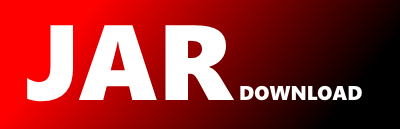
org.mentaqueue.test.sample.Example Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of menta-queue Show documentation
Show all versions of menta-queue Show documentation
A super fast inter-thread transfer queue.
The newest version!
package org.mentaqueue.test.sample;
import org.mentaqueue.AtomicQueue;
import org.mentaqueue.BatchingQueue;
import org.mentaqueue.util.Builder;
public class Example {
public static void main(String[] args) {
final BatchingQueue queue = new AtomicQueue(1024, new Builder() {
@Override
public StringBuilder newInstance() {
return new StringBuilder(1024);
}
});
Thread producer = new Thread(new Runnable() {
@Override
public void run() {
StringBuilder sb;
while(true) { // the main loop of the thread
// (...) do whatever you have to do here...
// and whenever you want to send a message to
// the other thread you can just do:
sb = queue.nextToDispatch();
sb.setLength(0);
sb.append("Hello!");
queue.flush();
// you can also send in batches to increase throughput:
sb = queue.nextToDispatch();
sb.setLength(0);
sb.append("Hi!");
sb = queue.nextToDispatch();
sb.setLength(0);
sb.append("Hi again!");
queue.flush(); // dispatch the two messages above...
}
}
}, "Thread-Producer");
Thread consumer = new Thread(new Runnable() {
@Override
public void run() {
while (true) {
// (...) do whatever you have to do here...
// and whenever you want to check if the producer
// has sent a message you just do:
long avail = queue.availableToPoll();
if (avail > 0) {
for(int i = 0; i < avail; i++) {
StringBuilder sb = queue.poll();
// (...) do whatever you want to do with the data
}
queue.donePolling();
}
}
}
}, "Thread-Consumer");
consumer.start();
producer.start();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy