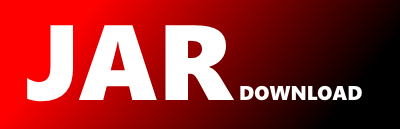
me.xhsun.guildwars2wrapper.SynchronousRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gw2wrapper Show documentation
Show all versions of gw2wrapper Show documentation
Guild Wars 2 API wrapper for Android
package me.xhsun.guildwars2wrapper;
import me.xhsun.guildwars2wrapper.error.ErrorCode;
import me.xhsun.guildwars2wrapper.error.GuildWars2Exception;
import me.xhsun.guildwars2wrapper.model.*;
import me.xhsun.guildwars2wrapper.model.account.*;
import me.xhsun.guildwars2wrapper.model.achievements.Achievement;
import me.xhsun.guildwars2wrapper.model.character.CharacterInventory;
import me.xhsun.guildwars2wrapper.model.character.Core;
import me.xhsun.guildwars2wrapper.model.commerce.Prices;
import me.xhsun.guildwars2wrapper.model.commerce.Transaction;
import me.xhsun.guildwars2wrapper.model.guild.Upgrade;
import me.xhsun.guildwars2wrapper.model.pvp.Hero;
import me.xhsun.guildwars2wrapper.model.unlockable.Finisher;
import me.xhsun.guildwars2wrapper.model.unlockable.Glider;
import me.xhsun.guildwars2wrapper.model.unlockable.MailCarrier;
import me.xhsun.guildwars2wrapper.model.unlockable.Outfit;
import retrofit2.Response;
import java.io.IOException;
import java.util.List;
/**
* This class contains all the method for accessing data synchronously
*
* @author xhsun
* @since 2017-06-04
*/
public class SynchronousRequest extends Request {
SynchronousRequest(GuildWars2API gw2API) {
super(gw2API);
}
//Token info
/**
* For more info on TokenInfo API go here
* Get detailed info related to this API key from server
*
* @param API API key
* @return API info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see TokenInfo API info
*/
public TokenInfo getAPIInfo(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response response = gw2API.getAPIInfo(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Accounts
/**
* For more info on Account API go here
* Get detailed info for account link to given API key
*
* @param API API key
* @return account info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Account Account info
*/
public Account getAccount(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response response = gw2API.getAccount(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account API go here
* Get an account's progress towards all their achievements.
*
* @param API API key
* @return list of account achievement info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AchievementProgression account achievement info
*/
public List getAchievementProgression(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getAchievementProgression(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Bank API go here
* Get detailed info for bank linked to given API key
*
* @param API API key
* @return bank info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Bank Bank info
*/
public List getBank(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getBank(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Dungeon progression API go here
*
* @param API API key
* @return an array of strings representing dungeon path names completed since daily dungeon reset
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getDailyDungeonProgression(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getDailyDungeonProgression(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account dyes API go here
* Get list of unlocked dyes ids linked to given API key
*
* @param API API key
* @return list of color ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color
*/
public List getUnlockedDyes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedDyes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account finishers API go here
* Get list of unlocked finishers linked to given API key
*
* @param API API key
* @return list of unlocked finisher info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see UnlockedFinisher unlocked finisher info
*/
public List getUnlockedFinishers(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedFinishers(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account gliders API go here
* Get list of unlocked glider id(s) linked to given API key
*
* @param API API key
* @return list of gliders id
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedGliders(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedGliders(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account cats API go here
* Get list of unlocked cats linked to given API key
*
* @param API API key
* @return list of cats
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Cat cat info
*/
public List getUnlockedCats(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedCats(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account nodes API go here
* Get list of unlocked home nodes linked to given API key
*
* @param API API key
* @return list of strings
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedHomeNodes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedHomeNodes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Shared Inventory API go here
* Get detailed info for shared inventory linked to given API key
*
* @param API API key
* @return share inventory info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see SharedInventory shared inventory info
*/
public List getSharedInventory(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getSharedInventory(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account mail carrier API go here
* Get list of unlocked mail carrier id(s) linked to given API key
*
* @param API API key
* @return list of mail carrier id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedMailCarriers(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedMailCarriers(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account masteries API go here
* Get list of unlocked masteries linked to given API key
*
* @param API API key
* @return list of unlocked masteries
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see UnlockedMastery unlocked mastery info
*/
public List getUnlockedMasteries(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedMasteries(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Material Storage API go here
* Get detailed info for material storage linked to given API key
*
* @param API API key
* @return material storage info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Material material storage info
*/
public List getMaterialStorage(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getMaterialBank(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account minis API go here
* Get list of unlocked mini id(s) linked to given API key
*
* @param API API key
* @return list of mini id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Mini mini info
*/
public List getUnlockedMinis(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedMinis(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account outfits API go here
* Get list of unlocked outfit id(s) linked to given API key
*
* @param API API key
* @return list of outfit id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedOutfits(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedOutfits(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account pvp heroes API go here
* Get list of unlocked pvp hero id(s) linked to given API key
*
* @param API API key
* @return list of pvp heroes id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedPvpHeroes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedPvpHeroes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account raid API go here
* Get list of cleared raid linked to given API key
*
* @param API API key
* @return list of cleared raid
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getWeeklyRaidProgression(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getWeeklyRaidProgression(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account recipes API go here
* Get list of unlocked recipe id(s) linked to given API key
*
* @param API API key
* @return list of unlocked recipe id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Recipe recipe info
*/
public List getUnlockedRecipes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedRecipes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account/Skins API go here
* Get list of unlocked skin ids linked to given API key
*
* @param API API key
* @return list of ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Skin skin info
*/
public List getUnlockedSkins(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedSkins(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account titles API go here
* Get list of unlocked title id(s) linked to given API key
*
* @param API API key
* @return list of unlocked title id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedTitles(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedTitles(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Wallet API go here
* Get detailed info for wallet linked to given API key
*
* @param API API key
* @return wallet info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Wallet wallet info
*/
public List getWallet(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getWallet(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Achievements
/**
* For more info on achievement API go here
* Get list of all available achievement id(s)
*
* @return list of achievement id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Achievement achievement info
*/
public List getAllAchievementID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllAchievementIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievement API go here
* Get list of achievement info corresponding to the given id(s)
*
* @param ids list of achievement id(s)
* @return list of achievement info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Achievement achievement info
*/
public List getAchievementInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getAchievementInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Characters
/**
* For more info on Character API go here
* Get all character names linked to given API key
*
* @param API API key
* @return list of character name
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getAllCharacterName(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getAllCharacterName(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Core API go here
* Get character information for the given character name that is linked to given API key
*
* @param API API key
* @param name name of character
* @return character detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Core character info
*/
public Core getCharacterInformation(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterCore(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Inventory API go here
* Get inventory info for the given character that is linked to given API key
*
* @param API API key
* @param name name of character to get inventory info
* @return character inventory detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see me.xhsun.guildwars2wrapper.model.util.Bag character inventory info
*/
public CharacterInventory getCharacterInventory(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterInventory(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Colors
/**
* For more info on Color API go here
* Get list of all available color id(s)
*
* @return list of color id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color color info
*/
public List getAllColorID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllColorIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Color API go here
* Get color info for the given color id(s)
*
* @param ids array of color id
* @return list of color info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color color info
*/
public List getColorInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getColorInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//TP
/**
* For more info on Transaction API go here
* Get transaction info linked to given API key
*
* @param API API key
* @param time current | History
* @param type buy | sell
* @return list of transaction base on the selection, if there is nothing, return empty list
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Transaction transaction info
*/
public List getListing(String API, Transaction.Time time, Transaction.Type type) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getListing(processListingTime(time), processListingType(type), API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Listing Price API go here
* Get all id of items that is on the TP
*
* @return list of item ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getAllListedItemID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllPrices().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Listing Price API go here
* Get price info for the given item id(s)
*
* @param ids list of item id
* @return list of listing price for given id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Prices listing price info
*/
public List getPrices(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getPrices(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Currencies
/**
* For more info on Currency API go here
* Get all currency ids
*
* @return list of currency ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Currency currency info
*/
public List getAllCurrencyID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllCurrencies().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Currency API go here
* Get currency info for the given currency id(s)
*
* @param ids list of currency id
* @return list of currency info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Currency currency info
*/
public List getCurrencyInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getCurrencyInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Dungeons
/**
* For more info on Dungeons API go here
* Get all dungeon name(s)
*
* @return list of dungeon name(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Dungeon dungeon info
*/
public List getAllDungeonName() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllDungeonName().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Dungeons API go here
* Get dungeon info for the given dungeon id(s)
*
* @param ids list of dungeon id
* @return list of dungeon info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Dungeon dungeon info
*/
public List getDungeonInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getDungeonInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Finishers
/**
* For more info on Finishers API go here
* Get all finisher id(s)
*
* @return list of finisher id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Finisher finisher info
*/
public List getAllFinisherID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllFinisherIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Finishers API go here
* Get finisher info for the given finisher id(s)
*
* @param ids list of finisher id
* @return list of finisher info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Finisher finisher info
*/
public List getFinisherInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getFinisherInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Glider
/**
* For more info on gliders API go here
* Get all glider id(s)
*
* @return list of glider id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Glider glider info
*/
public List getAllGliderID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllGliderIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on gliders API go here
* Get glider info for the given glider id(s)
*
* @param ids list of glider id
* @return list of glider info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Glider glider info
*/
public List getGliderInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getGliderInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Upgrades
/**
* For more info on guild upgrades API go here
* Get all guild upgrade id(s)
*
* @return list of guild upgrade id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Upgrade guild upgrade info
*/
public List getAllGuildUpgradeID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllGuildUpgradeIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on guild upgrades API go here
* Get guild upgrade info for the given guild upgrade id(s)
*
* @param ids list of guild upgrade id
* @return list of guild upgrade info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Upgrade guild upgrade info
*/
public List getGuildUpgradeInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getGuildUpgradeInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Items
/**
* For more info on Item API go here
* Get all available item ids
*
* @return list of item ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Item item info
*/
public List getAllItemID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllItemIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Item API go here
* Get item info for the given item id(s)
*
* @param ids list of item id
* @return list of item info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Item item info
*/
public List- getItemInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response
> response = gw2API.getItemInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Item Stats
/**
* For more info on Itemstat API go here
* Get all available itemstat ids
*
* @return list of itemstat ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ItemStats itemstat info
*/
public List getAllItemStatID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllItemStatIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Itemstat API go here
* Get itemstat info for the given itemstat id(s)
*
* @param ids list of itemstat id
* @return list of itemstat info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ItemStats itemstat info
*/
public List getItemStatInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getItemStatInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Mail Carriers
/**
* For more info on mail carriers API go here
* Get all mail carrier id(s)
*
* @return list of mail carrier info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see MailCarrier mail carrier info
*/
public List getAllMailCarrierID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllMailCarrierIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on mail carriers API go here
* Get mail carrier info for the given mail carrier id(s)
*
* @param ids list of mail carrier id
* @return list of mail carrier info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see MailCarrier mail carrier info
*/
public List getMailCarrierInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getMailCarrierInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Masteries
/**
* For more info on masteries API go here
* Get all mastery id(s)
*
* @return list of mastery id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Mastery mastery info
*/
public List getAllMasteryID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllMasteryIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on masteries API go here
* Get mastery info for the given mastery id(s)
*
* @param ids list of mastery id
* @return list of mastery info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Mastery mastery info
*/
public List getMasteryInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getMasteryInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Material Categories
/**
* For more info on Material Category API go here
* Get list of all available material category ids
*
* @return list of material category id
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see MaterialCategory material category info
*/
public List getAllMaterialCategoryID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllMaterialBankIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Material Category API go here
* Get material category info for the given category id(s)
*
* @param ids list of category id
* @return list of material category info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see MaterialCategory material category info
*/
public List getMaterialCategoryInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getMaterialBankInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Minis
/**
* For more info on Mini API go here
* Get list of all available mini id(s)
*
* @return list of mini id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Mini mini info
*/
public List getAllMiniID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllMiniIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Mini API go here
* Get mini info for the given mini id(s)
*
* @param ids list of mini id(s)
* @return list of mini info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Mini mini info
*/
public List getMiniInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getMiniInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Outfits
/**
* For more info on Outfits API go here
* Get all outfit id(s)
*
* @return list of outfit id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Outfit outfit info
*/
public List getAllOutfitID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllOutfitIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Outfits API go here
* Get outfit info for the given outfit id(s)
*
* @param ids list of outfit id(s)
* @return list of outfit info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Outfit outfit info
*/
public List getOutfitInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getOutfitInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//PvP Heroes
/**
* For more info on pvp heroes API go here
* Get all pvp hero id(s)
*
* @return list of pvp hero id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Hero pvp hero info
*/
public List getAllPvPHeroID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllPvPHeroIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on pvp heroes API go here
* Get pvp hero info for the given pvp hero id(s)
*
* @param ids list of pvp hero id(s)
* @return list of pvp hero info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Hero pvp hero info
*/
public List getPvPHeroInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getPvPHeroInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Raids
/**
* For more info on raids API go here
* Get all raid id(s)
*
* @return list of raid id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Raid raid info
*/
public List getAllRaidID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllRaidIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on raids API go here
* Get raid info for the given raid id(s)
*
* @param ids list of raid id(s)
* @return list of raid info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Raid raid info
*/
public List getRaidInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getRaidInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Recipes
/**
* For more info on Recipes API go here
* Get list of all available recipe id(s)
*
* @return list of recipe ids(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Recipe recipe info
*/
public List getAllRecipeID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllRecipeIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Recipes API go here
* Get recipe info for the given recipe id(s)
*
* @param ids array of recipe id
* @return list of recipe info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Recipe recipe info
*/
public List getRecipeInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getRecipeInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Recipes Search
/**
* For more info on Recipes search API go here
*
* @param isInput is given id an ingredient
* @param id recipe id
* @return list of recipe id
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List searchRecipes(boolean isInput, int id) throws GuildWars2Exception {
try {
Response> response = (isInput) ?
gw2API.searchInputRecipes(Integer.toString(id)).execute() :
gw2API.searchOutputRecipes(Integer.toString(id)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Skins
/**
* For more info on Skin API go here
* Get list of all available skin ids
*
* @return list of skin ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Skin skin info
*/
public List getAllSkinID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllSkinIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Skin API go here
* Get skin info for the given skin id(s)
*
* @param ids list of skin id
* @return list of skin info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Skin skin info
*/
public List getSkinInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getSkinInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Titles
/**
* For more info on titles API go here
* Get all title id(s)
*
* @return list of title id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Title title info
*/
public List getAllTitleID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllTitleIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on titles API go here
* Get title info for the given title id(s)
*
* @param ids list of title id(s)
* @return list of title info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Title title info
*/
public List getTitleInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getTitleInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Worlds
/**
* For more info on World API go here
* Get list of all available world id(s)
*
* @return list of world id
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see World world info
*/
public List getAllWorldID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllWorldsIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on World API go here
* Get world info for the given world id(s)
*
* @param ids list of world id
* @return list of world info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see World world info
*/
public List getWorldInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getWorldsInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy