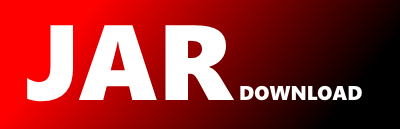
Java.target.apidocs.org.antlr.v4.runtime.Parser.html Maven / Gradle / Ivy
Show all versions of antlr4-perf-testsuite Show documentation
Parser (ANTLR 4 Runtime (Optimized) 4.13.1.3 API)
org.antlr.v4.runtime
Class Parser
- java.lang.Object
-
- org.antlr.v4.runtime.Recognizer<Token,ParserATNSimulator>
-
- org.antlr.v4.runtime.Parser
-
- Direct Known Subclasses:
- ParserInterpreter
public abstract class Parser
extends Recognizer<Token,ParserATNSimulator>
This is all the parsing support code essentially; most of it is error recovery stuff.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
Parser.TraceListener
static class
Parser.TrimToSizeListener
-
Field Summary
Fields
Modifier and Type
Field and Description
protected boolean
_buildParseTrees
Specifies whether or not the parser should construct a parse tree during
the parsing process.
protected ParserRuleContext
_ctx
The ParserRuleContext
object for the currently executing rule.
protected ANTLRErrorStrategy
_errHandler
The error handling strategy for the parser.
protected TokenStream
_input
The input stream.
protected List<ParseTreeListener>
_parseListeners
The list of ParseTreeListener
listeners registered to receive
events during the parse.
protected IntegerStack
_precedenceStack
protected int
_syntaxErrors
The number of syntax errors reported during parsing.
protected boolean
matchedEOF
Indicates parser has match()ed EOF token.
-
Fields inherited from class org.antlr.v4.runtime.Recognizer
_interp, EOF
-
Constructor Summary
Constructors
Constructor and Description
Parser(TokenStream input)
-
Method Summary
All Methods Instance Methods Concrete Methods Deprecated Methods
Modifier and Type
Method and Description
protected void
addContextToParseTree()
void
addParseListener(ParseTreeListener listener)
Registers listener
to receive events during the parsing process.
ParseTreePattern
compileParseTreePattern(String pattern,
int patternRuleIndex)
The preferred method of getting a tree pattern.
ParseTreePattern
compileParseTreePattern(String pattern,
int patternRuleIndex,
Lexer lexer)
The same as compileParseTreePattern(String, int)
but specify a
Lexer
rather than trying to deduce it from this parser.
Token
consume()
Consume and return the current symbol.
ErrorNode
createErrorNode(ParserRuleContext parent,
Token t)
How to create an error node, given a token, associated with a parent.
TerminalNode
createTerminalNode(ParserRuleContext parent,
Token t)
How to create a token leaf node associated with a parent.
void
dumpDFA()
For debugging and other purposes.
void
enterLeftFactoredRule(ParserRuleContext localctx,
int state,
int ruleIndex)
void
enterOuterAlt(ParserRuleContext localctx,
int altNum)
void
enterRecursionRule(ParserRuleContext localctx,
int ruleIndex)
Deprecated.
Use
enterRecursionRule(ParserRuleContext, int, int, int)
instead.
void
enterRecursionRule(ParserRuleContext localctx,
int state,
int ruleIndex,
int precedence)
void
enterRule(ParserRuleContext localctx,
int state,
int ruleIndex)
Always called by generated parsers upon entry to a rule.
void
exitRule()
ATN
getATNWithBypassAlts()
The ATN with bypass alternatives is expensive to create so we create it
lazily.
boolean
getBuildParseTree()
Gets whether or not a complete parse tree will be constructed while
parsing.
ParserRuleContext
getContext()
Token
getCurrentToken()
Match needs to return the current input symbol, which gets put
into the label for the associated token ref; e.g., x=ID.
List<String>
getDFAStrings()
For debugging and other purposes.
ANTLRErrorStrategy
getErrorHandler()
ParserErrorListener
getErrorListenerDispatch()
IntervalSet
getExpectedTokens()
Computes the set of input symbols which could follow the current parser
state and context, as given by Recognizer.getState()
and getContext()
,
respectively.
IntervalSet
getExpectedTokensWithinCurrentRule()
TokenStream
getInputStream()
ParserRuleContext
getInvokingContext(int ruleIndex)
int
getNumberOfSyntaxErrors()
Gets the number of syntax errors reported during parsing.
ParseInfo
getParseInfo()
If profiling during the parse/lex, this will return DecisionInfo records
for each decision in recognizer in a ParseInfo object.
List<ParseTreeListener>
getParseListeners()
int
getPrecedence()
Get the precedence level for the top-most precedence rule.
ParserRuleContext
getRuleContext()
int
getRuleIndex(String ruleName)
Get a rule's index (i.e., RULE_ruleName
field) or -1 if not found.
List<String>
getRuleInvocationStack()
Return List<String> of the rule names in your parser instance
leading up to a call to the current rule.
List<String>
getRuleInvocationStack(RuleContext p)
String
getSourceName()
TokenFactory
getTokenFactory()
boolean
getTrimParseTree()
boolean
inContext(String context)
boolean
isExpectedToken(int symbol)
Checks whether or not symbol
can follow the current state in the
ATN.
boolean
isMatchedEOF()
boolean
isTrace()
Gets whether a Parser.TraceListener
is registered as a parse listener
for the parser.
Token
match(int ttype)
Match current input symbol against ttype
.
Token
matchWildcard()
Match current input symbol as a wildcard.
void
notifyErrorListeners(String msg)
void
notifyErrorListeners(Token offendingToken,
String msg,
RecognitionException e)
boolean
precpred(RuleContext localctx,
int precedence)
void
pushNewRecursionContext(ParserRuleContext localctx,
int state,
int ruleIndex)
Like enterRule(org.antlr.v4.runtime.ParserRuleContext, int, int)
but for recursive rules.
void
removeParseListener(ParseTreeListener listener)
Remove listener
from the list of parse listeners.
void
removeParseListeners()
Remove all parse listeners.
void
reset()
reset the parser's state
void
setBuildParseTree(boolean buildParseTrees)
Track the ParserRuleContext
objects during the parse and hook
them up using the ParserRuleContext.children
list so that it
forms a parse tree.
void
setContext(ParserRuleContext ctx)
void
setErrorHandler(ANTLRErrorStrategy handler)
void
setInputStream(TokenStream input)
Set the token stream and reset the parser.
void
setProfile(boolean profile)
void
setTrace(boolean trace)
During a parse is sometimes useful to listen in on the rule entry and exit
events as well as token matches.
void
setTrimParseTree(boolean trimParseTrees)
Trim the internal lists of the parse tree during parsing to conserve memory.
protected void
triggerEnterRuleEvent()
Notify any parse listeners of an enter rule event.
protected void
triggerExitRuleEvent()
Notify any parse listeners of an exit rule event.
void
unrollRecursionContexts(ParserRuleContext _parentctx)
-
Methods inherited from class org.antlr.v4.runtime.Recognizer
action, addErrorListener, getATN, getErrorHeader, getErrorListeners, getGrammarFileName, getInterpreter, getRuleIndexMap, getRuleNames, getSerializedATN, getState, getTokenErrorDisplay, getTokenNames, getTokenType, getTokenTypeMap, getVocabulary, removeErrorListener, removeErrorListeners, sempred, setInterpreter, setState
-
-
Field Detail
-
_errHandler
@NotNull
protected ANTLRErrorStrategy _errHandler
The error handling strategy for the parser. The default value is a new
instance of DefaultErrorStrategy
.
-
_input
protected TokenStream _input
The input stream.
-
_precedenceStack
protected final IntegerStack _precedenceStack
-
_ctx
protected ParserRuleContext _ctx
The ParserRuleContext
object for the currently executing rule.
This is always non-null during the parsing process.
-
_buildParseTrees
protected boolean _buildParseTrees
Specifies whether or not the parser should construct a parse tree during
the parsing process. The default value is true
.
- See Also:
getBuildParseTree()
,
setBuildParseTree(boolean)
-
_parseListeners
@Nullable
protected List<ParseTreeListener> _parseListeners
The list of ParseTreeListener
listeners registered to receive
events during the parse.
-
_syntaxErrors
protected int _syntaxErrors
The number of syntax errors reported during parsing. This value is
incremented each time notifyErrorListeners(java.lang.String)
is called.
-
matchedEOF
protected boolean matchedEOF
Indicates parser has match()ed EOF token. See exitRule()
.
-
Constructor Detail
-
Parser
public Parser(TokenStream input)
-
Method Detail
-
reset
public void reset()
reset the parser's state
-
match
@NotNull
public Token match(int ttype)
throws RecognitionException
Match current input symbol against ttype
. If the symbol type
matches, ANTLRErrorStrategy.reportMatch(org.antlr.v4.runtime.Parser)
and consume()
are
called to complete the match process.
If the symbol type does not match,
ANTLRErrorStrategy.recoverInline(org.antlr.v4.runtime.Parser)
is called on the current error
strategy to attempt recovery. If getBuildParseTree()
is
true
and the token index of the symbol returned by
ANTLRErrorStrategy.recoverInline(org.antlr.v4.runtime.Parser)
is -1, the symbol is added to
the parse tree by calling createErrorNode(ParserRuleContext, Token)
then
ParserRuleContext.addErrorNode(ErrorNode)
.
- Parameters:
ttype
- the token type to match
- Returns:
- the matched symbol
- Throws:
RecognitionException
- if the current input symbol did not match
ttype
and the error strategy could not recover from the
mismatched symbol
-
matchWildcard
@NotNull
public Token matchWildcard()
throws RecognitionException
Match current input symbol as a wildcard. If the symbol type matches
(i.e. has a value greater than 0), ANTLRErrorStrategy.reportMatch(org.antlr.v4.runtime.Parser)
and consume()
are called to complete the match process.
If the symbol type does not match,
ANTLRErrorStrategy.recoverInline(org.antlr.v4.runtime.Parser)
is called on the current error
strategy to attempt recovery. If getBuildParseTree()
is
true
and the token index of the symbol returned by
ANTLRErrorStrategy.recoverInline(org.antlr.v4.runtime.Parser)
is -1, the symbol is added to
the parse tree by calling createErrorNode(ParserRuleContext, Token)
. then
ParserRuleContext.addErrorNode(ErrorNode)
- Returns:
- the matched symbol
- Throws:
RecognitionException
- if the current input symbol did not match
a wildcard and the error strategy could not recover from the mismatched
symbol
-
setBuildParseTree
public void setBuildParseTree(boolean buildParseTrees)
Track the ParserRuleContext
objects during the parse and hook
them up using the ParserRuleContext.children
list so that it
forms a parse tree. The ParserRuleContext
returned from the start
rule represents the root of the parse tree.
Note that if we are not building parse trees, rule contexts only point
upwards. When a rule exits, it returns the context but that gets garbage
collected if nobody holds a reference. It points upwards but nobody
points at it.
When we build parse trees, we are adding all of these contexts to
ParserRuleContext.children
list. Contexts are then not candidates
for garbage collection.
-
getBuildParseTree
public boolean getBuildParseTree()
Gets whether or not a complete parse tree will be constructed while
parsing. This property is true
for a newly constructed parser.
- Returns:
true
if a complete parse tree will be constructed while
parsing, otherwise false
-
setTrimParseTree
public void setTrimParseTree(boolean trimParseTrees)
Trim the internal lists of the parse tree during parsing to conserve memory.
This property is set to false
by default for a newly constructed parser.
- Parameters:
trimParseTrees
- true
to trim the capacity of the ParserRuleContext.children
list to its size after a rule is parsed.
-
getTrimParseTree
public boolean getTrimParseTree()
- Returns:
true
if the ParserRuleContext.children
list is trimmed
using the default Parser.TrimToSizeListener
during the parse process.
-
getParseListeners
@NotNull
public List<ParseTreeListener> getParseListeners()
-
addParseListener
public void addParseListener(@NotNull
ParseTreeListener listener)
Registers listener
to receive events during the parsing process.
To support output-preserving grammar transformations (including but not
limited to left-recursion removal, automated left-factoring, and
optimized code generation), calls to listener methods during the parse
may differ substantially from calls made by
ParseTreeWalker.DEFAULT
used after the parse is complete. In
particular, rule entry and exit events may occur in a different order
during the parse than after the parser. In addition, calls to certain
rule entry methods may be omitted.
With the following specific exceptions, calls to listener events are
deterministic, i.e. for identical input the calls to listener
methods will be the same.
- Alterations to the grammar used to generate code may change the
behavior of the listener calls.
- Alterations to the command line options passed to ANTLR 4 when
generating the parser may change the behavior of the listener calls.
- Changing the version of the ANTLR Tool used to generate the parser
may change the behavior of the listener calls.
- Parameters:
listener
- the listener to add
- Throws:
NullPointerException
- if listener is null
-
removeParseListener
public void removeParseListener(ParseTreeListener listener)
Remove listener
from the list of parse listeners.
If listener
is null
or has not been added as a parse
listener, this method does nothing.
- Parameters:
listener
- the listener to remove
- See Also:
addParseListener(org.antlr.v4.runtime.tree.ParseTreeListener)
-
removeParseListeners
public void removeParseListeners()
Remove all parse listeners.
-
triggerEnterRuleEvent
protected void triggerEnterRuleEvent()
Notify any parse listeners of an enter rule event.
-
triggerExitRuleEvent
protected void triggerExitRuleEvent()
Notify any parse listeners of an exit rule event.
-
getNumberOfSyntaxErrors
public int getNumberOfSyntaxErrors()
Gets the number of syntax errors reported during parsing. This value is
incremented each time notifyErrorListeners(java.lang.String)
is called.
- See Also:
notifyErrorListeners(java.lang.String)
-
getTokenFactory
public TokenFactory getTokenFactory()
-
getATNWithBypassAlts
@NotNull
public ATN getATNWithBypassAlts()
The ATN with bypass alternatives is expensive to create so we create it
lazily.
- Throws:
UnsupportedOperationException
- if the current parser does not
implement the Recognizer.getSerializedATN()
method.
-
compileParseTreePattern
public ParseTreePattern compileParseTreePattern(String pattern,
int patternRuleIndex)
The preferred method of getting a tree pattern. For example, here's a
sample use:
ParseTree t = parser.expr();
ParseTreePattern p = parser.compileParseTreePattern("<ID>+0", MyParser.RULE_expr);
ParseTreeMatch m = p.match(t);
String id = m.get("ID");
-
compileParseTreePattern
public ParseTreePattern compileParseTreePattern(String pattern,
int patternRuleIndex,
Lexer lexer)
The same as compileParseTreePattern(String, int)
but specify a
Lexer
rather than trying to deduce it from this parser.
-
getErrorHandler
@NotNull
public ANTLRErrorStrategy getErrorHandler()
-
setErrorHandler
public void setErrorHandler(@NotNull
ANTLRErrorStrategy handler)
-
getInputStream
public TokenStream getInputStream()
- Specified by:
getInputStream
in class Recognizer<Token,ParserATNSimulator>
-
setInputStream
public void setInputStream(TokenStream input)
Set the token stream and reset the parser.
-
getCurrentToken
@NotNull
public Token getCurrentToken()
Match needs to return the current input symbol, which gets put
into the label for the associated token ref; e.g., x=ID.
-
notifyErrorListeners
public final void notifyErrorListeners(@NotNull
String msg)
-
notifyErrorListeners
public void notifyErrorListeners(@NotNull
Token offendingToken,
@NotNull
String msg,
@Nullable
RecognitionException e)
-
consume
public Token consume()
Consume and return the current symbol.
E.g., given the following input with A
being the current
lookahead symbol, this function moves the cursor to B
and returns
A
.
A B
^
If the parser is not in error recovery mode, the consumed symbol is added
to the parse tree using ParserRuleContext.addChild(TerminalNode)
, and
ParseTreeListener.visitTerminal(org.antlr.v4.runtime.tree.TerminalNode)
is called on any parse listeners.
If the parser is in error recovery mode, the consumed symbol is
added to the parse tree using createErrorNode(ParserRuleContext, Token)
then
ParserRuleContext.addErrorNode(ErrorNode)
and
ParseTreeListener.visitErrorNode(org.antlr.v4.runtime.tree.ErrorNode)
is called on any parse
listeners.
-
createTerminalNode
public TerminalNode createTerminalNode(ParserRuleContext parent,
Token t)
How to create a token leaf node associated with a parent.
Typically, the terminal node to create is not a function of the parent.
- Since:
- 4.7
-
createErrorNode
public ErrorNode createErrorNode(ParserRuleContext parent,
Token t)
How to create an error node, given a token, associated with a parent.
Typically, the error node to create is not a function of the parent.
- Since:
- 4.7
-
addContextToParseTree
protected void addContextToParseTree()
-
enterRule
public void enterRule(@NotNull
ParserRuleContext localctx,
int state,
int ruleIndex)
Always called by generated parsers upon entry to a rule. Access field
_ctx
get the current context.
-
enterLeftFactoredRule
public void enterLeftFactoredRule(ParserRuleContext localctx,
int state,
int ruleIndex)
-
exitRule
public void exitRule()
-
enterOuterAlt
public void enterOuterAlt(ParserRuleContext localctx,
int altNum)
-
getPrecedence
public final int getPrecedence()
Get the precedence level for the top-most precedence rule.
- Returns:
- The precedence level for the top-most precedence rule, or -1 if
the parser context is not nested within a precedence rule.
-
enterRecursionRule
@Deprecated
public void enterRecursionRule(ParserRuleContext localctx,
int ruleIndex)
Deprecated. Use
enterRecursionRule(ParserRuleContext, int, int, int)
instead.
-
enterRecursionRule
public void enterRecursionRule(ParserRuleContext localctx,
int state,
int ruleIndex,
int precedence)
-
pushNewRecursionContext
public void pushNewRecursionContext(ParserRuleContext localctx,
int state,
int ruleIndex)
Like enterRule(org.antlr.v4.runtime.ParserRuleContext, int, int)
but for recursive rules.
Make the current context the child of the incoming localctx.
-
unrollRecursionContexts
public void unrollRecursionContexts(ParserRuleContext _parentctx)
-
getInvokingContext
public ParserRuleContext getInvokingContext(int ruleIndex)
-
getContext
public ParserRuleContext getContext()
-
setContext
public void setContext(ParserRuleContext ctx)
-
precpred
public boolean precpred(@Nullable
RuleContext localctx,
int precedence)
- Overrides:
precpred
in class Recognizer<Token,ParserATNSimulator>
-
getErrorListenerDispatch
public ParserErrorListener getErrorListenerDispatch()
- Overrides:
getErrorListenerDispatch
in class Recognizer<Token,ParserATNSimulator>
-
inContext
public boolean inContext(String context)
-
isExpectedToken
public boolean isExpectedToken(int symbol)
Checks whether or not symbol
can follow the current state in the
ATN. The behavior of this method is equivalent to the following, but is
implemented such that the complete context-sensitive follow set does not
need to be explicitly constructed.
return getExpectedTokens().contains(symbol);
- Parameters:
symbol
- the symbol type to check
- Returns:
true
if symbol
can follow the current state in
the ATN, otherwise false
.
-
isMatchedEOF
public boolean isMatchedEOF()
-
getExpectedTokens
@NotNull
public IntervalSet getExpectedTokens()
Computes the set of input symbols which could follow the current parser
state and context, as given by Recognizer.getState()
and getContext()
,
respectively.
- See Also:
ATN.getExpectedTokens(int, RuleContext)
-
getExpectedTokensWithinCurrentRule
@NotNull
public IntervalSet getExpectedTokensWithinCurrentRule()
-
getRuleIndex
public int getRuleIndex(String ruleName)
Get a rule's index (i.e., RULE_ruleName
field) or -1 if not found.
-
getRuleContext
public ParserRuleContext getRuleContext()
-
getRuleInvocationStack
public List<String> getRuleInvocationStack()
Return List<String> of the rule names in your parser instance
leading up to a call to the current rule. You could override if
you want more details such as the file/line info of where
in the ATN a rule is invoked.
This is very useful for error messages.
-
getRuleInvocationStack
public List<String> getRuleInvocationStack(RuleContext p)
-
dumpDFA
public void dumpDFA()
For debugging and other purposes.
-
getSourceName
public String getSourceName()
-
getParseInfo
public ParseInfo getParseInfo()
Description copied from class: Recognizer
If profiling during the parse/lex, this will return DecisionInfo records
for each decision in recognizer in a ParseInfo object.
- Overrides:
getParseInfo
in class Recognizer<Token,ParserATNSimulator>
-
setProfile
public void setProfile(boolean profile)
- Since:
- 4.3
-
setTrace
public void setTrace(boolean trace)
During a parse is sometimes useful to listen in on the rule entry and exit
events as well as token matches. This is for quick and dirty debugging.
-
isTrace
public boolean isTrace()
Gets whether a Parser.TraceListener
is registered as a parse listener
for the parser.
- See Also:
setTrace(boolean)
Copyright © 1992–2023 Daniel Sun. All rights reserved.