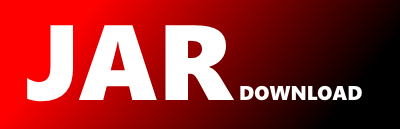
name.didier.david.test4j.assertions.jaxrs.ResponseAssert Maven / Gradle / Ivy
package name.didier.david.test4j.assertions.jaxrs;
import static javax.ws.rs.core.Response.Status.ACCEPTED;
import static javax.ws.rs.core.Response.Status.BAD_GATEWAY;
import static javax.ws.rs.core.Response.Status.BAD_REQUEST;
import static javax.ws.rs.core.Response.Status.CONFLICT;
import static javax.ws.rs.core.Response.Status.CREATED;
import static javax.ws.rs.core.Response.Status.EXPECTATION_FAILED;
import static javax.ws.rs.core.Response.Status.FORBIDDEN;
import static javax.ws.rs.core.Response.Status.FOUND;
import static javax.ws.rs.core.Response.Status.GATEWAY_TIMEOUT;
import static javax.ws.rs.core.Response.Status.GONE;
import static javax.ws.rs.core.Response.Status.HTTP_VERSION_NOT_SUPPORTED;
import static javax.ws.rs.core.Response.Status.INTERNAL_SERVER_ERROR;
import static javax.ws.rs.core.Response.Status.LENGTH_REQUIRED;
import static javax.ws.rs.core.Response.Status.METHOD_NOT_ALLOWED;
import static javax.ws.rs.core.Response.Status.MOVED_PERMANENTLY;
import static javax.ws.rs.core.Response.Status.NOT_ACCEPTABLE;
import static javax.ws.rs.core.Response.Status.NOT_FOUND;
import static javax.ws.rs.core.Response.Status.NOT_IMPLEMENTED;
import static javax.ws.rs.core.Response.Status.NOT_MODIFIED;
import static javax.ws.rs.core.Response.Status.NO_CONTENT;
import static javax.ws.rs.core.Response.Status.OK;
import static javax.ws.rs.core.Response.Status.PARTIAL_CONTENT;
import static javax.ws.rs.core.Response.Status.PAYMENT_REQUIRED;
import static javax.ws.rs.core.Response.Status.PRECONDITION_FAILED;
import static javax.ws.rs.core.Response.Status.PROXY_AUTHENTICATION_REQUIRED;
import static javax.ws.rs.core.Response.Status.REQUESTED_RANGE_NOT_SATISFIABLE;
import static javax.ws.rs.core.Response.Status.REQUEST_ENTITY_TOO_LARGE;
import static javax.ws.rs.core.Response.Status.REQUEST_TIMEOUT;
import static javax.ws.rs.core.Response.Status.REQUEST_URI_TOO_LONG;
import static javax.ws.rs.core.Response.Status.RESET_CONTENT;
import static javax.ws.rs.core.Response.Status.SEE_OTHER;
import static javax.ws.rs.core.Response.Status.SERVICE_UNAVAILABLE;
import static javax.ws.rs.core.Response.Status.TEMPORARY_REDIRECT;
import static javax.ws.rs.core.Response.Status.UNAUTHORIZED;
import static javax.ws.rs.core.Response.Status.UNSUPPORTED_MEDIA_TYPE;
import static javax.ws.rs.core.Response.Status.USE_PROXY;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import org.assertj.core.api.AbstractAssert;
import org.assertj.core.internal.Objects;
import name.didier.david.test4j.utils.JsonUtils;
/**
* Assertions dealing with {@link Response}.
*
* @author ddidier
*/
public class ResponseAssert
extends AbstractAssert {
/**
* @param actual the actual {@link Response} to assert against.
*/
public ResponseAssert(Response actual) {
super(actual, ResponseAssert.class);
}
/**
* @param actual the actual {@link Response} to assert against.
* @return a new {@link ResponseAssert}.
*/
public static ResponseAssert assertThat(Response actual) {
return new ResponseAssert(actual);
}
// -----------------------------------------------------------------------------------------------------------------
/**
* Verifies that the actual JSON content is equals to the given one, both will be normalized.
*
* @param expected the given JSON content to compare the actual JSON content to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual JSON content is not equal to the given one.
*/
public ResponseAssert hasJsonContent(String expected) {
isNotNull();
String content = actual.readEntity(String.class);
String actualContent = JsonUtils.normalize(content);
String expectedContent = JsonUtils.normalize(expected);
Objects.instance().assertEqual(info, actualContent, expectedContent);
return this;
}
/**
* Verifies that the actual JSON content is equals to the given one, both will be normalized. See
* {@link JsonUtils#json(CharSequence...)}.
*
* @param expectedElements the given JSON content to compare the actual JSON content to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual JSON content is not equal to the given one.
*/
public ResponseAssert hasJsonContent(CharSequence... expectedElements) {
return hasJsonContent(JsonUtils.json(expectedElements));
}
// -----------------------------------------------------------------------------------------------------------------
/**
* Verifies that the actual status is OK (200).
*
* @return this assertion.
* @throws AssertionError if the actual status is not OK.
*/
public ResponseAssert hasStatusOk() {
return assertStatus(OK);
}
/**
* Verifies that the actual status is CREATED (201).
*
* @return this assertion.
* @throws AssertionError if the actual status is not CREATED.
*/
public ResponseAssert hasStatusCreated() {
return assertStatus(CREATED);
}
/**
* Verifies that the actual status is ACCEPTED (202).
*
* @return this assertion.
* @throws AssertionError if the actual status is not ACCEPTED.
*/
public ResponseAssert hasStatusAccepted() {
return assertStatus(ACCEPTED);
}
/**
* Verifies that the actual status is NO_CONTENT (204).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NO_CONTENT.
*/
public ResponseAssert hasStatusNoContent() {
return assertStatus(NO_CONTENT);
}
/**
* Verifies that the actual status is RESET_CONTENT (205).
*
* @return this assertion.
* @throws AssertionError if the actual status is not RESET_CONTENT.
*/
public ResponseAssert hasStatusResetContent() {
return assertStatus(RESET_CONTENT);
}
/**
* Verifies that the actual status is PARTIAL_CONTENT (206).
*
* @return this assertion.
* @throws AssertionError if the actual status is not PARTIAL_CONTENT.
*/
public ResponseAssert hasStatusPartialContent() {
return assertStatus(PARTIAL_CONTENT);
}
/**
* Verifies that the actual status is MOVED_PERMANENTLY (301).
*
* @return this assertion.
* @throws AssertionError if the actual status is not MOVED_PERMANENTLY.
*/
public ResponseAssert hasStatusMovedPermanently() {
return assertStatus(MOVED_PERMANENTLY);
}
/**
* Verifies that the actual status is FOUND (302).
*
* @return this assertion.
* @throws AssertionError if the actual status is not FOUND.
*/
public ResponseAssert hasStatusFound() {
return assertStatus(FOUND);
}
/**
* Verifies that the actual status is SEE_OTHER (303).
*
* @return this assertion.
* @throws AssertionError if the actual status is not SEE_OTHER.
*/
public ResponseAssert hasStatusSeeOther() {
return assertStatus(SEE_OTHER);
}
/**
* Verifies that the actual status is NOT_MODIFIED (304).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NOT_MODIFIED.
*/
public ResponseAssert hasStatusNotModified() {
return assertStatus(NOT_MODIFIED);
}
/**
* Verifies that the actual status is USE_PROXY (305).
*
* @return this assertion.
* @throws AssertionError if the actual status is not USE_PROXY.
*/
public ResponseAssert hasStatusUseProxy() {
return assertStatus(USE_PROXY);
}
/**
* Verifies that the actual status is TEMPORARY_REDIRECT (307).
*
* @return this assertion.
* @throws AssertionError if the actual status is not TEMPORARY_REDIRECT.
*/
public ResponseAssert hasStatusTemporaryRedirect() {
return assertStatus(TEMPORARY_REDIRECT);
}
/**
* Verifies that the actual status is BAD_REQUEST (400).
*
* @return this assertion.
* @throws AssertionError if the actual status is not BAD_REQUEST.
*/
public ResponseAssert hasStatusBadRequest() {
return assertStatus(BAD_REQUEST);
}
/**
* Verifies that the actual status is UNAUTHORIZED (401).
*
* @return this assertion.
* @throws AssertionError if the actual status is not UNAUTHORIZED.
*/
public ResponseAssert hasStatusUnauthorized() {
return assertStatus(UNAUTHORIZED);
}
/**
* Verifies that the actual status is PAYMENT_REQUIRED (402).
*
* @return this assertion.
* @throws AssertionError if the actual status is not PAYMENT_REQUIRED.
*/
public ResponseAssert hasStatusPaymentRequired() {
return assertStatus(PAYMENT_REQUIRED);
}
/**
* Verifies that the actual status is FORBIDDEN (403).
*
* @return this assertion.
* @throws AssertionError if the actual status is not FORBIDDEN.
*/
public ResponseAssert hasStatusForbidden() {
return assertStatus(FORBIDDEN);
}
/**
* Verifies that the actual status is NOT_FOUND (404).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NOT_FOUND.
*/
public ResponseAssert hasStatusNotFound() {
return assertStatus(NOT_FOUND);
}
/**
* Verifies that the actual status is METHOD_NOT_ALLOWED (405).
*
* @return this assertion.
* @throws AssertionError if the actual status is not METHOD_NOT_ALLOWED.
*/
public ResponseAssert hasStatusMethodNotAllowed() {
return assertStatus(METHOD_NOT_ALLOWED);
}
/**
* Verifies that the actual status is NOT_ACCEPTABLE (406).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NOT_ACCEPTABLE.
*/
public ResponseAssert hasStatusNotAcceptable() {
return assertStatus(NOT_ACCEPTABLE);
}
/**
* Verifies that the actual status is PROXY_AUTHENTICATION_REQUIRED (407).
*
* @return this assertion.
* @throws AssertionError if the actual status is not PROXY_AUTHENTICATION_REQUIRED.
*/
public ResponseAssert hasStatusProxyAuthenticationRequired() {
return assertStatus(PROXY_AUTHENTICATION_REQUIRED);
}
/**
* Verifies that the actual status is REQUEST_TIMEOUT (408).
*
* @return this assertion.
* @throws AssertionError if the actual status is not REQUEST_TIMEOUT.
*/
public ResponseAssert hasStatusRequestTimeout() {
return assertStatus(REQUEST_TIMEOUT);
}
/**
* Verifies that the actual status is CONFLICT (409).
*
* @return this assertion.
* @throws AssertionError if the actual status is not CONFLICT.
*/
public ResponseAssert hasStatusConflict() {
return assertStatus(CONFLICT);
}
/**
* Verifies that the actual status is GONE (410).
*
* @return this assertion.
* @throws AssertionError if the actual status is not GONE.
*/
public ResponseAssert hasStatusGone() {
return assertStatus(GONE);
}
/**
* Verifies that the actual status is LENGTH_REQUIRED (411).
*
* @return this assertion.
* @throws AssertionError if the actual status is not LENGTH_REQUIRED.
*/
public ResponseAssert hasStatusLengthRequired() {
return assertStatus(LENGTH_REQUIRED);
}
/**
* Verifies that the actual status is PRECONDITION_FAILED (412).
*
* @return this assertion.
* @throws AssertionError if the actual status is not PRECONDITION_FAILED.
*/
public ResponseAssert hasStatusPreconditionFailed() {
return assertStatus(PRECONDITION_FAILED);
}
/**
* Verifies that the actual status is REQUEST_ENTITY_TOO_LARGE (413).
*
* @return this assertion.
* @throws AssertionError if the actual status is not REQUEST_ENTITY_TOO_LARGE.
*/
public ResponseAssert hasStatusRequestEntityTooLarge() {
return assertStatus(REQUEST_ENTITY_TOO_LARGE);
}
/**
* Verifies that the actual status is REQUEST_URI_TOO_LONG (414).
*
* @return this assertion.
* @throws AssertionError if the actual status is not REQUEST_URI_TOO_LONG.
*/
public ResponseAssert hasStatusRequestUriTooLong() {
return assertStatus(REQUEST_URI_TOO_LONG);
}
/**
* Verifies that the actual status is UNSUPPORTED_MEDIA_TYPE (415).
*
* @return this assertion.
* @throws AssertionError if the actual status is not UNSUPPORTED_MEDIA_TYPE.
*/
public ResponseAssert hasStatusUnsupportedMediaType() {
return assertStatus(UNSUPPORTED_MEDIA_TYPE);
}
/**
* Verifies that the actual status is REQUESTED_RANGE_NOT_SATISFIABLE (416).
*
* @return this assertion.
* @throws AssertionError if the actual status is not REQUESTED_RANGE_NOT_SATISFIABLE.
*/
public ResponseAssert hasStatusRequestedRangeNotSatisfiable() {
return assertStatus(REQUESTED_RANGE_NOT_SATISFIABLE);
}
/**
* Verifies that the actual status is EXPECTATION_FAILED (417).
*
* @return this assertion.
* @throws AssertionError if the actual status is not EXPECTATION_FAILED.
*/
public ResponseAssert hasStatusExpectationFailed() {
return assertStatus(EXPECTATION_FAILED);
}
/**
* Verifies that the actual status is INTERNAL_SERVER_ERROR (500).
*
* @return this assertion.
* @throws AssertionError if the actual status is not INTERNAL_SERVER_ERROR.
*/
public ResponseAssert hasStatusInternalServerError() {
return assertStatus(INTERNAL_SERVER_ERROR);
}
/**
* Verifies that the actual status is NOT_IMPLEMENTED (501).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NOT_IMPLEMENTED.
*/
public ResponseAssert hasStatusNotImplemented() {
return assertStatus(NOT_IMPLEMENTED);
}
/**
* Verifies that the actual status is BAD_GATEWAY (502).
*
* @return this assertion.
* @throws AssertionError if the actual status is not BAD_GATEWAY.
*/
public ResponseAssert hasStatusBadGateway() {
return assertStatus(BAD_GATEWAY);
}
/**
* Verifies that the actual status is SERVICE_UNAVAILABLE (503).
*
* @return this assertion.
* @throws AssertionError if the actual status is not SERVICE_UNAVAILABLE.
*/
public ResponseAssert hasStatusServiceUnavailable() {
return assertStatus(SERVICE_UNAVAILABLE);
}
/**
* Verifies that the actual status is GATEWAY_TIMEOUT (504).
*
* @return this assertion.
* @throws AssertionError if the actual status is not GATEWAY_TIMEOUT.
*/
public ResponseAssert hasStatusGatewayTimeout() {
return assertStatus(GATEWAY_TIMEOUT);
}
/**
* Verifies that the actual status is HTTP_VERSION_NOT_SUPPORTED (505).
*
* @return this assertion.
* @throws AssertionError if the actual status is not HTTP_VERSION_NOT_SUPPORTED.
*/
public ResponseAssert hasStatusHttpVersionNotSupported() {
return assertStatus(HTTP_VERSION_NOT_SUPPORTED);
}
// -----------------------------------------------------------------------------------------------------------------
// CSOFF: MagicNumberCheck
/**
* Verifies that the actual status is UNPROCESSABLE_ENTITY (422).
*
* @return this assertion.
* @throws AssertionError if the actual status is not UNPROCESSABLE_ENTITY.
*/
public ResponseAssert hasStatusUnprocessableEntity() {
return assertStatus(422);
}
/**
* Verifies that the actual status is MISDIRECTED_REQUEST (421).
*
* @return this assertion.
* @throws AssertionError if the actual status is not MISDIRECTED_REQUEST.
*/
public ResponseAssert hasStatusMisdirectedRequest() {
return assertStatus(421);
}
/**
* Verifies that the actual status is IM_A_TEAPOT (418).
*
* @return this assertion.
* @throws AssertionError if the actual status is not IM_A_TEAPOT.
*/
public ResponseAssert hasStatusImATeapot() {
return assertStatus(418);
}
/**
* Verifies that the actual status is NETWORK_AUTHENTICATION_REQUIRED (511).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NETWORK_AUTHENTICATION_REQUIRED.
*/
public ResponseAssert hasStatusNetworkAuthenticationRequired() {
return assertStatus(511);
}
/**
* Verifies that the actual status is NOT_EXTENDED (510).
*
* @return this assertion.
* @throws AssertionError if the actual status is not NOT_EXTENDED.
*/
public ResponseAssert hasStatusNotExtended() {
return assertStatus(510);
}
/**
* Verifies that the actual status is LOOP_DETECTED (508).
*
* @return this assertion.
* @throws AssertionError if the actual status is not LOOP_DETECTED.
*/
public ResponseAssert hasStatusLoopDetected() {
return assertStatus(508);
}
/**
* Verifies that the actual status is I_M_USED (226).
*
* @return this assertion.
* @throws AssertionError if the actual status is not I_M_USED.
*/
public ResponseAssert hasStatusIMUsed() {
return assertStatus(226);
}
/**
* Verifies that the actual status is INSUFFICIENT_STORAGE (507).
*
* @return this assertion.
* @throws AssertionError if the actual status is not INSUFFICIENT_STORAGE.
*/
public ResponseAssert hasStatusInsufficientStorage() {
return assertStatus(507);
}
/**
* Verifies that the actual status is VARIANT_ALSO_NEGOTIATES (506).
*
* @return this assertion.
* @throws AssertionError if the actual status is not VARIANT_ALSO_NEGOTIATES.
*/
public ResponseAssert hasStatusVariantAlsoNegotiates() {
return assertStatus(506);
}
/**
* Verifies that the actual status is UNAVAILABLE_FOR_LEGAL_REASONS (451).
*
* @return this assertion.
* @throws AssertionError if the actual status is not UNAVAILABLE_FOR_LEGAL_REASONS.
*/
public ResponseAssert hasStatusUnavailableForLegalReasons() {
return assertStatus(451);
}
/**
* Verifies that the actual status is PERMANENT_REDIRECT (308).
*
* @return this assertion.
* @throws AssertionError if the actual status is not PERMANENT_REDIRECT.
*/
public ResponseAssert hasStatusPermanentRedirect() {
return assertStatus(308);
}
/**
* Verifies that the actual status is SWITCH_PROXY (306).
*
* @return this assertion.
* @throws AssertionError if the actual status is not SWITCH_PROXY.
*/
public ResponseAssert hasStatusSwitchProxy() {
return assertStatus(306);
}
/**
* Verifies that the actual status is ALREADY_REPORTED (208).
*
* @return this assertion.
* @throws AssertionError if the actual status is not ALREADY_REPORTED.
*/
public ResponseAssert hasStatusAlreadyReported() {
return assertStatus(208);
}
/**
* Verifies that the actual status is MULTI_STATUS (207).
*
* @return this assertion.
* @throws AssertionError if the actual status is not MULTI_STATUS.
*/
public ResponseAssert hasStatusMultiStatus() {
return assertStatus(207);
}
/**
* Verifies that the actual status is REQUEST_HEADER_FIELDS_TOO_LARGE (431).
*
* @return this assertion.
* @throws AssertionError if the actual status is not REQUEST_HEADER_FIELDS_TOO_LARGE.
*/
public ResponseAssert hasStatusRequestHeaderFieldsTooLarge() {
return assertStatus(431);
}
/**
* Verifies that the actual status is TOO_MANY_REQUESTS (429).
*
* @return this assertion.
* @throws AssertionError if the actual status is not TOO_MANY_REQUESTS.
*/
public ResponseAssert hasStatusTooManyRequests() {
return assertStatus(429);
}
/**
* Verifies that the actual status is PRECONDITION_REQUIRED (428).
*
* @return this assertion.
* @throws AssertionError if the actual status is not PRECONDITION_REQUIRED.
*/
public ResponseAssert hasStatusPreconditionRequired() {
return assertStatus(428);
}
/**
* Verifies that the actual status is UPGRADE_REQUIRED (426).
*
* @return this assertion.
* @throws AssertionError if the actual status is not UPGRADE_REQUIRED.
*/
public ResponseAssert hasStatusUpgradeRequired() {
return assertStatus(426);
}
/**
* Verifies that the actual status is FAILED_DEPENDENCY (424).
*
* @return this assertion.
* @throws AssertionError if the actual status is not FAILED_DEPENDENCY.
*/
public ResponseAssert hasStatusFailedDependency() {
return assertStatus(424);
}
/**
* Verifies that the actual status is LOCKED (423).
*
* @return this assertion.
* @throws AssertionError if the actual status is not LOCKED.
*/
public ResponseAssert hasStatusLocked() {
return assertStatus(423);
}
// CSON: MagicNumberCheck
// -----------------------------------------------------------------------------------------------------------------
/**
* Verifies that the actual status is equal to the given one.
*
* @param expectedStatus the status to check against.
* @return this assertion.
* @throws AssertionError if the actual status is not equal to the given one.
*/
private ResponseAssert assertStatus(int expectedStatus) {
isNotNull();
if (actual.getStatus() != expectedStatus) {
failWithMessage("Expected status code to be <%d> but was <%d>", expectedStatus, actual.getStatus());
}
return this;
}
/**
* Verifies that the actual status is equal to the given one.
*
* @param expectedStatus the status to check against.
* @return this assertion.
* @throws AssertionError if the actual status is not equal to the given one.
*/
private ResponseAssert assertStatus(Status expectedStatus) {
return assertStatus(expectedStatus.getStatusCode());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy