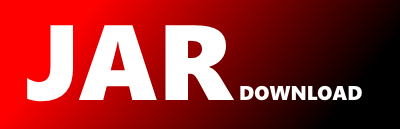
me.xhsun.guildwars2wrapper.GuildWars2API Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gw2wrapper Show documentation
Show all versions of gw2wrapper Show documentation
Guild Wars 2 API wrapper for Android
package me.xhsun.guildwars2wrapper;
import me.xhsun.guildwars2wrapper.model.*;
import me.xhsun.guildwars2wrapper.model.account.*;
import me.xhsun.guildwars2wrapper.model.achievements.Achievement;
import me.xhsun.guildwars2wrapper.model.character.CharacterInventory;
import me.xhsun.guildwars2wrapper.model.character.Core;
import me.xhsun.guildwars2wrapper.model.commerce.Prices;
import me.xhsun.guildwars2wrapper.model.commerce.Transaction;
import me.xhsun.guildwars2wrapper.model.guild.Upgrade;
import me.xhsun.guildwars2wrapper.model.pvp.Hero;
import me.xhsun.guildwars2wrapper.model.unlockable.Finisher;
import me.xhsun.guildwars2wrapper.model.unlockable.Glider;
import me.xhsun.guildwars2wrapper.model.unlockable.MailCarrier;
import me.xhsun.guildwars2wrapper.model.unlockable.Outfit;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Path;
import retrofit2.http.Query;
import java.util.List;
/**
* API interface for different calls
*
* @author xhsun
* @since 2017-02-07
*/
interface GuildWars2API {
//token info
@GET("/v2/tokeninfo")
Call getAPIInfo(@Query("access_token") String token);
//accounts
@GET("/v2/account")
Call getAccount(@Query("access_token") String token);
@GET("/v2/account/achievements")
Call> getAchievementProgression(@Query("access_token") String token);
@GET("/v2/account/bank")
Call> getBank(@Query("access_token") String token);
@GET("/v2/account/dungeons")
Call> getDailyDungeonProgression(@Query("access_token") String token);
@GET("/v2/account/dyes")
Call> getUnlockedDyes(@Query("access_token") String token);
@GET("/v2/account/finishers")
Call> getUnlockedFinishers(@Query("access_token") String token);
@GET("/v2/account/gliders")
Call> getUnlockedGliders(@Query("access_token") String token);
@GET("/v2/account/home/cats")
Call> getUnlockedCats(@Query("access_token") String token);
@GET("/v2/account/home/nodes")
Call> getUnlockedHomeNodes(@Query("access_token") String token);
@GET("/v2/account/inventory")
Call> getSharedInventory(@Query("access_token") String token);
@GET("/v2/account/mailcarriers")
Call> getUnlockedMailCarriers(@Query("access_token") String token);
@GET("/v2/account/masteries")
Call> getUnlockedMasteries(@Query("access_token") String token);
@GET("/v2/account/materials")
Call> getMaterialBank(@Query("access_token") String token);
@GET("/v2/account/minis")
Call> getUnlockedMinis(@Query("access_token") String token);
@GET("/v2/account/outfits")
Call> getUnlockedOutfits(@Query("access_token") String token);
@GET("/v2/account/pvp/heroes")
Call> getUnlockedPvpHeroes(@Query("access_token") String token);
@GET("/v2/account/raids")
Call> getWeeklyRaidProgression(@Query("access_token") String token);
@GET("/v2/account/recipes")
Call> getUnlockedRecipes(@Query("access_token") String token);
@GET("/v2/account/skins")
Call> getUnlockedSkins(@Query("access_token") String token);
@GET("/v2/account/titles")
Call> getUnlockedTitles(@Query("access_token") String token);
@GET("/v2/account/wallet")
Call> getWallet(@Query("access_token") String token);
//achievements
@GET("/v2/achievements")
Call> getAllAchievementIDs();
@GET("/v2/achievements")
Call> getAchievementInfo(@Query("ids") String ids);
//characters
@GET("/v2/characters")
Call> getAllCharacterName(@Query("access_token") String token);
@GET("/v2/characters/{name}/core")
Call getCharacterCore(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/inventory")
Call getCharacterInventory(@Path("name") String name, @Query("access_token") String token);
//colors
@GET("/v2/colors")
Call> getAllColorIDs();
@GET("/v2/colors")
Call> getColorInfo(@Query("ids") String ids);
//TP
@GET("/v2/commerce/transactions/{time}/{type}")
Call> getListing(@Path("time") String time, @Path("type") String type, @Query("access_token") String token);
@GET("/v2/commerce/prices")
Call> getAllPrices();
@GET("/v2/commerce/prices")
Call> getPrices(@Query("ids") String ids);
//currencies
@GET("/v2/currencies")
Call> getAllCurrencies();
@GET("/v2/currencies")
Call> getCurrencyInfo(@Query("ids") String ids);
//dungeons
@GET("/v2/dungeons")
Call> getAllDungeonName();
@GET("/v2/dungeons")
Call> getDungeonInfo(@Query("ids") String ids);
//Finishers
@GET("/v2/finishers")
Call> getAllFinisherIDs();
@GET("/v2/finishers")
Call> getFinisherInfo(@Query("ids") String ids);
//Gliders
@GET("/v2/gliders")
Call> getAllGliderIDs();
@GET("/v2/gliders")
Call> getGliderInfo(@Query("ids") String ids);
//Guild Upgrades
@GET("/v2/guild/upgrades")
Call> getAllGuildUpgradeIDs();
@GET("/v2/guild/upgrades")
Call> getGuildUpgradeInfo(@Query("ids") String ids);
//items
@GET("/v2/items")
Call> getAllItemIDs();
@GET("/v2/items")
Call> getItemInfo(@Query("ids") String ids);
//item stat
@GET("/v2/itemstats")
Call> getAllItemStatIDs();
@GET("/v2/itemstats")
Call> getItemStatInfo(@Query("ids") String ids);
//mail carriers
@GET("/v2/mailcarriers")
Call> getAllMailCarrierIDs();
@GET("/v2/mailcarriers")
Call> getMailCarrierInfo(@Query("ids") String ids);
//masteries
@GET("/v2/masteries")
Call> getAllMasteryIDs();
@GET("/v2/masteries")
Call> getMasteryInfo(@Query("ids") String ids);
//material categories
@GET("/v2/materials")
Call> getAllMaterialBankIDs();
@GET("/v2/materials")
Call> getMaterialBankInfo(@Query("ids") String ids);
//minis
@GET("/v2/minis")
Call> getAllMiniIDs();
@GET("/v2/minis")
Call> getMiniInfo(@Query("ids") String ids);
//Outfits
@GET("/v2/outfits")
Call> getAllOutfitIDs();
@GET("/v2/outfits")
Call> getOutfitInfo(@Query("ids") String ids);
//PvP Heroes
@GET("/v2/pvp/heroes")
Call> getAllPvPHeroIDs();
@GET("/v2/pvp/heroes")
Call> getPvPHeroInfo(@Query("ids") String ids);
//Raids
@GET("/v2/raids")
Call> getAllRaidIDs();
@GET("/v2/raids")
Call> getRaidInfo(@Query("ids") String ids);
//recipes
@GET("/v2/recipes")
Call> getAllRecipeIDs();
@GET("/v2/recipes")
Call> getRecipeInfo(@Query("ids") String ids);
//recipes search
@GET("/v2/recipes/search")
Call> searchInputRecipes(@Query("input") String id);
@GET("/v2/recipes/search")
Call> searchOutputRecipes(@Query("output") String id);
//skins
@GET("/v2/skins")
Call> getAllSkinIDs();
@GET("/v2/skins")
Call> getSkinInfo(@Query("ids") String ids);
//Titles
@GET("/v2/titles")
Call> getAllTitleIDs();
@GET("/v2/titles")
Call> getTitleInfo(@Query("ids") String ids);
//worlds
@GET("/v2/worlds")
Call> getAllWorldsIDs();
@GET("/v2/worlds")
Call> getWorldsInfo(@Query("ids") String ids);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy