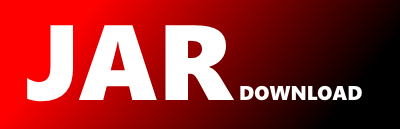
name.didier.david.test4j.dbunit.DatabaseSchemaExporter Maven / Gradle / Ivy
package name.didier.david.test4j.dbunit;
import static name.didier.david.check4j.ConciseCheckers.checkNotBlank;
import static name.didier.david.check4j.ConciseCheckers.checkNotNull;
import java.io.File;
import java.io.IOException;
import java.io.StringWriter;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import javax.sql.DataSource;
import org.dbunit.DatabaseUnitException;
import org.dbunit.database.DatabaseConnection;
import org.dbunit.database.DatabaseDataSourceConnection;
import org.dbunit.database.DatabaseSequenceFilter;
import org.dbunit.database.IDatabaseConnection;
import org.dbunit.dataset.DataSetException;
import org.dbunit.dataset.FilteredDataSet;
import org.dbunit.dataset.IDataSet;
import org.dbunit.dataset.filter.ITableFilter;
import org.dbunit.dataset.xml.FlatDtdDataSet;
import com.google.common.base.Charsets;
/**
* Export the schema of a database.
*
* @author ddidier
*/
public class DatabaseSchemaExporter {
/** The database connection. */
private final IDatabaseConnection connection;
/**
* @param connection the database connection.
*/
public DatabaseSchemaExporter(IDatabaseConnection connection) {
this.connection = checkNotNull(connection, "connection");
}
/**
* Create a {@link DatabaseSchemaExporter} with the given {@link Connection}.
*
* @param connection the database connection.
* @throws DatabaseUnitException if an error occurs.
*/
public DatabaseSchemaExporter(Connection connection)
throws DatabaseUnitException {
this(new DatabaseConnection(connection));
}
/**
* Create a {@link DatabaseSchemaExporter} with the given {@link DataSource}.
*
* @param dataSource the database connection.
* @throws SQLException if an error occurs.
*/
public DatabaseSchemaExporter(DataSource dataSource)
throws SQLException {
this(new DatabaseDataSourceConnection(dataSource));
}
/**
* Create a {@link DatabaseSchemaExporter} with the given database properties.
*
* @param url the connection URL.
* @param user the connection user name.
* @param password the connection password.
* @throws SQLException if a database access error occurs.
* @throws DatabaseUnitException if an error occurs.
*/
public DatabaseSchemaExporter(String url, String user, String password)
throws DatabaseUnitException, SQLException {
this(DriverManager.getConnection(checkNotBlank(url, "url"), user, password));
}
/**
* Create a {@link DatabaseSchemaExporter} with the given {@link Connection}.
*
* @param connection the database connection.
* @return a {@link DatabaseSchemaExporter} with the given {@link Connection}.
*/
public static DatabaseSchemaExporter from(Connection connection) {
try {
checkNotNull(connection, "connection");
return new DatabaseSchemaExporter(connection);
} catch (DatabaseUnitException e) {
// should not happen because there is no schema validation
throw new RuntimeException(e);
}
}
/**
* Create a {@link DatabaseSchemaExporter} with the given {@link DataSource}.
*
* @param dataSource the database connection.
* @return a {@link DatabaseSchemaExporter} with the given {@link DataSource}.
*/
public static DatabaseSchemaExporter from(DataSource dataSource) {
try {
checkNotNull(dataSource, "dataSource");
return new DatabaseSchemaExporter(dataSource);
} catch (SQLException e) {
// should not happen in the code...
throw new RuntimeException(e);
}
}
/**
* Create a {@link DatabaseSchemaExporter} with the given database properties.
*
* @param url the connection URL.
* @param user the connection user name.
* @param password the connection password.
* @return a {@link DatabaseSchemaExporter} with the given database properties.
* @throws SQLException if a database access error occurs.
*/
public static DatabaseSchemaExporter from(String url, String user, String password)
throws SQLException {
return from(DriverManager.getConnection(checkNotBlank(url, "url"), user, password));
}
/**
* Export the schema of a database as a DTD.
*
* @return the schema of a database as a DTD.
*/
public String exportDtd() {
try {
ITableFilter tableFilter = new DatabaseSequenceFilter(connection);
IDataSet dataSet = new FilteredDataSet(tableFilter, connection.createDataSet());
StringWriter buffer = new StringWriter();
FlatDtdDataSet.write(dataSet, buffer);
return buffer.toString();
} catch (DataSetException | IOException | SQLException e) {
throw new RuntimeException("Error while exporting schema", e);
}
}
/**
* Export the schema of a database to a file as a DTD.
*
* @param destinationFile the destination file.
*/
public void exportDtdTo(File destinationFile) {
try {
checkNotNull(destinationFile, "destinationFile");
Files.write(Paths.get(destinationFile.getAbsolutePath()), exportDtd().getBytes(Charsets.UTF_8));
} catch (IOException e) {
throw new RuntimeException("Error while exporting schema to " + destinationFile, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy