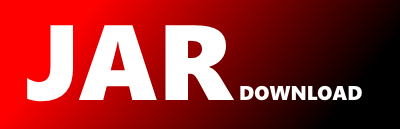
ml.comet.experiment.registrymodel.DownloadModelOptions Maven / Gradle / Ivy
package ml.comet.experiment.registrymodel;
import com.vdurmont.semver4j.Semver;
import static ml.comet.experiment.impl.resources.LogMessages.VERSION_AND_STAGE_SET_DOWNLOAD_MODEL;
import static ml.comet.experiment.impl.resources.LogMessages.getString;
/**
* Defines options to be used when downloading registry model.
*/
public class DownloadModelOptions {
private Semver semver;
private String stage;
private boolean expand;
private DownloadModelOptions() {
}
/**
* Returns the model version string or {@code null} if not set.
*
* @return the model version string or {@code null} if not set.
*/
public String getVersion() {
if (this.semver != null) {
return this.semver.getValue();
}
return null;
}
/**
* Returns {@code true} if downloaded model's ZIP should be expanded.
*
* @return the {@code true} if downloaded model's ZIP should be expanded.
*/
public boolean isExpand() {
return this.expand;
}
/**
* Returns stage to be associated with given model version.
*
* @return the stage to be associated with given model version.
*/
public String getStage() {
return this.stage;
}
/**
* Factory to create {@link DownloadModelOptionsBuilder} which can be used to create
* properly initialized instance of the {@link DownloadModelOptions}.
*
* @return the initialized {@link DownloadModelOptionsBuilder} instance.
*/
@SuppressWarnings("checkstyle:MethodName")
public static DownloadModelOptionsBuilder Op() {
return new DownloadModelOptionsBuilder();
}
/**
* The factory to create properly initialized instance of the {@link DownloadModelOptions}.
*/
public static final class DownloadModelOptionsBuilder {
final DownloadModelOptions options;
Boolean expand;
private DownloadModelOptionsBuilder() {
this.options = new DownloadModelOptions();
// set default value
this.expand = true;
}
/**
* Creates options with specific model version.
*
* @param version the version of the model in the registry.
* @return the instance of the {@link DownloadModelOptionsBuilder}.
*/
public DownloadModelOptionsBuilder withVersion(String version) {
this.options.semver = new Semver(version);
return this;
}
/**
* Creates options with TAG associated with particular model's version.
*
* @param stage the TAG associated with model version, such as: "production", "staging", etc.
* @return the instance of the {@link DownloadModelOptionsBuilder}.
*/
public DownloadModelOptionsBuilder withStage(String stage) {
this.options.stage = stage;
return this;
}
/**
* Creates options with flag to indicate whether downloaded ZIP with model files should be expanded.
*
* @param expand if {@code true} the downloaded ZIP with model files will be unzipped.
* @return the instance of the {@link DownloadModelOptionsBuilder}.
*/
public DownloadModelOptionsBuilder withExpand(boolean expand) {
this.expand = expand;
return this;
}
/**
* Creates properly initialized instance of the {@link DownloadModelOptions}.
*
* @return the properly initialized instance of the {@link DownloadModelOptions}.
* @throws IllegalArgumentException if simultaneously provided {@code version} and {@code stage} of the model.
*/
public DownloadModelOptions build() throws IllegalArgumentException {
if (this.options.stage != null && this.options.semver != null) {
throw new IllegalArgumentException(getString(VERSION_AND_STAGE_SET_DOWNLOAD_MODEL));
}
this.options.expand = this.expand;
return this.options;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy