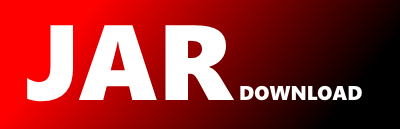
ml.comet.experiment.artifact.DownloadedArtifact Maven / Gradle / Ivy
package ml.comet.experiment.artifact;
import java.util.Map;
import java.util.Set;
/**
* Defines the public contract of the Comet Artifact which was downloaded.
*
* You can use this artifact object to update some properties of the artifact as well
* as to add new assets to it. After that, the artifact can be uploaded to the Comet
* using {@link ml.comet.experiment.OnlineExperiment#logArtifact(Artifact)} method.
*/
public interface DownloadedArtifact extends Artifact {
/**
* Returns unique identifier of the artifact.
*
* @return the unique identifier of the artifact.
*/
String getArtifactId();
/**
* Returns name of the artifact.
*
* @return the name of the artifact.
*/
String getName();
/**
* Returns the fully qualified name of the artifact in form 'workspace/name:version'.
*
* @return the fully qualified name of the artifact.
*/
String getFullName();
/**
* Returns type of the artifact.
*
* @return the type of the artifact.
*/
String getArtifactType();
/**
* Returns workspace name where artifact was logged.
*
* @return the workspace name where artifact was logged.
*/
String getWorkspace();
/**
* Returns the version of this artifact represented in semantic version format.
*
* @return the version of this artifact represented in semantic version format.
*/
String getVersion();
/**
* Sets new version for this artifact. If provided version is lower or equal than current version
* it would be applied but {@code false} will be returned. The Comet may accept version lower than current if it
* was not logged before. Thus, in general, it is safer to always log the greater version or check value returned
* but this method.
*
*
The version string should follow the semantic versioning rules and be in the form:
* {@code 1.2.3-beta.4+sha899d8g79f87}.
*
*
See {@link ArtifactBuilder#withVersion(String)} for details about version format.
*
* @param version the new version of the artifact.
* @return {@code true} if new version is valid, i.e., greater than current. The returned value can be used for
* quick check of the new version. If {@code true} returned then new version will be accepted by Comet backend.
* Otherwise, it may depend on backend already having provided artifact version logged.
*/
boolean setVersion(String version);
/**
* Allows bumping artifact version to the next major version.
*
*
See {@link ArtifactBuilder#withVersion(String)} for details about version format.
*
* @return the new artifact version.
*/
String incrementMajorVersion();
/**
* Allows bumping artifact version to the next minor version.
*
*
See {@link ArtifactBuilder#withVersion(String)} for details about version format.
*
* @return the new artifact version.
*/
String incrementMinorVersion();
/**
* Allows bumping artifact version to the next patch version.
*
*
See {@link ArtifactBuilder#withVersion(String)} for details about version format.
*
* @return the new artifact version.
*/
String incrementPatchVersion();
/**
* Returns set of TAGs associated with current version of the artifact.
*
* @return the set of TAGs associated with current version of the artifact.
*/
Set getVersionTags();
/**
* Sets the TAGs for the new version of the artifact. The current version TAGs will be replaced by
* provided ones.
*
* @param tags the set of version TAGs to replace existing.
*/
void setVersionTags(Set tags);
/**
* Returns set of aliases associated with the artifact.
*
* @return the set of aliases associated with artifact.
*/
Set getAliases();
/**
* Sets the new aliases to be associated with the artifact. The current aliases
* will be replaced by provided ones.
*
* @param aliases the set of aliases to be associated with artifact.
*/
void setAliases(Set aliases);
/**
* Returns metadata associated with artifact.
*
* @return the optional metadata associated with artifact.
*/
Map getMetadata();
}