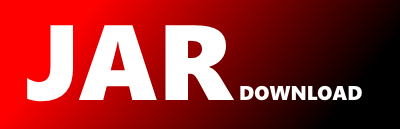
money.rave.common.backend.entity.user.User.kt Maven / Gradle / Ivy
package money.rave.common.backend.entity.user
import money.rave.common.backend.date.utc
import money.rave.common.backend.entity.wallet.Wallet
import money.rave.common.backend.entity.wallet.WalletOwner
import java.time.ZonedDateTime
import javax.persistence.*
@Entity
@Table(name = "user")
class User(
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
val id: Int? = null,
@Column(name = "email", nullable = false, length = 256, unique = true)
val email: String? = null, // unique
@Column(name = "password", nullable = false, columnDefinition = "TEXT")
val password: String? = null,
wallets: List = emptyList(),
@Column(name = "created_at", nullable = false)
val createdAt: ZonedDateTime = utc(),
@Column(name = "updated_at", nullable = false)
val updatedAt: ZonedDateTime = utc(),
) : WalletOwner(wallets) {
override fun equals(other: Any?): Boolean {
if (other !is User) return false
if (!super.equals(other)) return false
if (id != other.id) return false
if (email != other.email) return false
if (password != other.password) return false
if (createdAt != other.createdAt) return false
if (updatedAt != other.updatedAt) return false
return true
}
override fun hashCode(): Int {
var hashCode = super.hashCode()
hashCode = 31 * hashCode + id.hashCode()
hashCode = 31 * hashCode + email.hashCode()
hashCode = 31 * hashCode + password.hashCode()
hashCode = 31 * hashCode + createdAt.hashCode()
hashCode = 31 * hashCode + updatedAt.hashCode()
return hashCode
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy