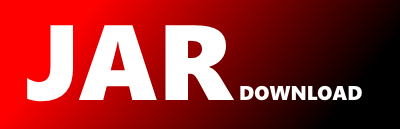
me.xhsun.guildwars2wrapper.GuildWars2API Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gw2wrapper Show documentation
Show all versions of gw2wrapper Show documentation
Guild Wars 2 API wrapper for Android
package me.xhsun.guildwars2wrapper;
import me.xhsun.guildwars2wrapper.model.v1.AllWvWMatchOverview;
import me.xhsun.guildwars2wrapper.model.v1.EventDetail;
import me.xhsun.guildwars2wrapper.model.v1.SimpleName;
import me.xhsun.guildwars2wrapper.model.v2.*;
import me.xhsun.guildwars2wrapper.model.v2.account.*;
import me.xhsun.guildwars2wrapper.model.v2.achievement.Achievement;
import me.xhsun.guildwars2wrapper.model.v2.achievement.AchievementCategory;
import me.xhsun.guildwars2wrapper.model.v2.achievement.AchievementGroup;
import me.xhsun.guildwars2wrapper.model.v2.achievement.DailyAchievement;
import me.xhsun.guildwars2wrapper.model.v2.backstory.BackStoryAnswer;
import me.xhsun.guildwars2wrapper.model.v2.backstory.BackStoryQuestion;
import me.xhsun.guildwars2wrapper.model.v2.character.Character;
import me.xhsun.guildwars2wrapper.model.v2.character.*;
import me.xhsun.guildwars2wrapper.model.v2.commerce.*;
import me.xhsun.guildwars2wrapper.model.v2.continent.Continent;
import me.xhsun.guildwars2wrapper.model.v2.continent.ContinentFloor;
import me.xhsun.guildwars2wrapper.model.v2.continent.ContinentMap;
import me.xhsun.guildwars2wrapper.model.v2.continent.ContinentRegion;
import me.xhsun.guildwars2wrapper.model.v2.guild.*;
import me.xhsun.guildwars2wrapper.model.v2.pvp.*;
import me.xhsun.guildwars2wrapper.model.v2.story.Story;
import me.xhsun.guildwars2wrapper.model.v2.story.StorySeason;
import me.xhsun.guildwars2wrapper.model.v2.util.Inventory;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWAbility;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWObjective;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWRank;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWUpgrade;
import me.xhsun.guildwars2wrapper.model.v2.wvw.matches.WvWMatchDetail;
import me.xhsun.guildwars2wrapper.model.v2.wvw.matches.WvWMatchOverview;
import me.xhsun.guildwars2wrapper.model.v2.wvw.matches.WvWMatchScore;
import me.xhsun.guildwars2wrapper.model.v2.wvw.matches.WvWMatchStat;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Path;
import retrofit2.http.Query;
import java.util.List;
import java.util.Map;
/**
* API interface for different calls
*
* @author xhsun
* @since 2017-02-07
*/
interface GuildWars2API {
//API:1
//Event Detail (lang)
@GET("/v1/event_details.json")
Call getAllEventDetailedInfo();
@GET("/v1/event_details.json")
Call getEventDetailedInfo(@Query("event_id") String id);
//Map Names (lang)
@GET("/v1/map_names.json")
Call> getAllMapNames();
//World Names (lang)
@GET("/v1/world_names.json")
Call> getAllWorldNames();
//WvW Matches
@GET("/v1/wvw/matches.json")
Call getAllWvWMatchOverview();
//WvW Objective Names (lang)
@GET("/v1/wvw/objective_names.json")
Call> getAllWvWObjectiveNames();
//API:2
//token info
@GET("/v2/tokeninfo")
Call getAPIInfo(@Query("access_token") String token);
//accounts
@GET("/v2/account")
Call getAccount(@Query("access_token") String token);
@GET("/v2/account/achievement")
Call> getAchievementProgression(@Query("access_token") String token);
@GET("/v2/account/bank")
Call> getBank(@Query("access_token") String token);
@GET("/v2/account/dungeons")
Call> getDailyDungeonProgression(@Query("access_token") String token);
@GET("/v2/account/dyes")
Call> getUnlockedDyes(@Query("access_token") String token);
@GET("/v2/account/finishers")
Call> getUnlockedFinishers(@Query("access_token") String token);
@GET("/v2/account/gliders")
Call> getUnlockedGliders(@Query("access_token") String token);
@GET("/v2/account/home/cats")
Call> getUnlockedCats(@Query("access_token") String token);
@GET("/v2/account/home/nodes")
Call> getUnlockedHomeNodes(@Query("access_token") String token);
@GET("/v2/account/inventory")
Call> getSharedInventory(@Query("access_token") String token);
@GET("/v2/account/mailcarriers")
Call> getUnlockedMailCarriers(@Query("access_token") String token);
@GET("/v2/account/masteries")
Call> getUnlockedMasteries(@Query("access_token") String token);
@GET("/v2/account/materials")
Call> getMaterialBank(@Query("access_token") String token);
@GET("/v2/account/minis")
Call> getUnlockedMinis(@Query("access_token") String token);
@GET("/v2/account/outfits")
Call> getUnlockedOutfits(@Query("access_token") String token);
@GET("/v2/account/pvp/heroes")
Call> getUnlockedPvpHeroes(@Query("access_token") String token);
@GET("/v2/account/raids")
Call> getWeeklyRaidProgression(@Query("access_token") String token);
@GET("/v2/account/recipes")
Call> getUnlockedRecipes(@Query("access_token") String token);
@GET("/v2/account/skins")
Call> getUnlockedSkins(@Query("access_token") String token);
@GET("/v2/account/titles")
Call> getUnlockedTitles(@Query("access_token") String token);
@GET("/v2/account/wallet")
Call> getWallet(@Query("access_token") String token);
//achievement
@GET("/v2/achievements")
Call> getAllAchievementIDs();
@GET("/v2/achievements")
Call> getAchievementInfo(@Query("ids") String ids);
@GET("/v2/achievements/categories")
Call> getAllAchievementCategoryIDs();
@GET("/v2/achievements/categories")
Call> getAchievementCategoryInfo(@Query("ids") String ids);
@GET("/v2/achievements/daily")
Call getCurrentDailyAchievements();
@GET("/v2/achievements/daily/tomorrow")
Call getNextDailyAchievements();
@GET("/v2/achievements/groups")
Call> getAllAchievementGroupIDs();
@GET("/v2/achievements/groups")
Call> getAchievementGroupInfo(@Query("ids") String ids);
//back story
@GET("/v2/backstory/answers")
Call> getAllBackStoryAnswerIDs();
@GET("/v2/backstory/answers")
Call> getBackStoryAnswerInfo(@Query("ids") String ids);
@GET("/v2/backstory/questions")
Call> getAllBackStoryQuestionIDs();
@GET("/v2/backstory/questions")
Call> getBackStoryQuestionInfo(@Query("ids") String ids);
//Current PvPGame Build
@GET("/v2/build")
Call getCurrentGameBuild();
//Cats
@GET("/v2/cats")
Call> getAllCatIDs();
@GET("/v2/cats")
Call> getCatInfo(@Query("ids") String ids);
//characters
@GET("/v2/characters")
Call> getAllCharacterName(@Query("access_token") String token);
@GET("/v2/characters/{name}")
Call getCharacter(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/backstory")
Call getCharacterBackStory(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/core")
Call getCharacterCore(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/crafting")
Call getCharacterCrafting(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/equipment")
Call getCharacterEquipment(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/heropoints")
Call> getCharacterHeroPoints(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/inventory")
Call getCharacterInventory(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/recipes")
Call getCharacterUnlockedRecipes(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/sab")
Call getCharacterSAB(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/skills")
Call getCharacterSkills(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/specializations")
Call getCharacterSpecialization(@Path("name") String name, @Query("access_token") String token);
@GET("/v2/characters/{name}/training")
Call getCharacterTraining(@Path("name") String name, @Query("access_token") String token);
//colors
@GET("/v2/colors")
Call> getAllColorIDs();
@GET("/v2/colors")
Call> getColorInfo(@Query("ids") String ids);
//TP
@GET("/v2/commerce/delivery")
Call> getTPDeliveryInfo(@Query("access_token") String token);
@GET("/v2/commerce/exchange")
Call> getAllExchangeCurrency();
@GET("/v2/commerce/exchange/{currency}")
Call getExchangeInfo(@Path("currency") String currency, @Query("quantity") String quantity);
@GET("/v2/commerce/listings")
Call> getAllTPListingIDs();
@GET("/v2/commerce/listings")
Call> getTPListingInfo(@Query("ids") String ids);
@GET("/v2/commerce/prices")
Call> getAllTPPriceIDs();
@GET("/v2/commerce/prices")
Call> getTPPriceInfo(@Query("ids") String ids);
@GET("/v2/commerce/transactions/{time}/{type}")
Call> getTPTransaction(@Path("time") String time, @Path("type") String type, @Query("access_token") String token);
//continents
@GET("/v2/continents")
Call> getAllContinentIDs();
@GET("/v2/continents")
Call> getContinentInfo(@Query("ids") String ids);
@GET("/v2/continents/{continentId}/floors")
Call> getAllContinentFloorIDs(@Path("continentId") String continent);
@GET("/v2/continents/{continentId}/floors")
Call> getContinentFloorInfo(@Path("continentId") String continent, @Query("ids") String ids);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions")
Call> getAllContinentRegionIDs(@Path("continentId") String continent, @Path("floorId") String floor);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions")
Call> getContinentRegionInfo(@Path("continentId") String continent, @Path("floorId") String floor, @Query("ids") String ids);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps")
Call> getAllContinentMapIDs(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps")
Call> getContinentMapInfo(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Query("ids") String ids);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps/{mapId}/sectors")
Call> getAllContinentSectorIDs(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Path("mapId") String map);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps/{mapId}/sectors")
Call> getContinentSectorInfo(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Path("mapId") String map, @Query("ids") String ids);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps/{mapId}/pois")
Call> getAllContinentPOIIDs(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Path("mapId") String map);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps/{mapId}/pois")
Call> getContinentPOIInfo(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Path("mapId") String map, @Query("ids") String ids);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps/{mapId}/tasks")
Call> getAllContinentTaskIDs(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Path("mapId") String map);
@GET("/v2/continents/{continentId}/floors/{floorId}/regions/{regionId}/maps/{mapId}/tasks")
Call> getContinentTaskInfo(@Path("continentId") String continent, @Path("floorId") String floor, @Path("regionId") String region, @Path("mapId") String map, @Query("ids") String ids);
//currencies
@GET("/v2/currencies")
Call> getAllCurrencies();
@GET("/v2/currencies")
Call> getCurrencyInfo(@Query("ids") String ids);
//dungeons
@GET("/v2/dungeons")
Call> getAllDungeonName();
@GET("/v2/dungeons")
Call> getDungeonInfo(@Query("ids") String ids);
//Emblem
@GET("/v2/emblem")
Call> getAllEmblemType();
@GET("/v2/emblem/{type}")
Call> getAllEmblemIDs(@Path("type") String type);
@GET("/v2/emblem/{type}")
Call> getAllEmblemInfo(@Path("type") String type, @Query("ids") String ids);
//Files
@GET("/v2/files")
Call> getAllFileIDs();
@GET("/v2/files")
Call> getAllFileInfo(@Query("ids") String ids);
//Finishers
@GET("/v2/finishers")
Call> getAllFinisherIDs();
@GET("/v2/finishers")
Call> getFinisherInfo(@Query("ids") String ids);
//Gliders
@GET("/v2/gliders")
Call> getAllGliderIDs();
@GET("/v2/gliders")
Call> getGliderInfo(@Query("ids") String ids);
//Guild
@GET("/v2/guild/{id}")
Call getGeneralGuildInfo(@Path("id") String id);
@GET("/v2/guild/{id}")
Call getDetailedGuildInfo(@Path("id") String id, @Query("access_token") String token);
//Guild Log
@GET("/v2/guild/{id}/log")
Call> getGuildLogInfo(@Path("id") String id, @Query("access_token") String token);
@GET("/v2/guild/{id}/log")
Call> getFilteredGuildLogInfo(@Path("id") String id, @Query("access_token") String token, @Query("since") String since);
//Guild Members
@GET("/v2/guild/{id}/members")
Call> getGuildMemberInfo(@Path("id") String id, @Query("access_token") String token);
//Guild Ranks
@GET("/v2/guild/{id}/ranks")
Call> getGuildRankInfo(@Path("id") String id, @Query("access_token") String token);
//Guild Stash
@GET("/v2/guild/{id}/stash")
Call> getGuildStashInfo(@Path("id") String id, @Query("access_token") String token);
//Guild Teams
@GET("/v2/guild/{id}/teams")
Call> getGuildTeamsInfo(@Path("id") String id, @Query("access_token") String token);
//Guild Treasury
@GET("/v2/guild/{id}/treasury")
Call> getGuildTreasuryInfo(@Path("id") String id, @Query("access_token") String token);
//Guild Unlocked Upgrades
@GET("/v2/guild/{id}/upgrades")
Call> getGuildUpgradeIDs(@Path("id") String id, @Query("access_token") String token);
//Guild Permissions
@GET("/v2/guild/permissions")
Call> getAllGuildPermissionIDs();
@GET("/v2/guild/permissions")
Call> getGuildPermissionInfo(@Query("ids") String ids);
//Guild Search
@GET("/v2/guild/search")
Call> searchGuildID(@Query("name") String name);
//Guild Upgrades
@GET("/v2/guild/upgrades")
Call> getAllGuildUpgradeIDs();
@GET("/v2/guild/upgrades")
Call> getGuildUpgradeInfo(@Query("ids") String ids);
//items
@GET("/v2/items")
Call> getAllItemIDs();
@GET("/v2/items")
Call> getItemInfo(@Query("ids") String ids);
//item stat
@GET("/v2/itemstats")
Call> getAllItemStatIDs();
@GET("/v2/itemstats")
Call> getItemStatInfo(@Query("ids") String ids);
//Legends
@GET("/v2/legends")
Call> getAllLegendIDs();
@GET("/v2/legends")
Call> getLegendInfo(@Query("ids") String ids);
//mail carriers
@GET("/v2/mailcarriers")
Call> getAllMailCarrierIDs();
@GET("/v2/mailcarriers")
Call> getMailCarrierInfo(@Query("ids") String ids);
//Map
@GET("/v2/maps")
Call> getAllMapIDs();
@GET("/v2/maps")
Call> getMapInfo(@Query("ids") String ids);
//masteries
@GET("/v2/masteries")
Call> getAllMasteryIDs();
@GET("/v2/masteries")
Call> getMasteryInfo(@Query("ids") String ids);
//material categories
@GET("/v2/materials")
Call> getAllMaterialBankIDs();
@GET("/v2/materials")
Call> getMaterialBankInfo(@Query("ids") String ids);
//minis
@GET("/v2/minis")
Call> getAllMiniIDs();
@GET("/v2/minis")
Call> getMiniInfo(@Query("ids") String ids);
//Nodes
@GET("/v2/nodes")
Call> getAllHomeInstanceNodeIDs();
//Outfits
@GET("/v2/outfits")
Call> getAllOutfitIDs();
@GET("/v2/outfits")
Call> getOutfitInfo(@Query("ids") String ids);
//Pets
@GET("/v2/pets")
Call> getAllPetIDs();
@GET("/v2/pets")
Call> getPetInfo(@Query("ids") String ids);
//Professions
@GET("/v2/professions")
Call> getAllProfessionIDs();
@GET("/v2/professions")
Call> getProfessionInfo(@Query("ids") String ids);
//PvP Amulets
@GET("/v2/pvp/amulets")
Call> getAllPvPAmuletIDs();
@GET("/v2/pvp/amulets")
Call> getPvPAmuletInfo(@Query("ids") String ids);
//PvP Games
@GET("/v2/pvp/games")
Call> getAllPvPGameIDs(@Query("access_token") String token);
@GET("/v2/pvp/games")
Call> getPvPGameInfo(@Query("access_token") String token, @Query("ids") String ids);
//PvP Heroes
@GET("/v2/pvp/heroes")
Call> getAllPvPHeroIDs();
@GET("/v2/pvp/heroes")
Call> getPvPHeroInfo(@Query("ids") String ids);
//PvP Ranks
@GET("/v2/pvp/ranks")
Call> getAllPvPRankIDs();
@GET("/v2/pvp/ranks")
Call> getPvPRankInfo(@Query("ids") String ids);
//PvP Seasons
@GET("/v2/pvp/seasons")
Call> getAllPvPSeasonIDs();
@GET("/v2/pvp/seasons")
Call> getPvPSeasonInfo(@Query("ids") String ids);
//PvP Seasons LeaderBoard
@GET("/v2/pvp/seasons/{id}/leaderboards/{type}/{region}")
Call> getPvPSeasonLeaderBoardInfo(@Path("id") String id, @Path("type") String type, @Path("region") String region);
//PvP Standings
@GET("/v2/pvp/standings")
Call> getPvPStandingInfo(@Query("access_token") String token);
//PvP Stats
@GET("/v2/pvp/stats")
Call getPvPStatInfo(@Query("access_token") String token);
//Quaggans
@GET("/v2/quaggans")
Call> getAllQuagganIDs();
@GET("/v2/quaggans")
Call>> getQuagganInfo(@Query("ids") String ids);
//Races
@GET("/v2/races")
Call> getAllRaceIDs();
@GET("/v2/races")
Call> getRaceInfo(@Query("ids") String ids);
//Raids
@GET("/v2/raids")
Call> getAllRaidIDs();
@GET("/v2/raids")
Call> getRaidInfo(@Query("ids") String ids);
//recipes
@GET("/v2/recipes")
Call> getAllRecipeIDs();
@GET("/v2/recipes")
Call> getRecipeInfo(@Query("ids") String ids);
//recipes search
@GET("/v2/recipes/search")
Call> searchInputRecipes(@Query("input") String id);
@GET("/v2/recipes/search")
Call> searchOutputRecipes(@Query("output") String id);
//Skills
@GET("/v2/skills")
Call> getAllSkillIDs();
@GET("/v2/skills")
Call> getSkillInfo(@Query("ids") String ids);
//skins
@GET("/v2/skins")
Call> getAllSkinIDs();
@GET("/v2/skins")
Call> getSkinInfo(@Query("ids") String ids);
//Specializations
@GET("/v2/specializations")
Call> getAllSpecializationIDs();
@GET("/v2/specializations")
Call> getSpecializationInfo(@Query("ids") String ids);
//Stories
@GET("/v2/stories")
Call> getAllStoryIDs();
@GET("/v2/stories")
Call> getStoryInfo(@Query("ids") String ids);
//Stories Seasons
@GET("/v2/stories/seasons")
Call> getAllStorySeasonIDs();
@GET("/v2/stories/seasons")
Call> getStorySeasonInfo(@Query("ids") String ids);
//Titles
@GET("/v2/titles")
Call> getAllTitleIDs();
@GET("/v2/titles")
Call> getTitleInfo(@Query("ids") String ids);
//Traits
@GET("/v2/traits")
Call> getAllTraitIDs();
@GET("/v2/traits")
Call> getTraitInfo(@Query("ids") String ids);
//worlds
@GET("/v2/worlds")
Call> getAllWorldsIDs();
@GET("/v2/worlds")
Call> getWorldsInfo(@Query("ids") String ids);
//WvW Abilities
@GET("/v2/wvw/abilities")
Call> getAllWvWAbilityIDs();
@GET("/v2/wvw/abilities")
Call> getWvWAbilityInfo(@Query("ids") String ids);
//WvW Matches
@GET("/v2/wvw/matches")
Call> getAllWvWMatchIDs();
@GET("/v2/wvw/matches")
Call getWvWMatchInfoUsingWorld(@Query("world") String world);
@GET("/v2/wvw/matches/overview")
Call getWvWMatchOverviewUsingWorld(@Query("world") String world);
@GET("/v2/wvw/matches/scores")
Call getWvWMatchScoreUsingWorld(@Query("world") String world);
@GET("/v2/wvw/matches/stats")
Call getWvWMatchStatUsingWorld(@Query("world") String world);
@GET("/v2/wvw/matches")
Call> getWvWMatchInfoUsingID(@Query("ids") String ids);
@GET("/v2/wvw/matches/overview")
Call> getWvWMatchOverviewUsingID(@Query("ids") String ids);
@GET("/v2/wvw/matches/scores")
Call> getWvWMatchScoreUsingID(@Query("ids") String ids);
@GET("/v2/wvw/matches/stats")
Call> getWvWMatchStatUsingID(@Query("ids") String ids);
//WvW Teams
// TODO this endpoint isn't returning anything, disabled for now
// @GET("/v2/wvw/matches/stats/{id}/teams/{side}/top/{type}")
// Call> getWvWMatchTeamInfo(@Path("id") String match_id, @Path("side") String side, @Path("type") String type);
//WvW Objectives
@GET("/v2/wvw/objectives")
Call> getAllWvWObjectiveIDs();
@GET("/v2/wvw/objectives")
Call> getWvWObjectiveInfo(@Query("ids") String ids);
//WvW Ranks
@GET("/v2/wvw/ranks")
Call> getAllWvWRankIDs();
@GET("/v2/wvw/ranks")
Call> getWvWRankInfo(@Query("ids") String ids);
//WvW Upgrades
@GET("/v2/wvw/upgrades")
Call> getAllWvWUpgradeIDs();
@GET("/v2/wvw/upgrades")
Call> getWvWUpgradeInfo(@Query("ids") String ids);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy