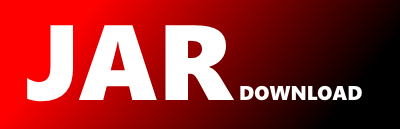
org.mentaqueue.AtomicQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of menta-queue Show documentation
Show all versions of menta-queue Show documentation
A super fast inter-thread transfer queue.
The newest version!
/*
* MentaQueue => http://mentaqueue.soliveirajr.com
* Copyright (C) 2012 Sergio Oliveira Jr. ([email protected])
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
package org.mentaqueue;
import static org.mentaqueue.util.BuilderUtils.createBuilder;
import org.mentaqueue.util.Builder;
import org.mentaqueue.util.NumberUtils;
import org.mentaqueue.util.PaddedAtomicLong;
public class AtomicQueue implements BatchingQueue {
private final static int DEFAULT_CAPACITY = 1024 * 16;
private final int capacity;
private final int capacityMinusOne;
private final E[] data;
private long lastOfferedSeq = -1;
private long lastPolledSeq = -1;
private long pollCount = 0;
private long maxSeqBeforeWrapping;
private final PaddedAtomicLong offerSequence = new PaddedAtomicLong(-1);
private final PaddedAtomicLong pollSequence = new PaddedAtomicLong(-1);
@SuppressWarnings("unchecked")
public AtomicQueue(int capacity, Builder builder) {
NumberUtils.ensurePowerOfTwo(capacity);
this.capacity = capacity;
this.capacityMinusOne = capacity - 1;
this.data = (E[]) new Object[capacity];
for (int i = 0; i < capacity; i++) {
this.data[i] = builder.newInstance();
}
this.maxSeqBeforeWrapping = calcMaxSeqBeforeWrapping();
}
public AtomicQueue(Builder builder) {
this(DEFAULT_CAPACITY, builder);
}
public AtomicQueue(Class klass) {
this(createBuilder(klass));
}
public AtomicQueue(int capacity, Class klass) {
this(capacity, createBuilder(klass));
}
private final long calcMaxSeqBeforeWrapping() {
return pollSequence.get() + capacity;
}
@Override
public final E nextToDispatch() {
if (++lastOfferedSeq > maxSeqBeforeWrapping) {
// this would wrap the buffer... calculate the new one...
this.maxSeqBeforeWrapping = calcMaxSeqBeforeWrapping();
if (lastOfferedSeq > maxSeqBeforeWrapping) {
lastOfferedSeq--;
return null;
}
}
return data[(int) (lastOfferedSeq & capacityMinusOne)];
}
@Override
public final void flush(boolean lazySet) {
if (lazySet) {
offerSequence.lazySet(lastOfferedSeq);
} else {
offerSequence.set(lastOfferedSeq);
}
}
@Override
public final void flush() {
flush(false);
}
@Override
public final long availableToPoll() {
return offerSequence.get() - lastPolledSeq;
}
@Override
public final E poll() {
pollCount++;
return data[(int) (++lastPolledSeq & capacityMinusOne)];
}
@Override
public final E peek() {
return data[(int) (lastPolledSeq & capacityMinusOne)];
}
@Override
public final void donePolling(boolean lazySet) {
if (lazySet) {
pollSequence.lazySet(lastPolledSeq);
} else {
pollSequence.set(lastPolledSeq);
}
pollCount = 0;
}
@Override
public final void rollback() {
rollback(pollCount);
}
@Override
public final void rollback(long count) {
if (count < 0 || count > pollCount) {
throw new RuntimeException("Invalid rollback request! polled=" + pollCount + " requested=" + count);
}
lastPolledSeq -= count;
pollCount -= count;
}
@Override
public final void donePolling() {
donePolling(false);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy