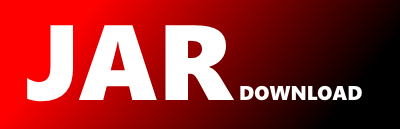
me.xhsun.guildwars2wrapper.SynchronousRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gw2wrapper Show documentation
Show all versions of gw2wrapper Show documentation
Guild Wars 2 API wrapper for Android
package me.xhsun.guildwars2wrapper;
import me.xhsun.guildwars2wrapper.error.ErrorCode;
import me.xhsun.guildwars2wrapper.error.GuildWars2Exception;
import me.xhsun.guildwars2wrapper.model.v1.AllWvWMatchOverview;
import me.xhsun.guildwars2wrapper.model.v1.EventDetail;
import me.xhsun.guildwars2wrapper.model.v1.SimpleName;
import me.xhsun.guildwars2wrapper.model.v2.*;
import me.xhsun.guildwars2wrapper.model.v2.account.*;
import me.xhsun.guildwars2wrapper.model.v2.achievement.Achievement;
import me.xhsun.guildwars2wrapper.model.v2.achievement.AchievementCategory;
import me.xhsun.guildwars2wrapper.model.v2.achievement.AchievementGroup;
import me.xhsun.guildwars2wrapper.model.v2.achievement.DailyAchievement;
import me.xhsun.guildwars2wrapper.model.v2.backstory.BackStoryAnswer;
import me.xhsun.guildwars2wrapper.model.v2.backstory.BackStoryQuestion;
import me.xhsun.guildwars2wrapper.model.v2.character.Character;
import me.xhsun.guildwars2wrapper.model.v2.character.*;
import me.xhsun.guildwars2wrapper.model.v2.commerce.*;
import me.xhsun.guildwars2wrapper.model.v2.continent.Continent;
import me.xhsun.guildwars2wrapper.model.v2.continent.ContinentFloor;
import me.xhsun.guildwars2wrapper.model.v2.continent.ContinentMap;
import me.xhsun.guildwars2wrapper.model.v2.continent.ContinentRegion;
import me.xhsun.guildwars2wrapper.model.v2.guild.*;
import me.xhsun.guildwars2wrapper.model.v2.pvp.*;
import me.xhsun.guildwars2wrapper.model.v2.story.Story;
import me.xhsun.guildwars2wrapper.model.v2.story.StorySeason;
import me.xhsun.guildwars2wrapper.model.v2.util.Inventory;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWAbility;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWObjective;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWRank;
import me.xhsun.guildwars2wrapper.model.v2.wvw.WvWUpgrade;
import me.xhsun.guildwars2wrapper.model.v2.wvw.matches.*;
import retrofit2.Response;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* This class contains all the method for accessing data synchronously
*
* @author xhsun
* @since 2017-06-04
*/
public class SynchronousRequest extends Request {
SynchronousRequest(GuildWars2API gw2API) {
super(gw2API);
}
//API:1
//Event Detail
/**
* For more info on event detail API go here
*
* @return event details
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see EventDetail event detail
*/
public EventDetail getAllEventDetailedInfo() throws GuildWars2Exception {
try {
Response response = gw2API.getAllEventDetailedInfo().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on event detail API go here
*
* @param id event id
* @return event details
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see EventDetail event detail
*/
public EventDetail getEventDetailedInfo(String id) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.ID, id));
try {
Response response = gw2API.getEventDetailedInfo(id).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Map Names
/**
* For more info on map name API go here
*
* @return list of names
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see SimpleName map name
*/
public List getAllMapNames() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllMapNames().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//World Names
/**
* For more info on world name API go here
*
* @return list of names
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see SimpleName world name
*/
public List getAllWorldNames() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllWorldNames().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//WvW Matches
/**
* For more info on v1 wvw matches API go here
*
* @return simple wvw matches info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AllWvWMatchOverview wvw matches
*/
public AllWvWMatchOverview getAllWvWMatchOverview() throws GuildWars2Exception {
try {
Response response = gw2API.getAllWvWMatchOverview().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//WvW Objective Names
/**
* For more info on WvW objective API go here
*
* @return list of names
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see SimpleName objective name
*/
public List getAllWvWObjectiveNames() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllWvWObjectiveNames().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//API:2
//Token info
/**
* For more info on TokenInfo API go here
* Get detailed info related to this API key from server
*
* @param API API key
* @return API info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see TokenInfo API info
*/
public TokenInfo getAPIInfo(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response response = gw2API.getAPIInfo(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Accounts
/**
* For more info on Account API go here
* Get detailed info for account link to given API key
*
* @param API API key
* @return account info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Account Account info
*/
public Account getAccountInfo(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response response = gw2API.getAccount(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account API go here
* Get an account's progress towards all their achievements.
*
* @param API API key
* @return list of account achievement info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AchievementProgression account achievement info
*/
public List getAchievementProgression(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getAchievementProgression(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Bank API go here
* Get detailed info for bank linked to given API key
*
* @param API API key
* @return bank info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Inventory Bank info
*/
public List getBank(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getBank(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Dungeon progression API go here
*
* @param API API key
* @return an array of strings representing dungeon path names completed since daily dungeon reset
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getDailyDungeonProgression(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getDailyDungeonProgression(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account dyes API go here
* Get list of unlocked dyes ids linked to given API key
*
* @param API API key
* @return list of color ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color
*/
public List getUnlockedDyes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedDyes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account finishers API go here
* Get list of unlocked finishers linked to given API key
*
* @param API API key
* @return list of unlocked finisher info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see UnlockedFinisher unlocked finisher info
*/
public List getUnlockedFinishers(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedFinishers(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account gliders API go here
* Get list of unlocked glider id(s) linked to given API key
*
* @param API API key
* @return list of gliders id
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedGliders(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedGliders(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account cats API go here
* Get list of unlocked cats linked to given API key
*
* @param API API key
* @return list of cats
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Cat cat info
*/
public List getUnlockedCats(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedCats(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account nodes API go here
* Get list of unlocked home nodes linked to given API key
*
* @param API API key
* @return list of strings
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedHomeNodes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedHomeNodes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Shared Inventory API go here
* Get detailed info for shared inventory linked to given API key
*
* @param API API key
* @return share inventory info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Inventory shared inventory info
*/
public List getSharedInventory(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getSharedInventory(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account mail carrier API go here
* Get list of unlocked mail carrier id(s) linked to given API key
*
* @param API API key
* @return list of mail carrier id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedMailCarriers(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedMailCarriers(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account masteries API go here
* Get list of unlocked masteries linked to given API key
*
* @param API API key
* @return list of unlocked masteries
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see UnlockedMastery unlocked mastery info
*/
public List getUnlockedMasteries(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedMasteries(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Material Storage API go here
* Get detailed info for material storage linked to given API key
*
* @param API API key
* @return material storage info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see MaterialStorage material storage info
*/
public List getMaterialStorage(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getMaterialBank(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account minis API go here
* Get list of unlocked mini id(s) linked to given API key
*
* @param API API key
* @return list of mini id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Mini mini info
*/
public List getUnlockedMinis(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedMinis(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account outfits API go here
* Get list of unlocked outfit id(s) linked to given API key
*
* @param API API key
* @return list of outfit id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedOutfits(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedOutfits(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account pvp heroes API go here
* Get list of unlocked pvp hero id(s) linked to given API key
*
* @param API API key
* @return list of pvp heroes id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedPvpHeroes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedPvpHeroes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account raid API go here
* Get list of cleared raid linked to given API key
*
* @param API API key
* @return list of cleared raid
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getWeeklyRaidProgression(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getWeeklyRaidProgression(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on account recipes API go here
* Get list of unlocked recipe id(s) linked to given API key
*
* @param API API key
* @return list of unlocked recipe id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Recipe recipe info
*/
public List getUnlockedRecipes(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedRecipes(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account/Skins API go here
* Get list of unlocked skin ids linked to given API key
*
* @param API API key
* @return list of ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Skin skin info
*/
public List getUnlockedSkins(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedSkins(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Account titles API go here
* Get list of unlocked title id(s) linked to given API key
*
* @param API API key
* @return list of unlocked title id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getUnlockedTitles(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getUnlockedTitles(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Wallet API go here
* Get detailed info for wallet linked to given API key
*
* @param API API key
* @return wallet info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Wallet wallet info
*/
public List getWallet(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getWallet(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Achievements
/**
* For more info on achievement API go here
* Get list of all available achievement id(s)
*
* @return list of achievement id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Achievement achievement info
*/
public List getAllAchievementID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllAchievementIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievement API go here
* Get list of achievement info corresponding to the given id(s)
*
* @param ids list of achievement id(s)
* @return list of achievement info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Achievement achievement info
*/
public List getAchievementInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getAchievementInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievements categories API go here
* Get list of all available achievement categories id(s)
*
* @return list of achievement categories id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AchievementCategory achievement categories info
*/
public List getAllAchievementCategoryID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllAchievementCategoryIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievements categories API go here
* Get list of achievement categories info corresponding to the given id(s)
*
* @param ids list of achievement categories id(s)
* @return list of achievement categories info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AchievementCategory achievement categories info
*/
public List getAchievementCategoryInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getAchievementCategoryInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievements daily API go here
* Get list of current daily achievements
*
* @return list of current daily achievements
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see DailyAchievement daily achievement info
*/
public DailyAchievement getCurrentDailyAchievements() throws GuildWars2Exception {
try {
Response response = gw2API.getCurrentDailyAchievements().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on next achievements daily API go here
* Get list of tomorrow's daily achievements
*
* @return list of tomorrow's daily achievements
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see DailyAchievement daily achievement info
*/
public DailyAchievement getNextDailyAchievements() throws GuildWars2Exception {
try {
Response response = gw2API.getNextDailyAchievements().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievements groups API go here
* Get list of all available achievement group id(s)
*
* @return list of achievement group id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AchievementGroup achievement group info
*/
public List getAllAchievementGroupID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllAchievementGroupIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on achievements groups API go here
* Get list of achievement group info corresponding to the given id(s)
*
* @param ids list of achievement group id(s)
* @return list of achievement group info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see AchievementGroup achievement group info
*/
public List getAchievementGroupInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getAchievementGroupInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Back Story
/**
* For more info on back story answer API go here
* Get list of all back story answer id(s)
*
* @return list of back story answer id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see BackStoryAnswer back story answer info
*/
public List getAllBackStoryAnswerID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllBackStoryAnswerIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on back story answer API go here
* Get list of back story answer info corresponding to the given id(s)
*
* @param ids list of back story answer id(s)
* @return list of back story answer info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see BackStoryAnswer back story answer info
*/
public List getBackStoryAnswerInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getBackStoryAnswerInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on back story questions API go here
* Get list of all available back story question id(s)
*
* @return list of back story question id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see BackStoryQuestion back story question info
*/
public List getAllBackStoryQuestionID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllBackStoryQuestionIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on back story questions API go here
* Get list of back story question info corresponding to the given id(s)
*
* @param ids list of back story question id(s)
* @return list of back story question info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see BackStoryQuestion back story question info
*/
public List getBackStoryQuestionInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getBackStoryQuestionInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Game Build
/**
* For more info on build API go here
* get current game bild
*
* @return current game build
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GameBuild game build info
*/
public GameBuild getCurrentGameBuild() throws GuildWars2Exception {
try {
Response response = gw2API.getCurrentGameBuild().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Cats
/**
* For more info on cats API go here
* Get list of all available cat id(s)
*
* @return list of cat id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color cat info
*/
public List getAllCatID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllCatIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on cats API go here
* Get cat info for the given cat id(s)
*
* @param ids array of cat id
* @return list of cat info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Cat cat info
*/
public List getCatInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getCatInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Characters
/**
* For more info on Character API go here
*
* @param API API key
* @return list of character name
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getAllCharacterName(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getAllCharacterName(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on character overview API go here
*
* @param API API key
* @param name name of character
* @return character detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Character character info
*/
public Character getCharacter(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacter(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on character back story API go here
*
* @param API API key
* @param name name of character
* @return list of {@link BackStoryAnswer#id}
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterBackStory back store answer info
*/
public CharacterBackStory getCharacterBackStory(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterBackStory(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Core API go here
*
* @param API API key
* @param name name of character
* @return character detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterCore basic character info
*/
public CharacterCore getCharacterInformation(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterCore(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on character crafting API go here
*
* @param API API key
* @param name name of character
* @return list of character crafting info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterCraftingLevel character crafting info
*/
public CharacterCraftingLevel getCharacterCrafting(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterCrafting(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on character equipment API go here
*
* @param API API key
* @param name name of character
* @return list of equipment
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterEquipment equipment info
*/
public CharacterEquipment getCharacterEquipment(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterEquipment(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on character hero points API go here
*
* @param API API key
* @param name name of character
* @return list of {@link BackStoryAnswer#id}
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see BackStoryAnswer hero points info
*/
public List getCharacterHeroPoints(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response> response = gw2API.getCharacterHeroPoints(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Inventory API go here
*
* @param API API key
* @param name name of character
* @return character inventory detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterInventory character inventory info
*/
public CharacterInventory getCharacterInventory(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterInventory(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Recipes API go here
*
* @param API API key
* @param name name of character
* @return list of {@link Recipe#id}
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Recipe recipe info
*/
public CharacterRecipes getCharacterUnlockedRecipes(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterUnlockedRecipes(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character SAB API go here
*
* @param API API key
* @param name name of character
* @return character SAB detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterSAB character SAB info
*/
public CharacterSAB getCharacterSAB(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterSAB(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Skills API go here
*
* @param API API key
* @param name name of character
* @return character inventory detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterSkills character skills info
*/
public CharacterSkills getCharacterSkills(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterSkills(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Specialization API go here
*
* @param API API key
* @param name name of character
* @return character specialization detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterSpecialization character specialization info
*/
public CharacterSpecialization getCharacterSpecialization(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterSpecialization(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Character Training API go here
*
* @param API API key
* @param name name of character
* @return list of character training detail
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see CharacterTraining character training info
*/
public CharacterTraining getCharacterTraining(String API, String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API), new ParamChecker(ParamType.CHAR, name));
try {
Response response = gw2API.getCharacterTraining(name, API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Colors
/**
* For more info on Color API go here
* Get list of all available color id(s)
*
* @return list of color id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color color info
*/
public List getAllColorID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllColorIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Color API go here
* Get color info for the given color id(s)
*
* @param ids array of color id
* @return list of color info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Color color info
*/
public List getColorInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getColorInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//TP
/**
* For more info on delivery API go here
* TODO Still need to test this; Use with caution
*
* @param API API key
* @return list of current items and coins available for pickup on this account
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Delivery delivery info
*/
public List getTPDeliveryInfo(String API) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getTPDeliveryInfo(API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on exchange API go here
*
* @return list of accepted resources for the gem exchange
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Exchange Exchange info
*/
public List getAllExchangeCurrency() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllExchangeCurrency().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on exchange coins API go here
*
* @param currency exchange currency type
* @param quantity The amount to exchange
* @return the current coins to gems exchange rate
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Exchange Exchange info
*/
public Exchange getExchangeInfo(Exchange.Type currency, long quantity) throws GuildWars2Exception {
isValueValid(quantity);
try {
Response response = gw2API.getExchangeInfo(currency.name(), Long.toString(quantity)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on listings API go here
*
* @return list of item ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Listing listing info
*/
public List getAllTPListingID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllTPListingIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on listings API go here
* Get listing info for the given item id(s)
*
* @param ids list of item id
* @return list of listings for given id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Listing listing info
*/
public List getTPListingInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getTPListingInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Listing Price API go here
* Get all id of items that is on the TP
*
* @return list of item ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getAllPriceID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllTPPriceIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Listing Price API go here
* Get price info for the given item id(s)
*
* @param ids list of item id
* @return list of listing price for given id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Prices listing price info
*/
public List getPriceInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getTPPriceInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Transaction API go here
* Get transaction info linked to given API key
*
* @param API API key
* @param time current | History
* @param type buy | sell
* @return list of transaction base on the selection, if there is nothing, return empty list
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Transaction transaction info
*/
public List getTPTransaction(String API, Transaction.Time time, Transaction.Type type) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.API, API));
try {
Response> response = gw2API.getTPTransaction(processListingTime(time), processListingType(type), API).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Continents
/**
* For more info on continents API go here
* Get all continent ids
*
* @return list of continent ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Continent continent info
*/
public List getAllContinentID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent info for the given continent id(s)
*
* @param ids list of continent id
* @return list of continent info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Continent continent info
*/
public List getContinentInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get all continent floor ids
*
* @param continentID {@link Continent#id}
* @return list of continent floor ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentFloor continent floor info
*/
public List getAllContinentFloorID(int continentID) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentFloorIDs(Integer.toString(continentID)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent info for the given continent floor id(s)
*
* @param continentID {@link Continent#id}
* @param ids list of continent floor id
* @return list of continent floor info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentFloor continent floor info
*/
public List getContinentFloorInfo(int continentID, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentFloorInfo(Integer.toString(continentID), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get all continent region ids
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @return list of continent region ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentRegion continent region info
*/
public List getAllContinentRegionID(int continentID, int floorID) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentRegionIDs(Integer.toString(continentID),
Integer.toString(floorID)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent region info for the given continent region id(s)
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param ids list of continent region id
* @return list of continent region info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentRegion continent region info
*/
public List getContinentRegionInfo(int continentID, int floorID, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentRegionInfo(Integer.toString(continentID),
Integer.toString(floorID), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get all continent map ids
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @return list of continent map ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap continent map info
*/
public List getAllContinentMapID(int continentID, int floorID, int regionID) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentMapIDs(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent map info for the given continent map id(s)
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param ids list of continent map id
* @return list of continent map info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap continent map info
*/
public List getContinentMapInfo(int continentID, int floorID, int regionID, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentMapInfo(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get all continent map sector ids
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param mapID {@link ContinentMap#id}
* @return list of continent map sector ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap.Sector continent map sector info
*/
public List getAllContinentSectorID(int continentID, int floorID, int regionID, int mapID) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentSectorIDs(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), Integer.toString(mapID)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent map sector info for the given continent map sector id(s)
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param mapID {@link ContinentMap#id}
* @param ids list of continent map sector id
* @return list of continent map sector info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap.Sector continent map sector info
*/
public List getContinentSectorInfo(int continentID, int floorID, int regionID, int mapID, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentSectorInfo(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), Integer.toString(mapID), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get all continent map PoI ids
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param mapID {@link ContinentMap#id}
* @return list of continent map PoI ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap.Sector continent map PoI info
*/
public List getAllContinentPOIID(int continentID, int floorID, int regionID, int mapID) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentPOIIDs(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), Integer.toString(mapID)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent map PoI info for the given continent map PoI id(s)
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param mapID {@link ContinentMap#id}
* @param ids list of continent map PoI id
* @return list of continent map PoI info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap.PoI continent map PoI info
*/
public List getContinentPOIInfo(int continentID, int floorID, int regionID, int mapID, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentPOIInfo(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), Integer.toString(mapID), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get all continent map task ids
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param mapID {@link ContinentMap#id}
* @return list of continent map task ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap.Task continent map task info
*/
public List getAllContinentTaskID(int continentID, int floorID, int regionID, int mapID) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllContinentTaskIDs(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), Integer.toString(mapID)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on continents API go here
* Get continent map task info for the given continent map task id(s)
*
* @param continentID {@link Continent#id}
* @param floorID {@link ContinentFloor#id}
* @param regionID {@link ContinentRegion#id}
* @param mapID {@link ContinentMap#id}
* @param ids list of continent map task id
* @return list of continent map task info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ContinentMap.Task continent map task info
*/
public List getContinentTaskInfo(int continentID, int floorID, int regionID, int mapID, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getContinentTaskInfo(Integer.toString(continentID),
Integer.toString(floorID), Integer.toString(regionID), Integer.toString(mapID), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Currencies
/**
* For more info on Currency API go here
* Get all currency ids
*
* @return list of currency ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Currency currency info
*/
public List getAllCurrencyID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllCurrencies().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Currency API go here
* Get currency info for the given currency id(s)
*
* @param ids list of currency id
* @return list of currency info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Currency currency info
*/
public List getCurrencyInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getCurrencyInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Dungeons
/**
* For more info on Dungeons API go here
* Get all dungeon name(s)
*
* @return list of dungeon name(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Dungeon dungeon info
*/
public List getAllDungeonName() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllDungeonName().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Dungeons API go here
* Get dungeon info for the given dungeon id(s)
*
* @param ids list of dungeon id
* @return list of dungeon info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Dungeon dungeon info
*/
public List getDungeonInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getDungeonInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Emblem
/**
* For more info on emblem API go here
*
* @return list containing two strings, foregrounds and backgrounds
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Emblem Emblem info
*/
public List getAllEmblemType() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllEmblemType().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on emblem API go here
*
* @param type foregrounds/backgrounds
* @return list of emblem id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Emblem Emblem info
*/
public List getAllEmblemIDs(Emblem.Type type) throws GuildWars2Exception {
try {
Response> response = gw2API.getAllEmblemIDs(type.name()).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Finishers API go here
* Get finisher info for the given finisher id(s)
*
* @param type foregrounds/backgrounds
* @param ids list of finisher id
* @return list of finisher info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Finisher finisher info
*/
public List getAllEmblemInfo(Emblem.Type type, int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
if (ids.length > 200)
throw new GuildWars2Exception(ErrorCode.ID, "id list too long; this endpoint is limited to 200 ids at once");
try {
Response> response = gw2API.getAllEmblemInfo(type.name(), processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Files
/**
* For more info on files API go here
*
* @return list of file id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Asset file info
*/
public List getAllFileID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllFileIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on files API go here
* Get file info for the given file id(s)
*
* @param ids list of file id
* @return Map of file id and file icon
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Asset file info
*/
public List getAllFileInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getAllFileInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Finishers
/**
* For more info on Finishers API go here
* Get all finisher id(s)
*
* @return list of finisher id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Finisher finisher info
*/
public List getAllFinisherID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllFinisherIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Finishers API go here
* Get finisher info for the given finisher id(s)
*
* @param ids list of finisher id
* @return list of finisher info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Finisher finisher info
*/
public List getFinisherInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getFinisherInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Gliders
/**
* For more info on gliders API go here
* Get all glider id(s)
*
* @return list of glider id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Glider glider info
*/
public List getAllGliderID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllGliderIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on gliders API go here
* Get glider info for the given glider id(s)
*
* @param ids list of glider id
* @return list of glider info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Glider glider info
*/
public List getGliderInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getGliderInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild
/**
* For more info on guild API go here
* Get guild info for the given guild id
*
* @param id {@link Guild#id}
* @return list of guild info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Guild guild info
*/
public Guild getGeneralGuildInfo(String id) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id));
try {
Response response = gw2API.getGeneralGuildInfo(id).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on guild API go here
* Get guild info for the given guild id
* Note: if the given account is not a member, sometime endpoint will return general guild info instead of detailed info
*
* @param id {@link Guild#id}
* @param api Guild Wars 2 API key
* @return list of guild info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Guild guild info
*/
public Guild getDetailedGuildInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response response = gw2API.getDetailedGuildInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Log
/**
* For more info on guild log API go here
* Get guild log info for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of guild log info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildLog guild log info
*/
public List getGuildLogInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildLogInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on guild log API go here
* filter out all log entries not newer than since
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @param since log id used to filter log entries
* @return list of guild log info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildLog guild log info
*/
public List getFilteredGuildLogInfo(String id, String api, int since) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getFilteredGuildLogInfo(id, api, Integer.toString(since)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Members
/**
* For more info on guild member API go here
* Get guild member info for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of guild member info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildMember guild member info
*/
public List getGuildMembersInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildMemberInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Ranks
/**
* For more info on guild rank API go here
* Get guild rank info for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of guild rank info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildRank guild rank info
*/
public List getGuildRankInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildRankInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Stash
/**
* For more info on guild stash API go here
* Get guild stash info for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of guild stash info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildStash guild stash info
*/
public List getGuildStashInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildStashInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Teams
/**
* For more info on guild teams API go here
* Get guild team info for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of guild team info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildTeam guild team info
*/
public List getGuildTeamsInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildTeamsInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Treasury
/**
* For more info on guild treasury API go here
* Get guild treasury info for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of guild treasury info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildTreasury guild treasury info
*/
public List getGuildTreasuryInfo(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildTreasuryInfo(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Unlocked Upgrades
/**
* For more info on guild unlocked upgrades API go here
* Get guild unlocked upgrades ids for the given guild id
*
* @param id {@link Guild#id}
* @param api Guild leader's Guild Wars 2 API key
* @return list of unlocked upgrades ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List getGuildUnlockedUpgradesID(String id, String api) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, id), new ParamChecker(ParamType.API, api));
try {
Response> response = gw2API.getGuildUpgradeIDs(id, api).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Permissions
/**
* For more info on guild permissions API go here
* Get all guild permission id(s)
*
* @return list of guild permission id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildPermission guild permission info
*/
public List getAllGuildPermissionID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllGuildPermissionIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on guild permissions API go here
* Get guild permission info for the given guild permission id(s)
*
* @param ids list of guild permission id
* @return list of guild permission info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildPermission guild permission info
*/
public List getGuildPermissionInfo(String[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getGuildPermissionInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Search
/**
* For more info on guild Search API go here
*
* @param name guild name
* @return list of guild id(s) of guilds that have matching name
* @throws GuildWars2Exception see {@link ErrorCode} for detail
*/
public List searchGuildID(String name) throws GuildWars2Exception {
isParamValid(new ParamChecker(ParamType.GUILD, name));
try {
Response> response = gw2API.searchGuildID(name).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Guild Upgrades
/**
* For more info on guild upgrades API go here
* Get all guild upgrade id(s)
*
* @return list of guild upgrade id(s)
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildUpgrade guild upgrade info
*/
public List getAllGuildUpgradeID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllGuildUpgradeIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on guild upgrades API go here
* Get guild upgrade info for the given guild upgrade id(s)
*
* @param ids list of guild upgrade id
* @return list of guild upgrade info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see GuildUpgrade guild upgrade info
*/
public List getGuildUpgradeInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getGuildUpgradeInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Items
/**
* For more info on Item API go here
* Get all available item ids
*
* @return list of item ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Item item info
*/
public List getAllItemID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllItemIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Item API go here
* Get item info for the given item id(s)
*
* @param ids list of item id
* @return list of item info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Item item info
*/
public List- getItemInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response
> response = gw2API.getItemInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Item Stats
/**
* For more info on Itemstat API go here
* Get all available itemstat ids
*
* @return list of itemstat ids
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ItemStats itemstat info
*/
public List getAllItemStatID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllItemStatIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on Itemstat API go here
* Get itemstat info for the given itemstat id(s)
*
* @param ids list of itemstat id
* @return list of itemstat info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see ItemStats itemstat info
*/
public List getItemStatInfo(int[] ids) throws GuildWars2Exception {
isParamValid(new ParamChecker(ids));
try {
Response> response = gw2API.getItemStatInfo(processIds(ids)).execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
//Legends
/**
* For more info on legends API go here
* Get all legend id(s)
*
* @return list of legend info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Legend legend info
*/
public List getAllLegendID() throws GuildWars2Exception {
try {
Response> response = gw2API.getAllLegendIDs().execute();
if (!response.isSuccessful()) throwError(response.code(), response.errorBody());
return response.body();
} catch (IOException e) {
throw new GuildWars2Exception(ErrorCode.Network, "Network Error: " + e.getMessage());
}
}
/**
* For more info on legends API go here
* Get legend info for the given legend id(s)
*
* @param ids list of legend id
* @return list of legend info
* @throws GuildWars2Exception see {@link ErrorCode} for detail
* @see Legend legend info
*/
public List