion(instance.getApiVersion());
this.withFieldPath(instance.getFieldPath());
this.withHost(instance.getHost());
this.withKind(instance.getKind());
this.withName(instance.getName());
this.withNamespace(instance.getNamespace());
this.withPath(instance.getPath());
this.withResourceVersion(instance.getResourceVersion());
this.withUid(instance.getUid());
}
public String getApiVersion(){
return this.apiVersion;
}
public A withApiVersion(String apiVersion){
this.apiVersion=apiVersion; return (A) this;
}
public Boolean hasApiVersion(){
return this.apiVersion != null;
}
public A withNewApiVersion(String arg1){
return (A)withApiVersion(new String(arg1));
}
public A withNewApiVersion(StringBuilder arg1){
return (A)withApiVersion(new String(arg1));
}
public A withNewApiVersion(StringBuffer arg1){
return (A)withApiVersion(new String(arg1));
}
public String getFieldPath(){
return this.fieldPath;
}
public A withFieldPath(String fieldPath){
this.fieldPath=fieldPath; return (A) this;
}
public Boolean hasFieldPath(){
return this.fieldPath != null;
}
public A withNewFieldPath(String arg1){
return (A)withFieldPath(new String(arg1));
}
public A withNewFieldPath(StringBuilder arg1){
return (A)withFieldPath(new String(arg1));
}
public A withNewFieldPath(StringBuffer arg1){
return (A)withFieldPath(new String(arg1));
}
public String getHost(){
return this.host;
}
public A withHost(String host){
this.host=host; return (A) this;
}
public Boolean hasHost(){
return this.host != null;
}
public A withNewHost(String arg1){
return (A)withHost(new String(arg1));
}
public A withNewHost(StringBuilder arg1){
return (A)withHost(new String(arg1));
}
public A withNewHost(StringBuffer arg1){
return (A)withHost(new String(arg1));
}
public String getKind(){
return this.kind;
}
public A withKind(String kind){
this.kind=kind; return (A) this;
}
public Boolean hasKind(){
return this.kind != null;
}
public A withNewKind(String arg1){
return (A)withKind(new String(arg1));
}
public A withNewKind(StringBuilder arg1){
return (A)withKind(new String(arg1));
}
public A withNewKind(StringBuffer arg1){
return (A)withKind(new String(arg1));
}
public String getName(){
return this.name;
}
public A withName(String name){
this.name=name; return (A) this;
}
public Boolean hasName(){
return this.name != null;
}
public A withNewName(String arg1){
return (A)withName(new String(arg1));
}
public A withNewName(StringBuilder arg1){
return (A)withName(new String(arg1));
}
public A withNewName(StringBuffer arg1){
return (A)withName(new String(arg1));
}
public String getNamespace(){
return this.namespace;
}
public A withNamespace(String namespace){
this.namespace=namespace; return (A) this;
}
public Boolean hasNamespace(){
return this.namespace != null;
}
public A withNewNamespace(String arg1){
return (A)withNamespace(new String(arg1));
}
public A withNewNamespace(StringBuilder arg1){
return (A)withNamespace(new String(arg1));
}
public A withNewNamespace(StringBuffer arg1){
return (A)withNamespace(new String(arg1));
}
public String getPath(){
return this.path;
}
public A withPath(String path){
this.path=path; return (A) this;
}
public Boolean hasPath(){
return this.path != null;
}
public A withNewPath(String arg1){
return (A)withPath(new String(arg1));
}
public A withNewPath(StringBuilder arg1){
return (A)withPath(new String(arg1));
}
public A withNewPath(StringBuffer arg1){
return (A)withPath(new String(arg1));
}
public String getResourceVersion(){
return this.resourceVersion;
}
public A withResourceVersion(String resourceVersion){
this.resourceVersion=resourceVersion; return (A) this;
}
public Boolean hasResourceVersion(){
return this.resourceVersion != null;
}
public A withNewResourceVersion(String arg1){
return (A)withResourceVersion(new String(arg1));
}
public A withNewResourceVersion(StringBuilder arg1){
return (A)withResourceVersion(new String(arg1));
}
public A withNewResourceVersion(StringBuffer arg1){
return (A)withResourceVersion(new String(arg1));
}
public String getUid(){
return this.uid;
}
public A withUid(String uid){
this.uid=uid; return (A) this;
}
public Boolean hasUid(){
return this.uid != null;
}
public A withNewUid(String arg1){
return (A)withUid(new String(arg1));
}
public A withNewUid(StringBuilder arg1){
return (A)withUid(new String(arg1));
}
public A withNewUid(StringBuffer arg1){
return (A)withUid(new String(arg1));
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
IngressSelectorFluentImpl that = (IngressSelectorFluentImpl) o;
if (apiVersion != null ? !apiVersion.equals(that.apiVersion) :that.apiVersion != null) return false;
if (fieldPath != null ? !fieldPath.equals(that.fieldPath) :that.fieldPath != null) return false;
if (host != null ? !host.equals(that.host) :that.host != null) return false;
if (kind != null ? !kind.equals(that.kind) :that.kind != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (namespace != null ? !namespace.equals(that.namespace) :that.namespace != null) return false;
if (path != null ? !path.equals(that.path) :that.path != null) return false;
if (resourceVersion != null ? !resourceVersion.equals(that.resourceVersion) :that.resourceVersion != null) return false;
if (uid != null ? !uid.equals(that.uid) :that.uid != null) return false;
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy