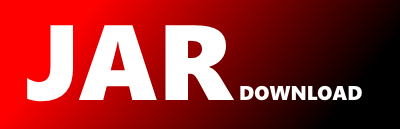
name.didier.david.test4j.junit.JUnitDataProviders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ndd-test4j Show documentation
Show all versions of ndd-test4j Show documentation
Test4J provides testing support.
The newest version!
package name.didier.david.test4j.junit;
import com.tngtech.java.junit.dataprovider.DataProvider;
import name.didier.david.test4j.unit.DataProviders;
/**
* Useful JUnit data providers for common testing patterns.
*
*
* Usage:
*
*
*
* import static name.didier.david.test4j.junit.JUnitDataProviders.BLANK_STRINGS;
*
* @Test
* @UseDataProvider(location = JUnitDataProviders.class, value = BLANK_STRINGS)
* public void test() { ... }
*
*
* @author ddidier
*/
public final class JUnitDataProviders {
/** Name of a data provider providing null strings. */
public static final String NULL_STRINGS = "nullStrings";
/** Name of a data provider providing empty strings. */
public static final String EMPTY_STRINGS = "emptyStrings";
/** Name of a data provider providing blank strings. */
public static final String BLANK_STRINGS = "blankStrings";
/** Name of a data provider providing empty but not null strings. */
public static final String EMPTY_BUT_NOT_NULL_STRINGS = "emptyButNotNullStrings";
/** Name of a data provider providing strictly negative numbers. */
public static final String STRICTLY_NEGATIVE_NUMBERS = "strictlyNegativeNumbers";
/** Name of a data provider providing negative numbers. */
public static final String NEGATIVE_NUMBERS = "negativeNumbers";
/** Name of a data provider providing strictly positive numbers. */
public static final String STRICTLY_POSITIVE_NUMBERS = "strictlyPositiveNumbers";
/** Name of a data provider providing positive numbers. */
public static final String POSITIVE_NUMBERS = "positiveNumbers";
/** Name of a data provider providing empty lists. */
public static final String EMPTY_LISTS = "emptyLists";
/** Name of a data provider providing empty sets. */
public static final String EMPTY_SETS = "emptySets";
/** Name of a data provider providing non existing files. */
public static final String NON_EXISTING_FILES = "nonExistingFiles";
/** The HMAC key. */
public static final String HMAC_KEY = "A very private key";
/** Name of a data provider providing HMAC using MD5 encoded in base 16. */
public static final String HMAC_MD5_BASE16 = "hmacMd5Base16";
/** Name of a data provider providing HMAC using MD5 encoded in base 64. */
public static final String HMAC_MD5_BASE64 = "hmacMd5Base64";
/** Name of a data provider providing HMAC using SHA 256 encoded in base 16. */
public static final String HMAC_SHA256_BASE16 = "hmacSha256Base16";
/** Name of a data provider providing HMAC using SHA 256 encoded in base 64. */
public static final String HMAC_SHA256_BASE64 = "hmacSha256Base64";
/** Utility class. */
private JUnitDataProviders() {
super();
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @return {@code null} strings.
*/
@DataProvider
public static Object[][] nullStrings() {
return DataProviders.nullStrings();
}
/**
* @return {@code null} and empty strings.
*/
@DataProvider
public static Object[][] emptyStrings() {
return DataProviders.emptyStrings();
}
/**
* @return {@code null}, empty and blank strings.
*/
@DataProvider
public static Object[][] blankStrings() {
return DataProviders.blankStrings();
}
/**
* @return empty and blank strings.
*/
@DataProvider
public static Object[][] emptyButNotNullStrings() {
return DataProviders.emptyButNotNullStrings();
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @return strictly negative numbers.
*/
@DataProvider
public static Object[][] strictlyNegativeNumbers() {
return DataProviders.strictlyNegativeNumbers();
}
/**
* @return negative or zero numbers.
*/
@DataProvider
public static Object[][] negativeNumbers() {
return DataProviders.negativeNumbers();
}
/**
* @return strictly positive numbers.
*/
@DataProvider
public static Object[][] strictlyPositiveNumbers() {
return DataProviders.strictlyPositiveNumbers();
}
/**
* @return positive or zero numbers.
*/
@DataProvider
public static Object[][] positiveNumbers() {
return DataProviders.positiveNumbers();
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @param the type of the elements in the list.
* @return {@code null} and empty {@link java.util.List}.
*/
@DataProvider
public static Object[][] emptyLists() {
return DataProviders.emptyLists();
}
/**
* @param the type of the elements in the set.
* @return {@code null} and empty {@link java.util.Set}.
*/
@DataProvider
public static Object[][] emptySets() {
return DataProviders.emptySets();
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @return non existing files: empty path, blank path, invalid path.
*/
@DataProvider
public static Object[][] nonExistingFiles() {
return DataProviders.nonExistingFiles();
}
// -----------------------------------------------------------------------------------------------------------------
// Digests and conversions done with:
// - http://www.freeformatter.com/hmac-generator.html
// - http://tomeko.net/online_tools/hex_to_base64.php?lang=en
/**
* @return a pair message/signature (HMAC using MD5 encoded in hexadecimal).
*/
@DataProvider
public static Object[][] hmacMd5Base16() {
return DataProviders.hmacMd5Base16();
}
/**
* @return a pair message/signature (HMAC using MD5 encoded in base 64).
*/
@DataProvider
public static Object[][] hmacMd5Base64() {
return DataProviders.hmacMd5Base64();
}
/**
* @return a pair message/signature (HMAC using SHA256 encoded in hexadecimal).
*/
@DataProvider
public static Object[][] hmacSha256Base16() {
return DataProviders.hmacSha256Base16();
}
/**
* @return a pair message/signature (HMAC using SHA256 encoded in base 64).
*/
@DataProvider
public static Object[][] hmacSha256Base64() {
return DataProviders.hmacSha256Base64();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy