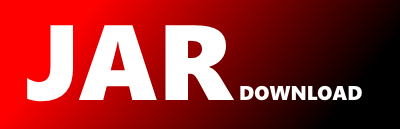
moe.maple.api.script.util.With Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of script-api Show documentation
Show all versions of script-api Show documentation
A java MapleStory(🍁) scripting api.
The newest version!
/*
* Copyright (C) 2019, y785, http://github.com/y785
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package moe.maple.api.script.util;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collection;
import java.util.function.Consumer;
public class With {
private static final Logger log = LoggerFactory.getLogger( With.class );
public static void silence(Consumers.Proxied proxy, Consumer exceptionConsumer) {
try {
proxy.work();
} catch (Exception e) {
exceptionConsumer.accept(e);
}
}
public static void silence(Consumers.Proxied proxy) {
silence(proxy, (e) -> {
log.debug("Trapped exception.", e);
});
}
public static void silentConsume(T object, Consumers.ContinueException consumer, Consumer exceptionConsumer) {
try {
consumer.accept(object);
} catch (Exception e) {
exceptionConsumer.accept(e);
}
}
public static void silentConsume(T object, Consumers.ContinueException consumer) {
silentConsume(object, consumer, (e) -> {
log.debug("Trapped exception.", e);
});
}
public static void continueException(Collection collection, Consumers.ContinueException consumer) {
for (T object : collection) {
silentConsume(object, consumer);
}
}
public static void continueException(T[] collection, Consumers.ContinueException consumer) {
for (T object : collection) {
silentConsume(object, consumer);
}
}
public static void index(Collection collection, Consumers.IndexedConsumer consumer) {
int index = 0;
for (T object : collection) {
consumer.accept(object, index++);
}
}
public static void index(T[] collection, Consumers.IndexedConsumer consumer) {
for (int i = 0; i < collection.length; i++) {
consumer.accept(collection[i], i);
}
}
public static void indexAndCount(Collection collection, Consumers.IndexedSizedConsumer consumer) {
var index = 0;
var length = collection.size();
for (T object : collection) {
consumer.accept(object, index++, length);
}
}
public static void indexAndCount(T[] collection, Consumers.IndexedSizedConsumer consumer) {
var length = collection.length;
for (int i = 0; i < collection.length; i++) {
consumer.accept(collection[i], i, length);
}
}
public static class Consumers {
@FunctionalInterface
public interface Proxied {
void work();
}
@FunctionalInterface
public interface IndexedConsumer {
void accept(T t, int index);
}
@FunctionalInterface
public interface IndexedSizedConsumer {
void accept(T t, int index, int length);
}
@FunctionalInterface
public interface ContinueException {
void accept(T t) throws Exception;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy