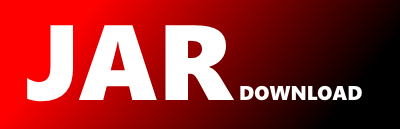
org.mentacontainer.impl.GenericFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of menta-container Show documentation
Show all versions of menta-container Show documentation
A IOC container as simple and pragmatic as it can get with programmatic configuration through a Fluent API.
package org.mentacontainer.impl;
import java.lang.reflect.Method;
import org.mentacontainer.Factory;
import org.mentacontainer.Interceptor;
import org.mentacontainer.util.FindMethod;
public class GenericFactory implements Factory, Interceptor {
private final Object factory;
private final Method method;
private final Class> type;
private Interceptor interceptor = null;
public GenericFactory(Object factory, String methodName) {
this.factory = factory;
try {
this.method = FindMethod.getMethod(factory.getClass(), methodName, new Class[] { });
this.method.setAccessible(true);
this.type = method.getReturnType();
} catch(Exception e) {
throw new RuntimeException(e);
}
}
public void setInterceptor(Interceptor interceptor) {
this.interceptor = interceptor;
}
@Override
public void onCreated(E createdObject) {
if (interceptor != null) {
interceptor.onCreated(createdObject);
}
}
@Override
public void onCleared(E clearedObject) {
if (interceptor != null) {
interceptor.onCleared(clearedObject);
}
}
@Override
public T getInstance() {
try {
return (T) method.invoke(factory, (Object[]) null);
} catch(Exception e) {
throw new RuntimeException("Cannot invoke method: " + method, e);
}
}
@Override
public Class> getType() {
return type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy