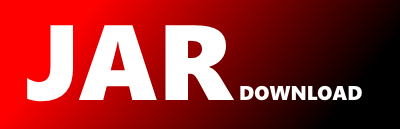
mx.bigdata.sat.cfdi.schema.TUbicacion Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-833
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.03.13 at 09:36:11 AM MST
//
package mx.bigdata.sat.cfdi.schema;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlType;
/**
* Tipo definido para expresar domicilios o direcciones
*
* Java class for t_Ubicacion complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="t_Ubicacion">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="calle">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="noExterior">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="noInterior">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="colonia">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="localidad">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="referencia">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="municipio">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="estado">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="pais" use="required">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="codigoPostal">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <whiteSpace value="collapse"/>
* </restriction>
* </simpleType>
* </attribute>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "t_Ubicacion")
public class TUbicacion
implements Serializable
{
private final static long serialVersionUID = -6026937020915831338L;
@XmlAttribute
protected String calle;
@XmlAttribute
protected String noExterior;
@XmlAttribute
protected String noInterior;
@XmlAttribute
protected String colonia;
@XmlAttribute
protected String localidad;
@XmlAttribute
protected String referencia;
@XmlAttribute
protected String municipio;
@XmlAttribute
protected String estado;
@XmlAttribute(required = true)
protected String pais;
@XmlAttribute
protected String codigoPostal;
/**
* Gets the value of the calle property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCalle() {
return calle;
}
/**
* Sets the value of the calle property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCalle(String value) {
this.calle = value;
}
/**
* Gets the value of the noExterior property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNoExterior() {
return noExterior;
}
/**
* Sets the value of the noExterior property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNoExterior(String value) {
this.noExterior = value;
}
/**
* Gets the value of the noInterior property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNoInterior() {
return noInterior;
}
/**
* Sets the value of the noInterior property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNoInterior(String value) {
this.noInterior = value;
}
/**
* Gets the value of the colonia property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getColonia() {
return colonia;
}
/**
* Sets the value of the colonia property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setColonia(String value) {
this.colonia = value;
}
/**
* Gets the value of the localidad property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLocalidad() {
return localidad;
}
/**
* Sets the value of the localidad property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLocalidad(String value) {
this.localidad = value;
}
/**
* Gets the value of the referencia property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReferencia() {
return referencia;
}
/**
* Sets the value of the referencia property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReferencia(String value) {
this.referencia = value;
}
/**
* Gets the value of the municipio property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMunicipio() {
return municipio;
}
/**
* Sets the value of the municipio property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMunicipio(String value) {
this.municipio = value;
}
/**
* Gets the value of the estado property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEstado() {
return estado;
}
/**
* Sets the value of the estado property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEstado(String value) {
this.estado = value;
}
/**
* Gets the value of the pais property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPais() {
return pais;
}
/**
* Sets the value of the pais property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPais(String value) {
this.pais = value;
}
/**
* Gets the value of the codigoPostal property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodigoPostal() {
return codigoPostal;
}
/**
* Sets the value of the codigoPostal property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodigoPostal(String value) {
this.codigoPostal = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy